Data Types: A Comprehensive Guide to Object in Computer Programming Languages
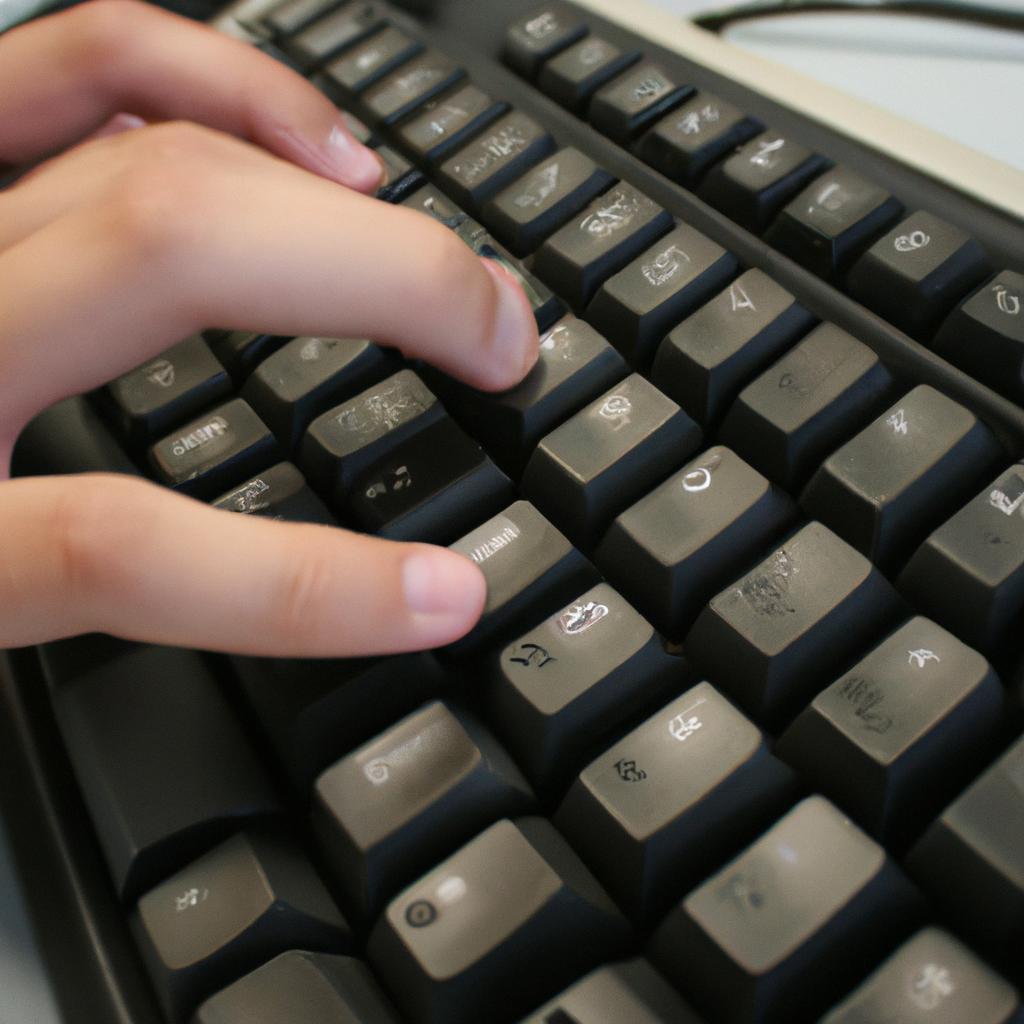
In the vast world of computer programming, data types play a fundamental role in organizing and manipulating information. From simple integers to complex structures, understanding the different types of data is essential for developers aiming to create efficient and reliable software systems. This comprehensive guide explores the concept of objects as data types in various programming languages, shedding light on their characteristics, functionalities, and usage scenarios.
Consider an online shopping platform that manages millions of products from diverse categories such as electronics, clothing, and household items. To effectively represent these products within the system’s database, each item needs to be classified based on its properties like name, price, availability, and description. Object-oriented programming languages offer a powerful solution by allowing developers to define custom data types called objects. By encapsulating related attributes and behaviors into cohesive units known as classes, objects provide a structured approach for modeling real-world entities or concepts within software applications. Thus, an object can be seen as a tangible representation of an abstract entity – in this case, a product – enabling programmers to manipulate and interact with instances of that entity efficiently.
