Scope: Key Concepts in Computer Programming Languages>Variables
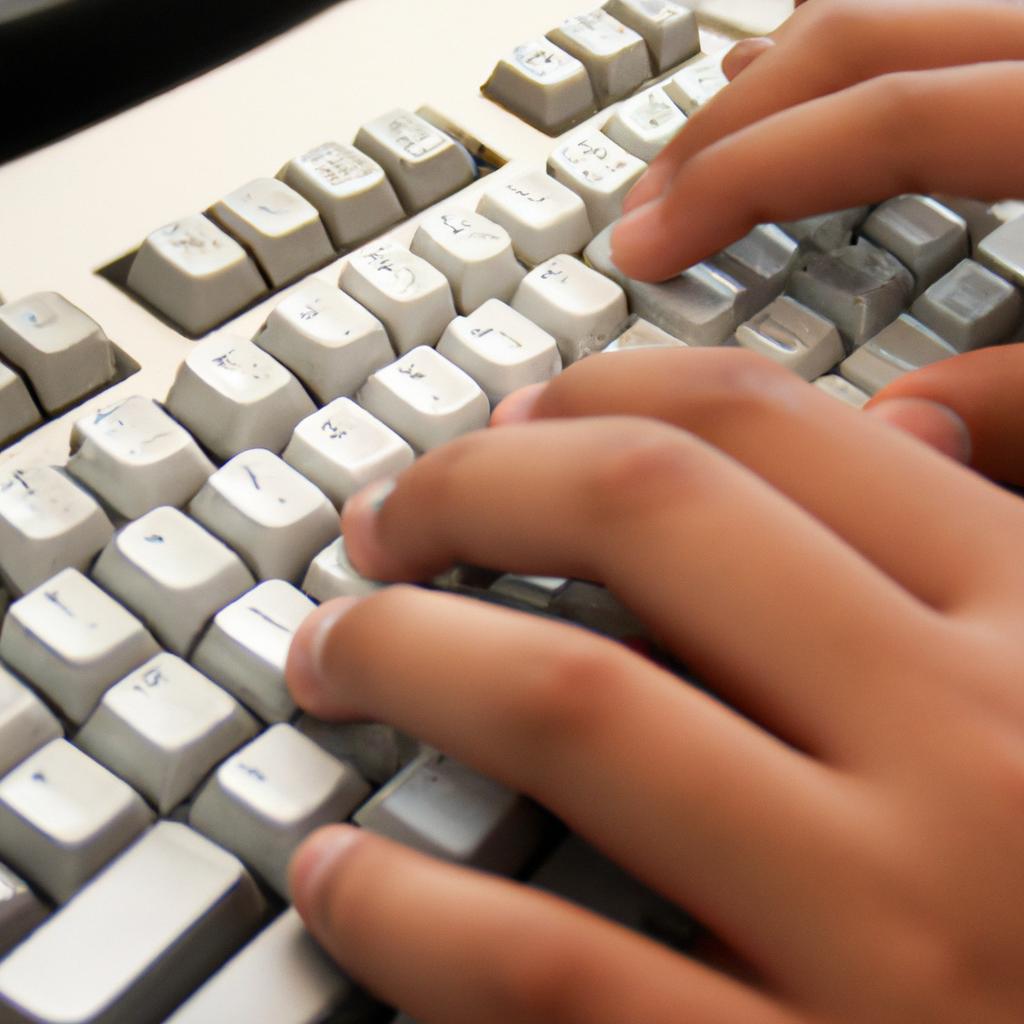
In the realm of computer programming languages, variables play a crucial role in defining and manipulating data. They serve as containers that hold values and can be accessed or modified throughout the program’s execution. Understanding the concept of variables is essential for any aspiring programmer, as it forms the foundation for writing effective and efficient code.
Consider a hypothetical scenario where a software developer is tasked with creating an inventory management system for a retail company. In this case, variables would be utilized to store information such as product names, quantities, prices, and other relevant details. By assigning appropriate variable names and values, the developer can ensure accurate tracking and manipulation of inventory data within the program.
To delve deeper into the significance of variables in computer programming languages, this article will explore key concepts related to their declaration, initialization, scope, and lifetime. Additionally, it will discuss different types of variables that exist in various programming languages and highlight best practices for utilizing them effectively. By gaining a comprehensive understanding of variables’ functionality and usage principles, programmers can enhance their ability to write robust code that fulfills specific requirements while optimizing resource utilization.
Definition of Scope in Computer Programming Languages
In computer programming languages, the concept of scope refers to the visibility and accessibility of variables within a program. It determines where a variable can be accessed or referenced throughout the code. To better understand this concept, let’s consider an example scenario:
Imagine you are tasked with creating a program that calculates the average score of students in a class. Within this program, you would need to define variables such as totalScore
, numberOfStudents
, and averageScore
. The concept of scope comes into play when determining where these variables can be used and accessed.
