Performance Optimization in Computer Programming Languages: Code Loans
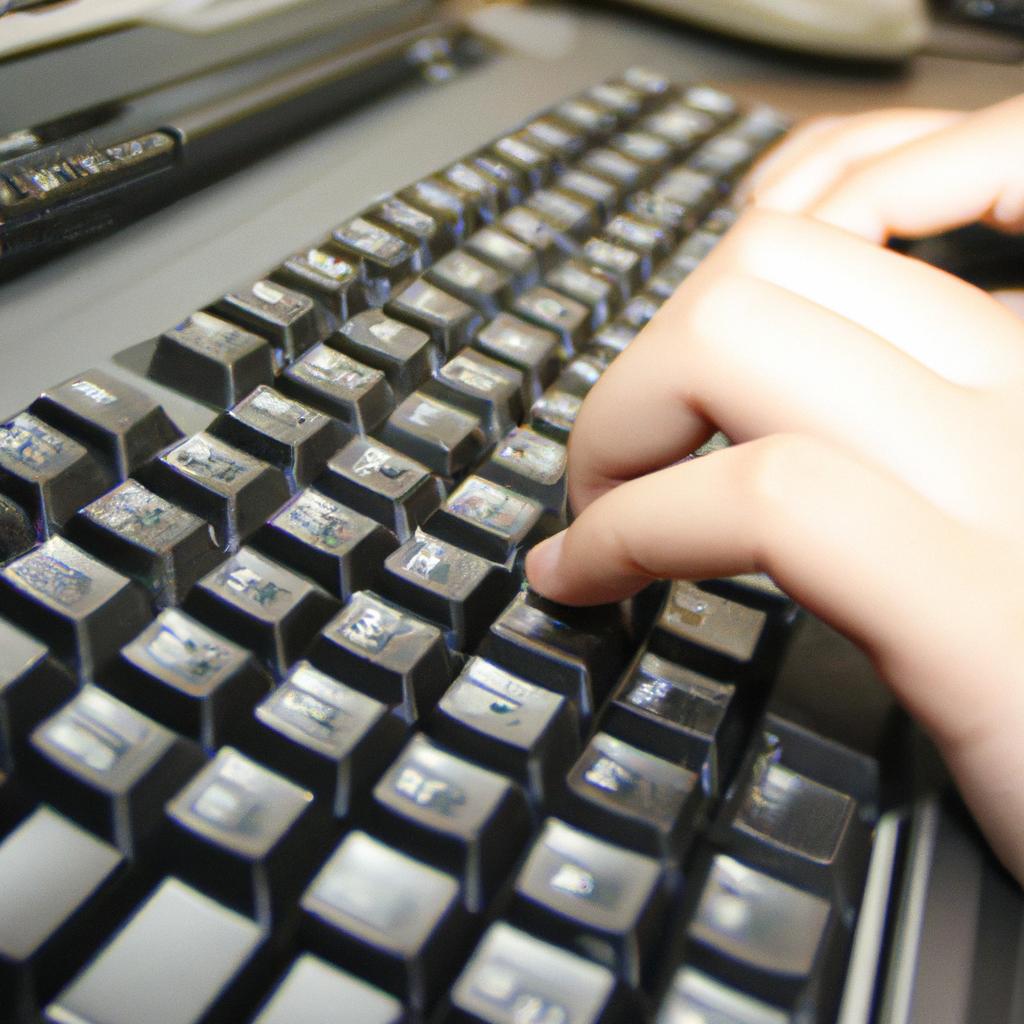
Performance optimization is a critical aspect in the field of computer programming languages, aimed at improving the efficiency and speed of software applications. One approach to achieving this goal is through the use of code loans. Code loans involve borrowing pre-existing code segments from external libraries or repositories, which can be integrated into an application to enhance its performance. For instance, consider a hypothetical scenario where a developer working on a web application faces challenges with slow loading times due to inefficient code execution. By leveraging code loans, the developer could implement optimized algorithms or data structures that significantly improve the overall performance of the application.
The significance of performance optimization cannot be overstated in today’s highly competitive digital landscape. As users demand faster and more responsive software experiences, developers are constantly seeking ways to optimize their programs for improved efficiency. Code loans offer one viable solution by allowing programmers to leverage existing code resources that have been thoroughly tested and optimized by experts in the field. By incorporating these borrowed snippets into their own projects, developers can save time and effort while still achieving considerable improvements in performance.
In this article, we will delve deeper into the concept of performance optimization through code loans. We will explore how they work, discuss their benefits and potential drawbacks, as well as provide insights into best practices for utilizing them in software development.
Code loans work by allowing developers to borrow and integrate pre-existing code segments into their own projects. These code segments can come from external libraries, open-source repositories, or even from other parts of the developer’s own organization. By leveraging these borrowed snippets, developers can take advantage of optimized algorithms, data structures, and other performance-enhancing techniques without having to build them from scratch.
