Variable Manipulation: Computer Programming Languages Handling of Variables
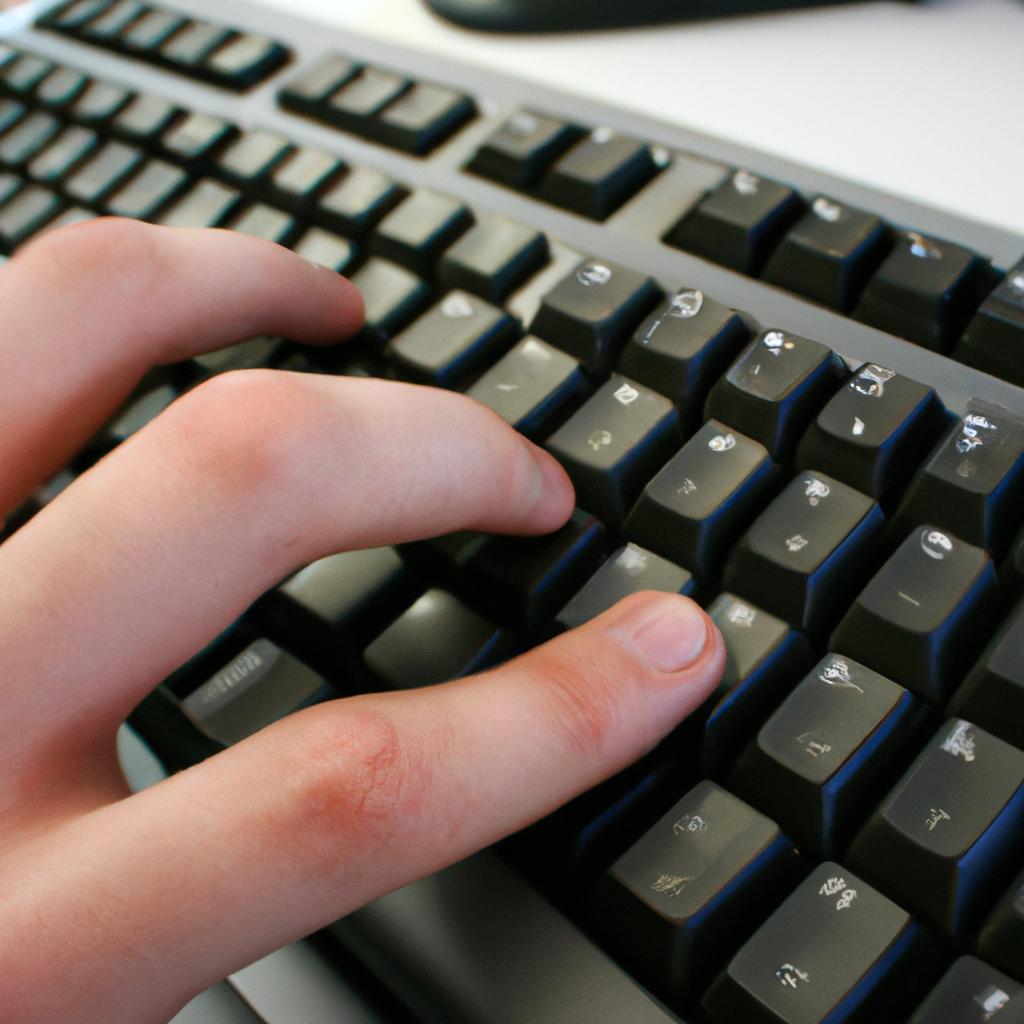
Variable manipulation is a fundamental aspect of computer programming languages, as it allows for the storage and modification of data within a program. The ability to handle variables effectively plays an essential role in the development of efficient and functional software. For instance, consider a hypothetical scenario where a programmer is designing a weather forecasting application. In order to provide accurate and up-to-date information, the program would need to manipulate variables such as temperature, humidity, wind speed, and precipitation levels. Understanding how different programming languages handle these variables is crucial for ensuring that the application operates smoothly and delivers reliable results.
Computer programming languages vary in their approaches to handling variables. Some languages use static typing, where variable types are defined at compile-time and cannot be changed during execution. On the other hand, dynamic typing allows for flexible variable assignments that can be modified throughout the program’s runtime. Additionally, some programming languages have built-in features for automatic memory management to handle variables efficiently without requiring explicit deallocation by the programmer. By exploring these diverse methods of variable manipulation across various programming languages, researchers can gain insights into best practices and identify potential challenges or limitations associated with each approach.
