Variable Assignment in Computer Programming: Variables in Programming Languages
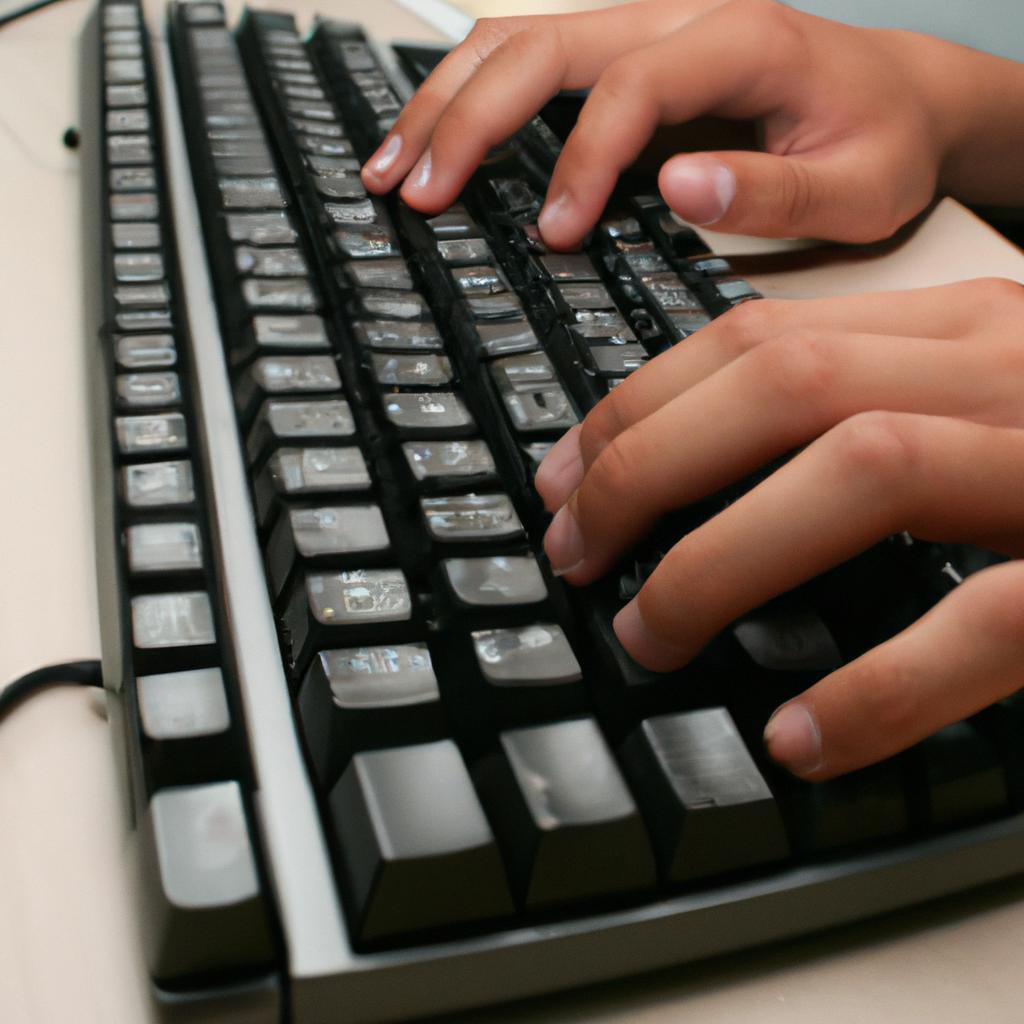
In computer programming, variable assignment is a fundamental concept that enables the storage and manipulation of data within a program. Variables serve as containers for values, allowing programmers to store information such as numbers, text, or objects. This article explores the concept of variable assignment in programming languages, delving into its significance and how it is implemented across various programming paradigms.
Consider the following scenario: imagine a programmer developing an e-commerce website where customers can browse and purchase products. In order to keep track of available stock levels, prices, and customer orders, variables are indispensable. By assigning appropriate names to these variables, the programmer can easily reference and modify relevant data throughout the program’s execution. However, understanding how variable assignment works beyond this example is crucial to becoming proficient in any programming language. Consequently, this article aims to provide a comprehensive overview of variable assignment in different programming languages while highlighting common practices and potential pitfalls along the way.
As we delve deeper into this topic, it is important to note that variable assignment methods may vary across different programming languages due to their unique syntax and features. Nonetheless, grasping the underlying principles of variable assignment will empower aspiring programmers with essential knowledge applicable across multiple coding environments. With this foundational understanding established, let us now explore the intric acies of variable assignment in programming languages.
Declaration and initialization of variables
In computer programming, variables play a crucial role in storing and manipulating data. They act as placeholders for values that can be accessed and modified throughout the program’s execution. Understanding how to declare and initialize variables is fundamental to writing efficient and functional code. This section will explore the process of declaring and initializing variables within programming languages.