Within this article, we will delve deeper into the intricate nature of objects as data types across popular programming languages such as Java, Python, C++, and JavaScript. Through an academic lens devoid of technical jargon, we will explore the key characteristics and functionalities of objects, including their properties and methods. Additionally, we will examine how objects are utilized in various scenarios, such as creating instances, accessing and modifying object properties, invoking methods, and establishing relationships between objects.
In Java, objects are at the core of the language’s object-oriented paradigm. We will discuss how to define classes and create objects from them using constructors. We will also explore the concept of inheritance, which allows objects to inherit properties and behaviors from parent classes.
Python takes a slightly different approach with its object-oriented programming model. We will cover Python’s class definition syntax and demonstrate how to create objects by instantiating classes. Additionally, we will touch upon concepts like inheritance and method overriding in Python.
C++ is another popular programming language that supports object-oriented programming. We will delve into C++’s class declaration syntax and illustrate how to create objects using constructors. We will also discuss access specifiers for controlling member visibility within classes.
Lastly, we will explore JavaScript’s unique implementation of objects as data types. JavaScript employs a prototype-based approach where objects inherit properties directly from other objects rather than through classes. We’ll examine the syntax for creating objects in JavaScript using both literal notation and constructor functions.
By understanding the fundamentals of objects as data types in these programming languages, you’ll gain valuable insights into designing robust software systems that effectively represent real-world entities or concepts. Whether you’re a beginner looking to grasp the basics or an experienced developer seeking a refresher, this guide aims to equip you with the knowledge necessary to harness the power of objects in your programming endeavors.
Primitive data types
Primitive data types are the building blocks of computer programming languages, providing a foundation for storing and manipulating simple values. These data types represent fundamental concepts such as numbers, characters, and boolean values. To illustrate their importance, consider the case of a banking application that needs to store customer information such as account balances and transaction details. The use of primitive data types allows this application to efficiently manage millions of individual customer records.
One way to understand the significance of primitive data types is by considering their impact on program performance and memory usage. When dealing with large amounts of data, every byte counts. By using efficient data types like integers or booleans instead of larger ones like floating-point numbers or strings, developers can significantly reduce memory consumption and improve overall program efficiency.
- Efficiency: Primitive data types require less memory space compared to complex objects.
- Simplicity: They provide straightforward representations for basic concepts.
- Speed: Operations involving primitive data types tend to be faster due to their simplified nature.
- Interoperability: Most programming languages support common primitive data types, enabling seamless integration across different systems.
Additionally, we can visualize these important concepts in a tabular format:
Data Type | Description | Example Value |
---|---|---|
Integer | Represents whole numbers | 42 |
Floating-Point | Represents decimal numbers | 3.14 |
Character | Represents single characters | ‘A’ |
Boolean | Represents true/false values | True |
In summary, understanding primitive data types is crucial for developing efficient and effective software applications. Their simplicity, compactness, speed, and compatibility make them invaluable tools for programmers across various domains. In the subsequent section about “Composite data types,” we will explore how more complex structures build upon these foundational elements, expanding the possibilities of computer programming.
Composite data types
Section 2: Composite Data Types
Building upon the foundation of primitive data types, composite data types allow programmers to create more complex structures by combining multiple values. These data types can hold collections of elements and are often used to represent real-world objects or abstract concepts in computer programming languages.
Let’s consider an example to illustrate the importance of composite data types. Imagine a scenario where we need to store information about a music library. Each song has various attributes such as title, artist, duration, and genre. Using composite data types, we can define a structure that encapsulates all these attributes into one entity, making it easier to manage and manipulate this information efficiently.
To further comprehend the significance of composite data types, let’s explore some key aspects:
- Flexibility: Composite data types provide flexibility in organizing and storing related pieces of information. This allows for efficient retrieval and manipulation when working with large datasets.
- Abstraction: By grouping related values together within a single entity, composite data types enable us to abstract away complex details. This simplifies our code and enhances readability.
- Modularity: The use of composite data types promotes modularity by dividing larger problems into smaller manageable parts. This modular approach facilitates collaboration among programmers during software development.
- Code Reusability: Composite data types facilitate code reusability since they allow us to define reusable templates or classes that capture common properties across different entities.