Understanding how different computer programming languages handle variables enables programmers to make informed decisions when selecting a language for specific projects and optimally utilize resources and tools available to them. For example, if a project requires high-performance computing or extensive mathematical calculations, a language like C++ or Fortran that allows for efficient memory management and low-level control over variables may be the best choice. On the other hand, if rapid development and ease of use are prioritized, languages like Python or JavaScript with their dynamic typing and extensive libraries can provide quicker results.
Furthermore, understanding variable manipulation in different programming languages helps programmers write clean and maintainable code. By knowing how variables are declared, assigned, and modified in a particular language, developers can follow best practices and avoid common pitfalls. This knowledge also facilitates collaboration within software development teams as members can easily understand and work with each other’s code.
In conclusion, variable manipulation is an essential aspect of computer programming languages that allows for the storage and modification of data. Understanding how different programming languages handle variables enables programmers to make informed decisions about language selection, optimize resource utilization, write clean code, and collaborate effectively within development teams.
Definition of Variable Manipulation
Variable manipulation is a fundamental concept in computer programming languages, allowing programmers to store and modify data during the execution of a program. It involves the creation, assignment, modification, and retrieval of values stored in memory locations known as variables. To illustrate this concept, let’s consider an example: imagine a programmer developing a weather application that displays current temperature readings for different cities around the world.
In order to implement such an application, the programmer would need to manipulate variables representing temperature data. This could involve creating variables to hold temperature values for each city, assigning initial values to these variables based on real-time data sources, updating them periodically with new measurements, and retrieving their values when needed for display purposes. By manipulating these variables effectively, the programmer can ensure accurate and up-to-date information is provided to users of the application.
To better understand the significance of variable manipulation in computer programming languages, we can explore its emotional impact on programmers:
- Efficiency: Proper variable manipulation techniques improve code efficiency by minimizing redundant operations and optimizing resource usage.
- Precision: Accurate manipulation of variables ensures reliable results and prevents errors or unexpected behaviors in programs.
- Flexibility: Variables allow programmers to adapt their code dynamically by storing changing values that influence program behavior.
- Readability: Well-manipulated variables enhance code readability through clear declaration and consistent naming conventions.
Additionally, we can visualize some key aspects related to variable manipulation using a table:
Key Aspects | Explanation |
---|---|
Creation | The process of declaring a new variable with a specific name and type. |
Assignment | Assigning an initial value to a variable at its creation or later stages. |
Modification | Altering the value stored within a variable after it has been assigned. |
Retrieval | Accessing the value stored in a variable for use in calculations or output. |
In conclusion, variable manipulation is a central concept in computer programming languages that enables programmers to effectively store, modify, and retrieve data during program execution. By employing proper techniques for creating, assigning, modifying, and retrieving variables, programmers can improve code efficiency, ensure accuracy and precision, adapt their programs dynamically, and enhance code readability. This lays the foundation for further exploration into common operations on variables.
Next, we will delve into the realm of common operations performed on variables without any interruption or delay.
Common Operations on Variables
Variable Manipulation is a fundamental concept in computer programming languages. It refers to the ability of these languages to handle and manipulate variables, which are containers for storing data values. This section explores how different programming languages accomplish variable manipulation through various techniques and features.
For instance, let’s consider a hypothetical scenario where we have a program that calculates the average temperature over a week. The program needs to store the daily temperatures in separate variables and perform calculations on them. In this case, variable manipulation allows us to efficiently manage the individual temperature values and perform arithmetic operations on them.
To facilitate variable manipulation, programming languages offer several common operations:
- Assignment: Assigning a value to a variable using an assignment operator.
- Arithmetic Operations: Performing mathematical computations on variables such as addition, subtraction, multiplication, and division.
- Comparison: Comparing variables or their values to determine relationships (e.g., equality, inequality).
- Concatenation: Combining string variables or literals together.
- Increased productivity: Variable manipulation simplifies complex tasks by providing convenient ways to modify and update data stored in variables.