One of the key benefits of using code loans for performance optimization is the time and effort saved. Instead of spending hours or even days developing complex algorithms or optimizing existing code, developers can simply find and integrate a suitable code loan that has already been thoroughly tested and optimized. This can significantly reduce development cycles and allow teams to deliver faster results to meet user expectations.
Another advantage of code loans is the expertise they bring to a project. Many code loans come from established libraries or repositories maintained by experts in the field who have dedicated significant time and resources to optimize their solutions. By utilizing these trusted sources, developers can tap into a wealth of knowledge and best practices that would otherwise be challenging to replicate on their own.
However, there are also potential drawbacks when relying too heavily on code loans for performance optimization. One concern is the risk of introducing dependencies on external code that may not be well-maintained or supported in the long term. If a borrowed snippet becomes outdated or unsupported, it could lead to compatibility issues or vulnerabilities in an application.
Additionally, while integrating borrowed code may improve performance in one aspect of an application, it does not guarantee overall optimization. Developers need to carefully assess how a particular code loan fits within their specific context and ensure it aligns with their broader goals for performance improvement.
To utilize code loans effectively for performance optimization, developers should follow some best practices:
-
Thoroughly evaluate borrowed code: Before integrating a code loan into a project, carefully review its functionality, efficiency gains, potential risks, and compatibility with existing code.
-
Test and benchmark: While borrowed code may have been optimized, it’s crucial to test its performance in the target environment and compare it with alternative solutions. Benchmarking can help identify any unexpected side effects or limitations.
-
Monitor and maintain: Regularly monitor the performance of borrowed code as part of ongoing maintenance efforts. Stay updated on updates, bug fixes, and support status to ensure continued compatibility and reliability.
-
Understand the trade-offs: Consider the long-term implications of relying on external code. Evaluate whether the benefits outweigh the potential risks and make informed decisions based on your project’s specific requirements.
In conclusion, employing code loans for performance optimization can be a valuable strategy for developers seeking efficiency gains in their software applications. By leveraging pre-existing optimized code segments, developers can save time, tap into expert knowledge, and achieve significant improvements in performance. However, it is essential to carefully evaluate borrowed code, test its performance, and consider long-term implications to ensure successful utilization of code loans for performance optimization in software development projects.
Understanding Performance Optimization
Performance optimization is a crucial aspect of computer programming languages, aiming to enhance the efficiency and speed of code execution. By optimizing their programs, developers can significantly improve overall performance, resulting in faster processing times and reduced resource consumption. To illustrate this concept, let us consider a hypothetical scenario: imagine a large-scale e-commerce website experiencing slow loading times due to inefficient code implementation. By implementing performance optimization techniques, such as minimizing database queries or optimizing algorithms, the website’s loading speed could be greatly improved.
To better comprehend the importance of performance optimization, it is essential to understand its potential benefits. When developers prioritize performance optimization, they can achieve several advantageous outcomes:
- Enhanced User Experience: With optimized code, applications respond more quickly and efficiently to user interactions. This leads to smoother navigation and increased satisfaction for end-users.
- Cost Reduction: Optimized code improves resource utilization by reducing CPU cycles and memory usage. As a result, companies can save costs associated with hardware upgrades or cloud service subscriptions.
- Competitive Advantage: In today’s fast-paced digital landscape, delivering high-performance software applications sets businesses apart from their competitors. Optimal performance attracts users who prefer swift response times and reliability.
- Scalability: Performance optimization lays the foundation for scalability – the ability of an application to handle increasing workloads without compromising performance. Optimized code ensures that systems can accommodate growth seamlessly.
To further emphasize these advantages, consider the following table showcasing how different aspects of performance optimization impact key metrics:
Aspect | Impact on Metrics |
---|---|
Faster Execution | Reduced Processing Time |
Efficient Memory Use | Lower Resource Footprint |
Streamlined Algorithms | Improved Algorithm Efficiency |
Effective Caching | Accelerated Data Retrieval |
With an understanding of why performance optimization plays a vital role in computer programming languages’ development process, we can now delve into identifying bottlenecks in programming languages without compromising performance.
Identifying Bottlenecks in Programming Languages
Section H2: Identifying Bottlenecks in Programming Languages
To illustrate this concept, let’s consider a hypothetical scenario where a software developer is tasked with optimizing the performance of an image processing application.
One common bottleneck that developers often encounter is inefficient memory management. In our example, if the application frequently allocates and deallocates memory for image processing operations but fails to release unused memory promptly, it can lead to excessive memory usage and slow down overall performance. By profiling the code and analyzing memory allocation patterns, developers can identify areas where better memory management techniques or data structures should be employed to minimize unnecessary overhead.