One important aspect of understanding scope is recognizing different levels at which variables can be declared. These levels include global scope, local scope, function scope, and block scope. Each level has its own set of rules regarding variable accessibility.
To provide a clearer picture, here is a markdown-formatted bullet point list highlighting key aspects related to the notion of scope:
- Global Scope: Variables declared outside any functions or blocks have global scope. They are accessible from anywhere within the program.
- Local Scope: Variables declared inside a specific function have local scope. They are only accessible within that particular function.
- Function Scope: Similar to local scope, but applies specifically to named functions that contain their own set of variables.
- Block Scope: Introduced by certain statements like loops or conditional statements (e.g., if/else). Variables defined within these blocks have limited visibility restricted to those blocks.
To further illustrate how these scopes work in practice, consider the following table:
Variable Name | Global Scope | Local Scope |
---|---|---|
totalScore | Yes | No |
numberOfStudents | Yes | No |
averageScore | Yes | Yes |
As we move forward and delve into our exploration of variables’ role in programming languages, it’s crucial to grasp the concept of scope. By understanding the visibility and accessibility of variables within a program, we can effectively design and develop reliable software solutions without encountering unexpected errors or conflicts.
Next, let’s explore how variables contribute to the overall functionality of programming languages.
Understanding the Role of Variables in Programming Languages
Building upon the previous section’s discussion on scope, it is essential to delve into the significance of variables within programming languages. To illustrate this concept, let us consider a hypothetical scenario where we are developing a weather application that displays current temperature data for various cities around the world. In order to accurately represent each city’s temperature, we would need to utilize variables.
Variables play a crucial role in storing and manipulating data within computer programs. They act as containers that hold different values during program execution. For instance, when designing our weather application, we could assign a variable called “temperature” to store the current temperature value of a specific city. By utilizing variables, programmers can create dynamic applications that adapt to changing circumstances or user input.
To emphasize the importance of variables further, here are some key points:
- Flexibility: Variables allow programmers to write more flexible code by enabling them to reuse and modify stored values throughout their programs.
- Dynamic Behavior: Through variables, program behavior can be altered based on conditions or inputs provided at runtime.
- Efficiency: Utilizing variables enables efficient use of memory resources by allowing data storage only when needed.
- Simplicity: By assigning meaningful names to variables, code readability improves significantly.
Let us highlight these benefits through the following table:
Benefit | Description |
---|---|
Flexibility | Enables reusability and modification of values |
Dynamic Behavior | Adapts program behavior based on runtime conditions |
Efficiency | Optimizes memory usage by storing data only when necessary |
Simplicity | Enhances code readability with meaningful variable names |
In conclusion, understanding how variables function in programming languages is vital for creating effective and efficient software solutions. Through their ability to store and manipulate data dynamically, they provide flexibility and simplify complex coding tasks. The next section will explore types of scope: global versus local variables, shedding light on the different ways variables can be accessed within a program.
Types of Scope: Global vs. Local Variables
In the previous section, we explored the fundamental concept of variables in programming languages. Now, let’s delve deeper into this topic by examining key concepts related to variables and their significance.
Consider a scenario where you are developing a web application that requires storing user information, such as names and email addresses. In order to accomplish this task, you would need to create variables to hold these values temporarily. For instance, you might have a variable called “name” that stores the user’s name and another variable named “email” for holding their email address. These variables allow you to manipulate and access the stored data easily throughout your program.
To further understand the importance of variables in programming languages, let’s explore some key points:
- Variables provide flexibility: By using variables, programmers can store different types of data (e.g., numbers, strings) and modify them as needed during runtime.
- Efficient memory management: Variables help optimize memory usage by allocating space only when necessary.
- Enhanced code readability: The use of well-named variables improves code comprehension and makes it easier for other developers to collaborate on projects.
- Debugging assistance: When encountering errors or bugs in a program, having clearly defined variables allows for efficient troubleshooting.
Let’s summarize the main takeaways from this discussion with a table highlighting the benefits of utilizing variables:
Benefits of Using Variables |
---|
Flexibility |
Efficient Memory Management |
Improved Code Readability |
Debugging Assistance |
As we move forward, our focus will shift towards exploring scoping rules and variable accessibility. Understanding how these elements operate is crucial for effectively working with variables within computer programming languages.
Scoping Rules and Variable Accessibility
Transition from Previous Section:
Having explored the concepts of global and local variables, it is now imperative to delve into scoping rules and variable accessibility. Understanding these principles will allow programmers to effectively manage variables within their programs.
Scoping Rules and Variable Accessibility
To comprehend how programming languages handle scoping, consider a hypothetical scenario in which you are tasked with creating a program that calculates the average temperature for each day of the week. As a diligent programmer, you define variables such as “temperature” and “averageTemp” to store relevant values. However, an issue arises when there are multiple instances of these variables throughout your program. This is where scoping rules come into play.