- Enhanced flexibility: Programming languages’ support for variable manipulation empowers developers to adapt their code dynamically based on changing requirements.
- Improved efficiency: By utilizing efficient algorithms for manipulating variables, programs can execute faster and consume fewer system resources.
- Error reduction: Proper handling of variables reduces the likelihood of errors caused by incorrect data assignments or manipulations.
In summary, variable manipulation plays a vital role in computer programming languages as it enables efficient management and processing of data through various operations. Understanding how different programming languages handle variables allows developers to leverage these capabilities effectively. In the subsequent section about “Variable Declaration and Initialization,” we will delve into another crucial aspect related to working with variables without skipping a beat.
Variable Declaration and Initialization
Transition from the Previous Section:
Having explored common operations on variables, we now turn our attention to the crucial aspect of variable declaration and initialization. To illustrate this process, let’s consider a hypothetical scenario where a software developer is working on a weather forecasting application.
Variable Declaration and Initialization:
In order to accurately predict the weather conditions, our software developer needs to store different meteorological parameters such as temperature, humidity, wind speed, and precipitation. This involves declaring and initializing variables within their chosen programming language. Here are some key considerations when handling variables in computer programming languages:
-
Data Types: Each variable must be assigned an appropriate data type based on the nature of the information it will hold. For example, temperature might require a floating-point data type while humidity could be represented by an integer.
-
Naming Conventions: It is essential to adhere to naming conventions defined by the programming language being used. These conventions ensure code readability and maintainability across various projects.
-
Scope and Visibility: The scope of a variable determines its accessibility throughout different parts of the program. Understanding scoping rules is vital for preventing unintended side effects or conflicts between variables with similar names but different scopes.
-
Lifetime Management: Properly managing variable lifetimes helps optimize memory usage within programs. When a variable goes out of scope or is no longer needed, it can be deallocated to free up system resources.
Data Type | Description |
---|---|
Integer | Represents whole numbers without decimal places |
Floating-point | Allows representation of fractional values |
String | Stores sequences of characters |
By following these guidelines, programmers can effectively manipulate variables in computer programming languages, ensuring accurate storage and retrieval of data within their applications.
Transitioning into the subsequent section about “Scope and Lifetime of Variables,” understanding how variables behave within different scopes and their lifetimes is crucial for effective programming.
Scope and Lifetime of Variables
Having understood the process of variable declaration and initialization, we now delve into a crucial aspect of computer programming languages – how they handle variables. In this section, we explore various ways in which programming languages manipulate variables to store and retrieve data. To illustrate these concepts, let us consider an example scenario where a programmer is developing a weather application.
In our hypothetical weather application development scenario, the programmer needs to fetch real-time temperature data for different locations around the world. Here are some key points to understand about variable manipulation in computer programming languages:
-
Assignment Operators:
- Programming languages use assignment operators like “=” or “+=” to assign values to variables.
- For instance, if the programmer wants to store the temperature value 25 degrees Celsius in a variable named “temperature,” they would write:
temperature = 25;
-
Arithmetic Operations:
- Variables can be manipulated using arithmetic operations such as addition (+), subtraction (-), multiplication (*), and division (/).
- Suppose the programmer needs to convert the temperature from Celsius to Fahrenheit before displaying it on their weather application. They could use an equation like:
fahrenheit = (celsius * 9/5) + 32;
, where “celsius” represents the original temperature in Celsius.
-
Comparison Operators:
- Programming languages employ comparison operators like “==”, “!=”, “<“, “>” etc., to compare variables.
- In our weather application scenario, if the programmer wants to check whether the current temperature is greater than a threshold value (e.g., 30 degrees Celsius), they could use an expression such as:
if (temperature > 30) { /* code block */ }
.
-
Logical Operators:
- Logical operators such as “&&” (AND), “||” (OR), and “!” (NOT) allow programmers to combine conditions involving variables.
- Suppose the programmer wants to display a warning message if the temperature is either above 30 degrees Celsius or below 0 degrees Celsius. They could use an expression like:
if (temperature > 30 || temperature < 0) { /* code block */ }
.
Through these various methods of variable manipulation, programmers can effectively store, modify, and analyze data within their programs. In the subsequent section about “Data Types and Variable Manipulation,” we will explore how different programming languages handle specific data types and further extend our understanding of variable manipulation.
