Variable Declaration in Computer Programming Languages: A Comprehensive Guide
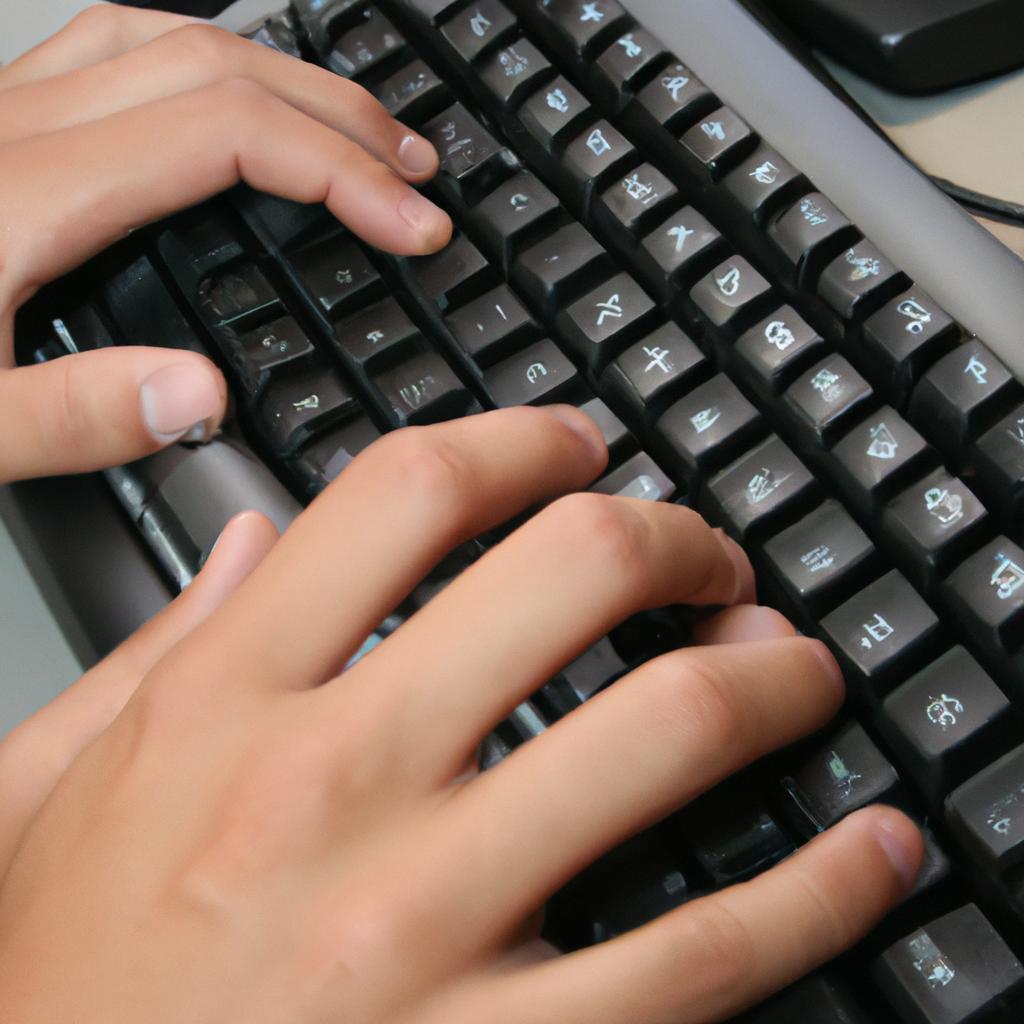
Variable declaration is a fundamental concept in computer programming languages, serving as the foundation upon which programs are built. It involves assigning a name to a memory location and specifying its data type, allowing programmers to manipulate and store values for use throughout their code. For instance, consider the case of a hypothetical e-commerce application that needs to track inventory levels. By declaring variables such as “productID” and “quantity”, the program can efficiently manage stock information and update it dynamically based on customer purchases or restocking activities.
Understanding variable declaration is crucial for aspiring programmers as it establishes the groundwork for writing efficient and functional code. This comprehensive guide aims to provide insights into different aspects of variable declaration across various programming languages. Emphasizing an academic tone devoid of personal pronouns, this article will explore key topics including variable naming conventions, data types, scoping rules, initialization techniques, and best practices in order to equip readers with a thorough understanding of how to effectively declare variables in their chosen language. Whether one’s interest lies in Java, Python, C++, or any other popular programming language, this guide strives to offer clear explanations accompanied by illustrative examples to aid comprehension and facilitate practical implementation.
Syntax of Variable Declaration
Consider the following example: imagine a scenario where a programmer is tasked with developing a software application that calculates and displays the average temperature of a given city. In order to accomplish this task, the programmer needs to declare variables to store information such as city name, temperature values, and the final calculated result. This initial step in computer programming is known as variable declaration.