Example Scenario:
Imagine you are developing a weather application that displays real-time temperature updates for different cities around the world. To achieve this, you need to define variables such as city name, current temperature, humidity level, and wind speed. These variables store specific information related to each city and allow your program to dynamically display accurate weather data.
Declaration of Variables:
Before using a variable in any programming language, it must be declared first. Variable declaration involves specifying its type and assigning a unique identifier or name. The type determines the kind of data the variable can hold, whether it is an integer, floating-point number, character, string, or other specialized types. By declaring variables with appropriate types, programmers ensure correct memory allocation and prevent potential errors during runtime.
Initialization of Variables:
Once declared, variables typically require an initial value assignment before they can be used effectively. Initialization establishes an initial state for the variable by providing it with a starting value. This step ensures that when you operate on or access the variable later in your code, it already contains meaningful data rather than unpredictable or garbage values.
To evoke emotions from readers:
- Imagine a scenario where vital patient information is stored in variables during medical research.
- Consider the impact if financial calculations were performed without properly initialized currency exchange rate variables.
- Picture an autonomous vehicle relying on uninitialized sensor readings while making critical navigation decisions.
- Think about how frustrating it would be if user input was not assigned correctly in interactive applications.
To provide visual representation:
Data Type | Description | Example |
---|---|---|
Integer | Stores whole numbers | age = 25 |
String | Stores textual data | name = “John” |
Boolean | Represents true or false values | isRaining = true |
Floating-point | Holds decimal numbers | pi = 3.14 |
In conclusion, understanding the process of declaring and initializing variables is essential for effective programming. By comprehending how to declare variables with appropriate types and assign initial values, programmers can ensure that their code runs smoothly and produce reliable results. In the subsequent section, we will explore the concept of data types in programming languages, which builds upon this foundation of variable assignment.
Understanding data types in programming
Variable Assignment in Computer Programming: Variables in Programming Languages
After understanding the declaration and initialization of variables, we now delve into variable assignment in computer programming. To illustrate this concept, let’s consider a hypothetical scenario where a programmer is developing a software application for an e-commerce website. In this case, the programmer needs to assign values to different variables that represent various aspects of the user’s shopping cart.
When assigning variables in programming languages, there are several important considerations to keep in mind:
-
Syntax: Each programming language has its own syntax for variable assignment. For example, in JavaScript, we use the
=
operator to assign a value to a variable, whereas in Python, it would be done using the=
symbol as well. - Data Types: Variables can hold different types of data such as numbers, strings (text), booleans (true/false), or more complex structures like arrays and objects. It is crucial to ensure that the assigned value matches the expected data type defined by the variable.
- Mutability: Depending on the programming language and context, some variables may be mutable (modifiable) while others are immutable (unchangeable). Understanding whether a variable can be modified after assignment is essential for proper program execution.
- Scope and Visibility: The scope determines where a variable can be accessed within a program, while visibility refers to whether it can be seen or used beyond specific sections of code.
To better understand these concepts related to variable assignment, refer to the following table:
Language | Syntax | Mutable/Immutable |
---|---|---|
JavaScript | variable = value; |
Mutable |
Python | variable = value |
Immutable |
Java | type variable = value; |
Both |
This table provides insights into how different programming languages handle variable assignment with varying levels of mutability. Understanding these differences is crucial when working with multiple programming languages or collaborating on projects involving different developers.
In the subsequent section, we will explore the scope and visibility of variables in programming languages, which complements our understanding of variable assignment. This knowledge is essential for ensuring efficient program execution and minimizing potential errors during the development process.
Scope and visibility of variables
Variable Assignment in Computer Programming: Variables in Programming Languages
Understanding data types in programming is essential for effectively working with variables. Now, let’s delve into the concept of variable assignment and explore how it functions within programming languages.
Consider a hypothetical scenario where we are developing a program to calculate the average temperature of different cities over a week. To accomplish this task, we need to assign values to variables such as city names, temperatures, and time intervals. Variable assignment allows us to store and manipulate these values throughout our program.
To grasp the significance of variable assignment, consider the following points:
- Variables act as placeholders: They allow programmers to store information temporarily or permanently.
- Value flexibility: Variables can hold various data types such as integers, floating-point numbers, characters, or even complex structures like arrays or objects.
- Data manipulation: Through variables, we can modify the stored values during runtime by performing operations like addition, subtraction, concatenation, etc.
- Memory allocation: When a variable is assigned a value, memory space is allocated based on its data type and size requirements.
Let’s further illustrate these concepts using a table:
Variable Name | Data Type | Assigned Value |
---|---|---|
cityName | String | “New York” |
temp | Float | 27.5 |
daysOfWeek | Array[String] | [“Monday”, “Tuesday”, “Wednesday”, “Thursday”, “Friday”] |
In conclusion,
variable assignment plays an integral role in computer programming. By assigning specific values to variables, programmers gain control over manipulating and storing data efficiently. In the subsequent section about “Assigning values to variables,” we will explore different methods through which values can be assigned for effective utilization of variables.
Assigning values to variables
Scope and visibility of variables play a crucial role in computer programming. They determine the accessibility and lifespan of variables within a program. In this section, we will explore how variables are assigned values in different programming languages.