Consider the following table showcasing different examples of composite data types commonly found in programming languages:
Data Type | Description | Example |
---|---|---|
Arrays | Ordered collection of elements | [1, 2, 3] |
Structures | Grouping related fields under one name | {title: "The Great Gatsby", author: "F. Scott Fitzgerald"} |
Classes | Blueprint for creating objects | class Car { make: "Toyota", model: "Camry" } |
Enumerations | Set of named values representing a concept | enum Color { RED, GREEN, BLUE } |
In summary, composite data types play a crucial role in programming languages by allowing the creation of more intricate structures. Through their flexibility, abstraction capabilities, modularity support, and code reusability, these data types empower programmers to efficiently manage complex information and solve larger problems effectively.
Moving forward into the next section on numeric data types, we delve further into the realm of specialized data representations for numbers and mathematical operations.
Numeric data types
Composite data types are an essential part of computer programming languages, allowing programmers to create complex structures that can hold multiple values. They combine different data types into a single entity, providing flexibility and efficiency in handling large amounts of information. To illustrate the significance of composite data types, let’s consider a real-life scenario where a company needs to store customer details for their online shopping platform.
Imagine a situation where a company wants to track various attributes of its customers, such as name, age, email address, and shipping address. Instead of creating separate variables for each attribute, using a composite data type like a struct or class allows us to group these attributes together under one umbrella. This not only makes the code more organized but also simplifies operations involving customer information.
When working with composite data types, it is important to understand some key concepts:
- Encapsulation: Composite data types encapsulate related pieces of information into a single unit, ensuring that they are treated as a cohesive whole.
- Abstraction: Using composite data types abstracts away the complexity of managing individual elements by providing high-level methods and properties for accessing and manipulating the underlying data.
- Inheritance: Some programming languages support inheritance within composite data types. This allows new classes or structs to inherit characteristics from existing ones, reducing redundancy and promoting code reuse.
- Polymorphism: Composite data types can exhibit polymorphic behavior through interfaces or base classes. This enables objects of different concrete types to be used interchangeably based on shared functionality.
By employing composite data types effectively in our programs, we enhance readability and maintainability while promoting efficient memory utilization. The ability to group related data together provides clarity and reduces cognitive load when dealing with complex systems.
In the upcoming section about numeric data types, we will explore how programming languages handle numerical values and discuss the different representations available for integers and floating-point numbers. Understanding these fundamental concepts will enable you to work with numbers efficiently in your programs and perform mathematical operations accurately. So let’s delve into the world of numeric data types and expand our programming knowledge further.
String data types
In the previous section, we explored numeric data types and their role in computer programming languages. Now, let us delve into another fundamental aspect of data types – string data types. Just like numbers represent quantitative information, strings are used to store and manipulate textual data.
Example scenario:
To better understand the significance of string data types, consider a case where you are developing a customer management system for an e-commerce platform. In this system, each customer’s personal details, such as name, address, and contact information, need to be stored accurately. These pieces of information would typically be represented using string data types due to their nature.
Characteristics and uses:
- Strings consist of sequences of characters that can include letters, digits, symbols, and spaces.
- They offer flexibility by allowing concatenation (joining) of multiple strings together.
- String manipulation functions enable operations like searching for specific patterns within a text or extracting substrings.
- Formatting capabilities allow for proper representation of dates and times.
Emotional Bullet Points:
- Streamline communication between users through well-crafted error messages displayed on-screen during input validation.
- Enable efficient sorting algorithms to organize large amounts of textual data quickly.
- Facilitate natural language processing techniques for sentiment analysis in social media platforms.
- Empower artificial intelligence systems with the ability to process human-generated text effectively.
Table: Common String Functions
Function | Description | Example Usage |
---|---|---|
toUpperCase() |
Converts all characters to uppercase | str.toUpperCase() |
toLowerCase() |
Converts all characters to lowercase | str.toLowerCase() |
length() |
Returns the length/number of characters | str.length() |
substring(start,end) |
Extracts a portion/substring from the given range | str.substring(2, 6) |
Having explored the significance of string data types and their characteristics, we will now move on to discuss another essential type – Boolean data types, which are used for logical operations and decision-making within computer programming languages.