Variable declaration refers to the process of reserving memory space for storing data within a program. It involves specifying both the type and name of each variable. The syntax used for declaring variables varies across different programming languages; however, there are some common principles that can be observed.
To provide clarity on these principles, here are several key points regarding the syntax of variable declaration:
- Variables must be declared before they can be used.
- Each variable should have a unique identifier or name assigned to it.
- The data type determines what kind of values can be stored in a particular variable.
- Some programming languages require explicit initialization of variables, while others automatically assign default values if no initial value is specified.
Such rules ensure consistency and efficiency when working with variables in computer programs. By adhering to proper syntax guidelines, programmers reduce errors and facilitate better understanding among team members who may collaborate on code development.
In the upcoming section about “Data Types and Variable Declaration,” we will delve into more detail about various types of data that can be stored within variables and how they impact program execution. Understanding these concepts will further enhance our comprehension of variable declaration in computer programming languages.
Data Types and Variable Declaration
Transitioning from the previous section on the syntax of variable declaration, we now delve into an equally important aspect of computer programming languages: the scope and lifetime of variables. Understanding these concepts is crucial for writing efficient and bug-free code.
To illustrate the significance of scope and lifetime in variable declaration, consider a scenario where a programmer is developing a web application that requires authentication. In this case, the programmer may declare a “username” variable to store user input during the login process. The scope of this variable would be limited to the specific function or block where it is declared, ensuring its availability only within that particular context.
When discussing scope and lifetime, several key points should be considered:
-
Visibility: A variable’s visibility refers to which parts of a program can access it. Depending on how variables are declared, they can have different scopes such as global (accessible throughout the entire program), local (limited to a specific block or function), or class-level (accessible within objects instantiated from a certain class).
-
Duration: The duration or lifetime of a variable determines when it is created and destroyed. Variables with automatic storage duration are created when their enclosing block starts executing and cease to exist once the block completes execution. On the other hand, variables with static storage duration persist throughout the entire program’s execution.
-
Shadowing: Another essential concept related to scope is shadowing. It occurs when two variables with the same name exist in nested blocks but have different scopes. In such cases, the innermost variable shadows any outer variables with identical names until its own scope ends.
To better understand these principles, let us examine them through an example table:
Variable Type | Scope | Lifetime |
---|---|---|
Global | Whole Program | Entire Execution |
Local | Specific Function/Block | Block Execution |
Class-Level | Objects Instantiated From a Class | Object Lifetime |
In summary, the scope and lifetime of variables play a vital role in programming languages. By properly managing variable declarations, programmers can enhance code readability, avoid naming conflicts, and optimize resource usage. In the subsequent section on “Scope and Lifetime of Variables,” we will explore more advanced concepts related to these aspects.
Transitioning into the subsequent section about “Scope and Lifetime of Variables,” we continue our exploration of crucial considerations in computer programming that further contribute to efficient program design.
Scope and Lifetime of Variables
In the previous section, we discussed data types and variable declaration in computer programming languages. Now, let’s delve into the important concept of scope and lifetime of variables. To illustrate this concept, consider a scenario where you are writing a program to calculate the total cost of items purchased by customers in an online store.
The scope of a variable refers to its visibility or accessibility within different parts of a program. For instance, if you declare a variable inside a function, it will have local scope and can only be accessed within that specific function. On the other hand, if you declare a variable outside any functions, it will have global scope and can be accessed throughout the entire program. This allows for modular code organization while also minimizing naming conflicts between variables.
Now let’s explore the lifetime of variables. The lifetime of a variable is determined by how long it exists during program execution. Local variables typically have shorter lifetimes as they are created when their enclosing block begins executing and destroyed when the block ends. Conversely, global variables tend to have longer lifetimes as they persist throughout the entire duration of the program.
To better understand these concepts, here are some key points to keep in mind:
- Variables with local scope are known as automatic variables.
- Automatic variables are allocated on the stack memory.
- Global variables occupy memory space throughout the execution of the program.
- Memory management is crucial to prevent issues like memory leaks or accessing invalid memory locations.
Variable Type | Scope | Lifetime |
---|---|---|
Automatic | Limited | Block duration |
Global | Entire Program | Program duration |
Understanding scope and lifetime is essential for efficient coding practices and ensuring proper utilization of system resources. In our next section about initializing variables, we will explore how values can be assigned to declared variables right from their inception without leaving them uninitialized or containing garbage values.
Now, let’s move on to the subsequent section about “Initializing Variables” where we will explore how variables can be given initial values.
Initializing Variables
In the previous section, we explored the concept of scope and lifetime in relation to variables. Now, let us delve deeper into the crucial aspect of initializing variables. To illustrate this further, consider a hypothetical scenario where you are developing a program that calculates the average temperature for a given week. In order to perform this calculation accurately, you would need to declare and initialize variables such as temperature
and sum
.