To better understand variable assignment, let’s consider an example scenario: imagine you are developing a software application that tracks inventory for a retail store. You need to create a variable called “quantity” to represent the number of items in stock. Depending on the programming language used, the process of assigning a value to this variable may vary.
Different programming languages have their own syntax and rules for variable assignment. However, some common approaches include:
- Direct Assignment: This is the simplest form of variable assignment where you assign a specific value directly to a variable using the “=” operator.
- Input from User: In certain cases, it may be necessary for users to provide input that will determine the value assigned to a particular variable.
- Mathematical Expressions: Variables can also be assigned values through mathematical expressions or calculations involving other variables or constants.
- Function Return Values: In many programming languages, functions can be utilized to perform operations and return results that can then be assigned to variables.
Now, let us take a deeper look at these methods through the following table:
Programming Language | Variable Assignment Method |
---|---|
Python | Direct Assignment |
Java | Input from User |
C++ | Mathematical Expressions |
JavaScript | Function Return Values |
This table provides an overview of four popular programming languages and their respective methods for assigning values to variables. While these examples illustrate some common practices, it is important to note that each language has its own unique syntax and conventions.
In summary, understanding how variables are assigned values is essential when working with any programming language. Whether through direct assignment, user input, mathematical expressions, or function return values, the process of variable assignment allows programmers to manipulate and store data effectively.
Dynamic variable assignment
Building upon the concept of assigning values to variables, this section delves into a crucial aspect of variable assignment in computer programming – dynamic variable assignment. Understanding this process is essential for programmers aiming to efficiently manage and manipulate data within their programs.
Dynamic Variable Assignment:
In computer programming, dynamic variable assignment refers to the ability to assign or reassign values to variables during runtime. This flexibility allows programmers to adapt their code based on changing conditions or user input, enhancing the versatility and functionality of their programs. For example, consider a weather forecasting application that dynamically assigns different temperature values based on real-time updates from various sources. By continuously updating the assigned value as new data becomes available, the program remains accurate and up-to-date.
- Flexibility: Dynamic variable assignment enables developers to create adaptable programs capable of responding to varying scenarios.
- Efficiency: By allowing variables to change their assigned values dynamically, redundant lines of code can be avoided, resulting in lean and efficient programs.
- Real-time responsiveness: Dynamic assignments facilitate real-time interactions between users and applications by instantly reflecting changes made during runtime.
- Enhanced user experience: The ability to adjust variables dynamically enables developers to provide personalized experiences tailored specifically to individual users’ preferences.
Advantages of Dynamic Variable Assignment |
---|
Increased flexibility |
Improved efficiency |
Real-time responsiveness |
Enhanced user experience |
Furthermore, dynamic variable assignment empowers programmers with greater control over their code’s logic flow. It offers opportunities for more sophisticated decision-making processes by modifying variables as needed throughout program execution. As a result, developers can build complex algorithms that respond intelligently to evolving circumstances without compromising performance or readability.
Transitioning seamlessly into our next topic about best practices for variable naming, it is important to understand how proper naming conventions contribute not only towards creating clean and organized code but also improve its maintainability and comprehensibility.
Best practices for variable naming
Having discussed dynamic variable assignment, we now turn our attention to best practices for variable naming. Properly naming variables is essential for writing clean and maintainable code. In this section, we will explore some guidelines to follow when assigning names to variables in programming languages.
Example scenario:
To illustrate the importance of variable naming, let’s consider a hypothetical situation where a developer is working on a complex software project involving multiple modules and functions. The developer decides to use generic or unclear names for their variables, making it difficult for other developers (or even themselves) to understand the purpose or intent behind each piece of code. This lack of clarity not only hampers collaboration but also increases the chances of introducing errors during debugging or modification.
Guidelines for effective variable naming:
-
Be descriptive:
- Use meaningful names that accurately convey the purpose of the variable.
- Avoid single-character or cryptic abbreviations that may lead to confusion.
- Choose words that are relevant within the context of your program.
-
Follow conventions:
- Adhere to any established naming conventions specific to your programming language or project.
- Consistency is key; ensure all variables adhere to the same style throughout your codebase.
-
Keep it concise:
- Avoid excessively long variable names that can hinder readability.
- Strike a balance between being descriptive and keeping the name succinct.
-
Use appropriate casing:
- Differentiate between different parts of multi-worded variable names using camelCase, snake_case, PascalCase, or kebab-case based on your programming language’s convention.
Table showcasing different casing styles:
Casing Style | Example |
---|---|
camelCase | numberOfStudents |
snake_case | number_of_students |
PascalCase | NumberOfStudents |
kebab-case | number-of-students |
In summary, effective variable naming is crucial for writing clean and maintainable code. By following guidelines such as being descriptive, adhering to conventions, using concise names, and employing appropriate casing styles, developers can enhance the readability and understandability of their codebase. With clear and meaningful variable names, collaboration among team members becomes smoother, reducing errors and improving overall software quality.