One way programming languages address this challenge is through lexical scoping or static scoping. Lexical scoping establishes the scope of a variable based on its position within the source code. In our example, if you declare the “temperature” variable inside a loop block, its scope will be limited to that specific block only. On the other hand, if you declare it outside of any blocks (e.g., at the beginning of your program), it becomes accessible throughout your entire program.
To grasp the intricacies of scoping rules more comprehensively, let us consider some key points:
- Scopes can be nested within one another; inner scopes have access to variables declared in outer scopes.
- Variables defined in inner scopes may shadow similarly named variables in outer scopes.
- Scope resolution follows a hierarchical structure, flowing from innermost to outermost scopes.
- Programming languages differ in their specific implementation of scoping rules; understanding language-specific nuances is crucial for effective coding.
Language | Default Scope | Notable Features |
---|---|---|
Python | Local | Global keyword enables explicit global declarations |
JavaScript | Function | Block-level scope introduced with ES6 |
C++ | Block | Namespaces provide additional scope management |
Java | Class | Access modifiers restrict variable accessibility |
In conclusion, understanding scoping rules and variable accessibility is vital for proficient programming. By adhering to these principles, programmers can prevent naming conflicts, improve code organization, and optimize the use of variables within their programs.
Transition to Next Section:
Having established a foundational understanding of scoping rules and variable accessibility, let us now delve into an equally crucial aspect – scope resolution. This will shed light on how programming languages determine the values of variables in different scopes.
Scope Resolution: How Programming Languages Determine Variable Values
In the previous section, we discussed scoping rules and variable accessibility in programming languages. Now, let’s delve into the concept of scope resolution and how it affects the determination of variable values. To illustrate this, consider a hypothetical scenario where you are working on a program that calculates the total revenue of a company based on various factors such as sales, expenses, and taxes.
When a programming language encounters a variable name within a specific context or block of code, it needs to determine which value should be assigned to that variable. This process is known as scope resolution. The language follows certain rules to identify the correct variable value based on its visibility within different scopes.
To better understand scope resolution, let’s explore some key aspects:
-
Lexical Scoping: Many programming languages use lexical scoping, also known as static scoping. In this approach, variables are resolved by examining their position in the source code rather than at runtime. As a result, each block of code inherits access to variables defined in its outer blocks.
-
Shadowing: Sometimes, multiple variables with the same name exist in nested scopes. When this happens, the innermost variable shadows (hides) any similarly named variables from higher-level scopes until it goes out of scope again.
-
Scope Hierarchy: Variables can have global or local scope depending on their declaration location. Global variables are accessible throughout the entire program, whereas local variables are limited to specific functions or blocks.
-
Ambiguity Resolution: If there is ambiguity between two or more variables with similar names but different scopes, most programming languages follow explicit resolution rules based on precedence levels or namespaces.
The table below summarizes these concepts for easy reference:
Concept | Description |
---|---|
Lexical Scoping | Variables resolved by examining their position in source code |
Shadowing | Innermost variable hides similarly named variables from higher-level scopes |
Scope Hierarchy | Global variables accessible throughout program, local variables limited to specific functions/blocks |
Ambiguity Resolution | Explicit rules for resolving ambiguity between similar-named variables with different scopes |
Understanding scope resolution is crucial in programming as it ensures accurate and predictable behavior of variable assignments. By following the language-specific rules, programmers can effectively manage variable values within their programs.
Moving forward, let’s explore common mistakes and best practices related to variable scope that developers should keep in mind when writing code.
Common Mistakes and Best Practices in Variable Scope
Transitioning from the previous section that discussed how programming languages determine variable values, we now delve into the concept of variable scope. Understanding variable scope is crucial in computer programming as it defines the accessibility and lifespan of variables within a program. By examining different approaches to variable scope, programmers can effectively manage their code and avoid potential errors.
Consider the following example scenario: In a web development project, multiple functions are implemented to handle user interactions. Each function requires access to certain variables defined outside its own block of code. The question arises: How do programming languages determine which variables are accessible within each function? This challenge highlights the significance of understanding variable scope.
To provide clarity regarding variable scope, let us explore some key concepts:
-
Global Scope:
- Variables declared at the highest level of a program.
- Available throughout the entire program.
- Can be accessed by any function or block within the program.
-
Local Scope:
- Variables declared within a specific function or block.
- Limited to that particular function or block’s scope.
- Cannot be accessed by other functions or blocks unless explicitly passed as parameters.
-
Block Scope:
- Introduced in modern programming languages like JavaScript with ‘let’ and ‘const’ keywords.
- Variables declared within a set of curly braces {} have block-level scope.
- Accessible only within that specific block.
-
Lexical Scope:
- Also known as static scoping.
- Determines variable accessibility based on where they are declared in source code rather than runtime context.
By comprehending these concepts, programmers gain insight into how variables are scoped and managed within their programs. Utilizing proper scoping techniques enables developers to write more efficient and maintainable code while minimizing conflicts between variables used across various parts of their programs.
In summary, grasp