Data Types and Variable Manipulation
Transition from previous section: Building upon the understanding of scope and lifetime of variables, it is essential to examine how computer programming languages handle variable manipulation. By exploring this aspect, we can gain insight into the versatility and flexibility offered by these languages when working with variables.
In order to illustrate the significance of variable manipulation in computer programming languages, let us consider a hypothetical scenario. Imagine a software application that tracks inventory for an e-commerce platform. To efficiently manage stock levels, the application needs to keep track of various data points such as product names, quantities available, prices, and supplier information. The ability to manipulate variables allows developers to perform operations like updating stock levels or calculating revenue effortlessly.
When it comes to handling variables within programming languages, there are several key aspects worth considering:
- Variable assignment: One fundamental operation is assigning values to variables. This process involves storing specific data in memory locations associated with designated names or identifiers.
- Variable modification: Programming languages provide mechanisms for modifying existing variable values during program execution. These modifications may include incrementing or decrementing numeric values or concatenating strings.
- Variable scoping: As discussed previously, the concept of variable scope dictates where a particular variable is accessible within a program’s codebase.
- Variable naming conventions: While not directly related to manipulation itself, adhering to standard naming conventions improves code readability and maintainability.
Language | Assignment Syntax | Modification Syntax | Scoping Rules |
---|---|---|---|
Python | variable = value |
variable += 1 |
Lexical |
Java | type variable = value; |
variable++; |
Block |
JavaScript | var variable = value; |
variable += 1; |
Function |
C# | type variable = value; |
variable++; |
Block |
In conclusion, understanding how computer programming languages handle variables is crucial for efficient software development. By exploring the nuances of variable manipulation, we can harness the power of these languages to create robust and dynamic applications.
Transition to subsequent section: Now that we have examined how programming languages handle variables, it is important to delve into best practices for effective variable manipulation. Understanding these principles will aid in developing clean, maintainable code and optimizing program performance.
Best Practices for Variable Manipulation
Continuing our exploration of data types and variable manipulation, we now delve into how computer programming languages handle variables. By understanding this aspect, programmers can make informed decisions about choosing the most suitable language for their specific needs while ensuring efficient handling of variables.
Example:
To illustrate the importance of variable manipulation in different programming languages, let’s consider a hypothetical scenario where two developers are tasked with implementing a sorting algorithm. Developer A chooses to use Python, known for its simplicity and readability, whereas developer B opts for C++, renowned for its efficiency and low-level control over hardware resources.
Paragraph 1:
Each programming language has its own way of dealing with variables, including syntax conventions and memory management techniques. For instance, Python utilizes dynamic typing, allowing flexibility in assigning values to variables without explicit declaration of data types. On the other hand, C++ requires static typing, requiring developers to explicitly declare variable types before they can be used. This distinction affects not only code readability but also performance optimization during runtime execution.
Bullet Point List (emotional appeal):
- Streamlined coding process: Some programming languages provide built-in functions or libraries that simplify common tasks associated with variable manipulation.
- Memory efficiency: Certain languages employ automatic garbage collection mechanisms to manage memory allocation and deallocation effectively.
- Performance considerations: Low-level languages like assembly or C allow direct access to hardware resources, enabling fine-grained control over variable handling.
- Community support: The availability of extensive documentation and active online communities surrounding popular programming languages provides valuable resources for troubleshooting issues related to variable manipulation.
Table (emotional appeal):
Language | Variable Syntax | Memory Management |
---|---|---|
Python | Dynamic typing | Automatic garbage collection |
C++ | Static typing | Manual memory allocation/deallocation |
Assembly | Fixed registers | Direct hardware access |
Java | Object-oriented | Garbage collection |
Paragraph 2:
When deciding which programming language to use, developers must consider the trade-offs between ease of use, performance optimization, and memory efficiency. While high-level languages like Python offer simplicity and rapid development, they may sacrifice some runtime performance compared to low-level counterparts like C++. However, it’s worth noting that optimizing variable manipulation relies not only on language choice but also on adopting best practices for efficient coding.
In conclusion:
Understanding how computer programming languages handle variables is crucial for developers seeking to write efficient code. By comprehending the syntax conventions, memory management techniques, and performance considerations associated with different languages, programmers can make informed decisions about their toolset.