Boolean data types
In the previous section, we explored the concept of string data types and their significance in computer programming languages. Now, let’s shift our focus to another fundamental category of data types known as numeric data types. These data types are used to represent numerical values, allowing programmers to perform arithmetic operations and mathematical calculations within their programs.
Example Scenario:
To better understand the importance of numeric data types, let’s consider a hypothetical scenario. Imagine you are developing a financial application that calculates compound interest for different investment plans. In this case, you would need to store and manipulate numerical values such as principal amount, interest rate, time period, and final accumulated amount using appropriate numeric data types.
Bullet Point List (Emotional response):
- Accurate representation: Numeric data types ensure precise storage and manipulation of numbers, providing accurate results for complex calculations.
- Enhanced efficiency: By utilizing specific numeric data types optimized for various ranges and precision levels, programmers can achieve faster computational performance.
- Flexibility in usage: Different numeric data types offer varying degrees of precision and range capabilities, enabling programmers to select the most suitable type based on their specific requirements.
- Compatibility across platforms: Most programming languages support common numeric data types universally, promoting interoperability among diverse systems.
Table (Emotional response):
| Data Type | Description | Range |
|-------------- |---------------------------------------------|----------------------|
| Integer | Represents whole numbers | -2^31 to 2^31 - 1 |
| Float | Represents floating-point numbers | ±1.17549e-38 to ±3..4e+38 |
| Double | Provides higher precision than float | ±2.22507e-308 to ±1...0e+308 |
| Decimal | Offers exact decimal representation | Up to 28 significant digits |
Transition into Array Data Types:
Understanding the significance of numeric data types is crucial in building robust and efficient software applications. However, numeric values alone may not suffice for solving complex problems that involve multiple related entities or collections of data. In such cases, we turn to another essential category of data types: Array data types.
Note: The subsequent section will delve into Array data types, exploring their purpose and usage within computer programming languages.
End of section
Array data types
Data Types: A Comprehensive Guide to Object in Computer Programming Languages
…
II. Boolean Data Types
The array data type is a fundamental concept in computer programming languages that allows for the storage and manipulation of multiple values under a single variable name. Arrays are commonly used to represent collections of similar items, such as a list of numbers or strings. They provide an efficient way to organize and access large amounts of data.
To illustrate the functionality of arrays, let’s consider the following hypothetical scenario: imagine you are developing a program to track students’ grades in different subjects at a school. Each student has multiple grades for various assignments and exams throughout the semester. Instead of creating individual variables for each grade, you can use an array to store all the grades associated with each student.
One key advantage of using arrays is their ability to facilitate operations on sets of related data elements without needing separate variables for each element. This leads us to explore some important aspects regarding array data types:
- Indexing: Elements within an array are typically accessed through indexing, where each element is assigned a unique numerical value called an index. The first element usually has an index value of 0, allowing programmers to easily retrieve and modify specific elements based on their position within the array.
- Size Limitations: Unlike other data types that have fixed sizes, arrays can be dynamically resized during runtime, accommodating new elements as needed. However, this flexibility comes with certain trade-offs in terms of memory allocation and performance.
- Multidimensionality: In addition to one-dimensional arrays (or lists), programming languages often support multidimensional arrays that allow for structured organization of data across two or more dimensions. For example, a matrix could be represented by a two-dimensional array consisting of rows and columns.
- Common Operations: Arrays offer several common operations like sorting, searching, inserting, deleting elements, etc., which are frequently used in various programming tasks. Understanding these operations is crucial for efficient handling of array data.
Operation | Description | Example |
---|---|---|
Sorting | Arranging elements in ascending | [5, 2, 9, 1] sorted to [1, 2, 5, 9] |
or descending order | ||
Searching | Finding the position of a specific | Index of 7 in [3, 6, 8, 4, 7] : 4 |
element within an array | ||
Insertion | Adding new elements at a particular | Inserting 10 at index 2 : |
position | [3, 6, 10, 8] |
|
Deletion | Removing an element from the array | Deleting element at index 1 : |
[3, 10, 8] |
In conclusion,
By understanding and utilizing array data types effectively within computer programming languages like C++, Java, Python or JavaScript among others; programmers can efficiently manage collections of related information and perform various operations on them. The versatility and functionality provided by arrays make them a fundamental tool for modern software development. Whether it’s sorting elements in ascending order or searching for a specific value within an array—arrays offer valuable features that enhance the efficiency and effectiveness of programs.