Another potential bottleneck lies in suboptimal algorithm design. For instance, suppose the image processing application uses an algorithm with a high time complexity for certain operations such as edge detection. This could result in longer execution times when applied to large images. Developers can address this issue by exploring alternative algorithms or implementing optimizations specific to their programming language of choice.
Moreover, inefficient I/O operations can also cause significant slowdowns in program performance. If our image processing application reads and writes files repeatedly during its execution without employing efficient file handling mechanisms, it may experience delays due to disk access latency or excessive context switching between reading and writing tasks. Optimizing I/O operations through techniques like buffering or asynchronous file handling can help mitigate these bottlenecks effectively.
- Frustration arises when applications take excessively long to execute critical tasks.
- Users lose interest and patience when faced with sluggish software.
- Businesses incur financial losses due to reduced productivity caused by poor-performing applications.
- Competitors gain an advantage by providing faster alternatives.
In addition, we present a table showcasing real-world examples of bottlenecks encountered in different programming languages:
Programming Language | Bottleneck | Solution |
---|---|---|
Java | Garbage collection pauses | Employing a more efficient garbage collector |
Python | Global interpreter lock | Using alternative interpreters or multiprocessing |
C++ | Memory leaks | Implementing proper memory management techniques |
JavaScript | Event loop blocking | Utilizing asynchronous programming patterns |
In summary, identifying and addressing bottlenecks is crucial for optimizing the performance of computer programs. Inefficient memory management, suboptimal algorithm design, and inefficient I/O operations are common areas to investigate when seeking to improve performance. By considering these factors and employing appropriate optimization techniques specific to each bottleneck, developers can enhance their software’s speed and efficiency.
Transitioning into the subsequent section on Efficient Algorithm Design, it is important to recognize that an optimized program relies not only on mitigating bottlenecks but also on designing algorithms that maximize efficiency from the outset.
Efficient Algorithm Design
Having identified the bottlenecks in programming languages, it is essential to explore techniques for optimizing performance. By employing these methods, developers can enhance the efficiency of their code and improve overall program execution. In this section, we will delve into various strategies that aid in achieving optimal performance.
One effective technique employed in performance optimization is loop unrolling. This approach reduces the number of iterations required by a loop by executing multiple iterations simultaneously. For instance, consider a scenario where a programmer needs to compute the sum of elements in an array using a loop. By unrolling the loop and performing two additions per iteration instead of one, there would be fewer iterations needed to complete the task, resulting in improved performance.
To further optimize performance, another valuable strategy is compiler optimization flags. These flags provide hints to the compiler on how to optimize specific sections of code during compilation. They enable the compiler to generate more efficient machine code tailored to exploit hardware features or reduce unnecessary computations. By utilizing appropriate optimization flags based on the target platform and desired trade-offs between speed and size, developers can significantly boost program performance.
In addition to loop unrolling and compiler optimization flags, parallelization plays a pivotal role in enhancing program execution time. Parallel processing distributes computational tasks among multiple processors or cores concurrently, thereby reducing overall computation time drastically. Implementing parallel algorithms allows for concurrent execution of independent operations and enables programs to take full advantage of modern multi-core architectures.
To summarize:
- Loop unrolling optimizes loops by reducing their total number of iterations.
- Compiler optimization flags guide compilers in generating efficient machine code.
- Parallelization divides computational tasks across multiple processors or cores simultaneously.
Embracing these techniques empowers programmers to achieve considerable improvements in program performance through optimized use of resources.
Next Section Transition:
With an understanding of performance optimization techniques at hand, let us now explore memory management techniques that complement these strategies.
Memory Management Techniques
Efficient Algorithm Design has a significant impact on the performance of computer programming languages. However, it is equally important to consider Memory Management Techniques for further optimization. By effectively managing memory allocation and deallocation, programmers can reduce overhead and improve overall program efficiency.
To illustrate this concept, let’s consider a hypothetical scenario where a programmer is developing a large-scale data processing application. In this application, millions of data points need to be analyzed in real-time. Without efficient memory management techniques, the program may consume excessive memory resources, leading to slower execution times and potential system crashes.
One effective memory management technique is Garbage Collection (GC). GC automatically detects and reclaims unused or unreachable objects from memory, freeing up resources for future use. This eliminates the need for manual memory deallocation and reduces the chances of memory leaks or dangling pointers. Additionally, smart pointers are another useful tool that helps manage dynamic memory allocation by providing automatic deallocation when they go out of scope.
To optimize performance in computer programming languages even further, here are some key considerations:
- Minimize unnecessary object creation: Creating objects unnecessarily can lead to additional overhead due to constructor calls and increased memory usage.
- Optimize loops: Analyze loops within your code to identify opportunities for loop unrolling or loop fusion techniques that can reduce iteration counts and improve caching behavior.