Initializing variables is an essential step in computer programming languages. By assigning an initial value to a variable during declaration, you ensure its proper functioning within your program. Here are some key points to keep in mind:
- Initialization prevents potential bugs: When you initialize a variable with an appropriate value at the outset, you reduce the risk of unexpected behavior or errors later on in your code.
- Default initialization: If no explicit initialization is provided by the programmer, most programming languages will assign a default value based on data type (e.g., 0 for integers or null for objects).
- Best practices for initialization: It is considered good practice to always explicitly initialize variables to avoid any ambiguity or confusion. This promotes readability and maintainability of your code.
To better understand how initialization works across different programming languages, refer to the following table:
Programming Language | Default Value |
---|---|
Java | 0 |
C++ | Unspecified |
Python | None |
JavaScript | undefined |
By examining these default values, programmers can make informed decisions about when and how they should initialize their variables effectively.
In summary, initializing variables is a critical step in computer programming languages as it ensures accurate execution of programs while minimizing errors. By providing an initial value during declaration, programmers prevent bugs and improve code quality. In our next section on “Constants and Variable Declaration,” we will explore another vital aspect related to variable management and usage.
[Next section: Constants and Variable Declaration]
Constants and Variable Declaration
Transitioning from the previous section on initializing variables, we now delve into the topic of constants and variable declaration in computer programming languages. To better understand this concept, let’s consider an example scenario where a software developer is creating a program to calculate the average temperature for a given week. In this case, they would need to declare variables such as “temperature” and “daysOfWeek” to store the necessary data.
When it comes to declaring variables in computer programming languages, there are several important considerations:
-
Data Type Specification:
- Variables should be declared with their appropriate data types (e.g., integer, float, string) to ensure efficient memory allocation.
- This helps prevent potential errors caused by mismatched data types during operations or assignments.
-
Naming Conventions:
- Meaningful and descriptive names aid in code readability and maintainability.
- It is recommended to use camelCase or snake_case formatting conventions for variable naming.
-
Scope Rules:
- Understanding scope rules ensures that variables are accessible within the appropriate context.
- Local variables have limited visibility within a specific block of code, while global variables can be accessed throughout the entire program.
-
Constant Declaration:
- Constants are immutable values whose values cannot be modified once assigned.
- By using constants instead of regular variables when applicable, developers can ensure consistency and prevent accidental modifications.
To further illustrate these considerations, here is a table demonstrating different variable declarations used in common programming languages:
Language | Syntax | Example |
---|---|---|
Python | variable = value |
temperature = 25 |
Java | type name = value; |
int daysOfWeek = 7; |
JavaScript | let name = value; |
const PI = 3.14; |
In summary, constants and variable declaration play a crucial role in computer programming. By following best practices such as specifying data types, using meaningful names, understanding scope rules, and utilizing constants appropriately, developers can create more readable and maintainable code. In the subsequent section on “Best Practices for Variable Declaration,” we will explore additional techniques to enhance the efficiency and effectiveness of variable handling within programming languages.
Best Practices for Variable Declaration
Transitioning seamlessly from the previous section, let us explore further aspects of variable declaration in computer programming languages. To illustrate its importance, consider a hypothetical scenario where a software developer is tasked with designing an application that calculates and displays real-time stock market prices. In this case, the variables representing different stocks would need to be declared accurately to ensure accurate calculations and timely updates.
When declaring variables in programming languages, it is crucial to follow best practices to optimize code readability, maintainability, and performance. Here are some key considerations:
-
Descriptive Naming Convention:
- Use meaningful names for variables that reflect their purpose or content.
- Avoid ambiguous or generic labels like “temp” or “x.”
-
Proper Initialization:
- Initialize variables at the point of declaration whenever possible.
- This helps prevent potential bugs caused by uninitialized variables.
-
Scope Management:
- Declare variables within the appropriate scope to limit their visibility.
- This minimizes conflicts between similarly named variables used elsewhere in the program.
-
Consistent Data Types:
- Ensure consistent data types across declarations to avoid type-related errors.
- Different programming languages have varying rules regarding explicit type declaration.
To emphasize these best practices visually, let’s take a look at the following table showcasing examples of both good and poor variable declaration habits:
Good Practice | Poor Practice |
---|---|
totalPrice | t |
numberOfItems | nI |
customerName | cn |
productDescription | pd |
By adhering to proper variable declaration practices, developers can enhance code clarity and facilitate collaboration among team members while minimizing debugging efforts. Implementing these guidelines not only improves the efficiency of individual programmers but also contributes to the overall quality and maintainability of software projects.
In summary, variable declaration plays a crucial role in computer programming languages. By following best practices such as using descriptive names, proper initialization, managing scope effectively, and ensuring consistent data types, programmers can write cleaner code that is easier to understand and maintain. Incorporating these guidelines into programming workflows fosters efficient development processes and ultimately leads to more robust and reliable software systems.