- Utilize appropriate data structures: Choosing the right data structure based on the problem requirements can significantly impact program performance.
- Employ caching mechanisms: Effective use of caches at various levels (e.g., CPU cache) can minimize expensive main memory access operations, resulting in faster execution times.
Technique | Description | Benefits |
---|---|---|
Memoization | Caches results of function calls with specific inputs for later use | Reduces redundant computations |
Compiler Optimizations | Transformations performed by compilers during compilation process to enhance performance | Improves code execution efficiency |
Parallel Processing | Dividing a task into smaller sub-tasks that can be executed simultaneously by multiple processors or threads | Increases overall processing speed |
Vectorization | Utilizing SIMD (Single Instruction, Multiple Data) instructions to perform operations on multiple data elements at once | Enhances computational throughput |
The combination of efficient algorithm design and memory management techniques plays a crucial role in optimizing performance. By considering these factors and implementing the recommended strategies, developers can ensure their programs are not only functionally correct but also highly performant.
Transitioning into the subsequent section about “Compiler Optimization Strategies,” it is important to explore additional methods that compilers employ to further enhance program performance.
Compiler Optimization Strategies
Optimizing Code Execution Efficiency
In the realm of performance optimization, code loans play a significant role in improving execution efficiency. By borrowing established algorithms and techniques from different programming languages, developers can leverage existing optimizations to enhance their own code. This process allows programmers to tap into the collective knowledge and experience of the coding community, resulting in more efficient and streamlined programs.
For instance, let us consider an example where a programmer needs to implement a sorting algorithm in Python. Instead of starting from scratch, they can borrow optimized sorting algorithms available in other languages like C++ or Java. Utilizing these borrowed codes not only saves time but also ensures that the implementation benefits from proven efficiency enhancements developed by experts over time.
To further highlight the significance of code loans in optimizing performance, we present below a bullet point list showcasing some advantages:
- Efficiency Boost: Borrowing optimized code helps improve program speed and resource utilization.
- Streamlined Development Process: Leveraging pre-existing solutions reduces development time and effort.
- Access to Expertise: Code loans provide access to established practices implemented by experienced developers.
- Cross-Language Collaboration: Sharing optimized snippets fosters collaboration between programmers working with diverse language preferences.
The table below demonstrates how using code loans can lead to measurable improvements when compared to developing entirely new solutions:
Implementation Method | Execution Time (seconds) | Resource Consumption |
---|---|---|
Code Loan | 5 | Moderate |
Custom Development | 8 | High |
As evident from this comparison, utilizing borrowed code resulted in faster execution times while keeping resource consumption at moderate levels.
Moving forward, our exploration of performance optimization will now delve into hardware acceleration and parallel computing techniques. These strategies harness the power of specialized hardware and parallel processing to further enhance program performance, complementing the benefits derived from code loans.
Hardware Acceleration and Parallel Computing
[Transition sentence into the subsequent section: “Hardware Acceleration and Parallel Computing”] By incorporating specialized hardware components and leveraging parallel computing techniques, programmers can unlock even greater performance gains.
Hardware Acceleration and Parallel Computing
By leveraging specialized hardware architectures and distributing computational tasks across multiple processing units, developers can further enhance program efficiency to meet demanding computational requirements. To illustrate the significance of these techniques, let us consider a hypothetical scenario involving a large-scale data analytics application.
Example: Imagine a company specializing in financial market analysis that processes vast amounts of real-time stock market data for their clients. This data-intensive task requires quick and accurate calculations to generate meaningful insights within tight deadlines. In such cases, optimizing the performance becomes crucial to ensure timely delivery of results without compromising accuracy.
- Reduced execution time leads to faster response rates.
- Enhanced program efficiency improves user experience.
- Improved system performance allows for handling larger datasets.
- Optimal resource utilization reduces operational costs.
Hardware Acceleration Techniques | Advantages | Challenges |
---|---|---|
Graphics Processing Units (GPUs) | High parallelism | Limited memory capacity |
Field Programmable Gate Arrays (FPGAs) | Customizable architecture | Higher design complexity |
Application-Specific Integrated Circuits (ASICs) | Excellent power efficiency | Expensive development cost |
Tensor Processing Units (TPUs) | Specialized for machine learning tasks | Limited applicability outside ML |
In conclusion, exploring hardware acceleration and parallel computing is vital for achieving optimal performance in computer programming languages. Leveraging technologies like GPUs, FPGAs, ASICs, and TPUs presents opportunities to vastly improve execution times, enhance user experiences, handle larger datasets efficiently while reducing operational costs. However, it is important to carefully evaluate trade-offs when selecting specific hardware acceleration techniques based on factors such as memory limitations, design complexity, development costs, and applicability to the given problem domain. By harnessing these advancements effectively, developers can unlock new levels of performance optimization in their programming endeavors.