Debugging: A Guide to Fixing Code Loans in Computer Programming Languages
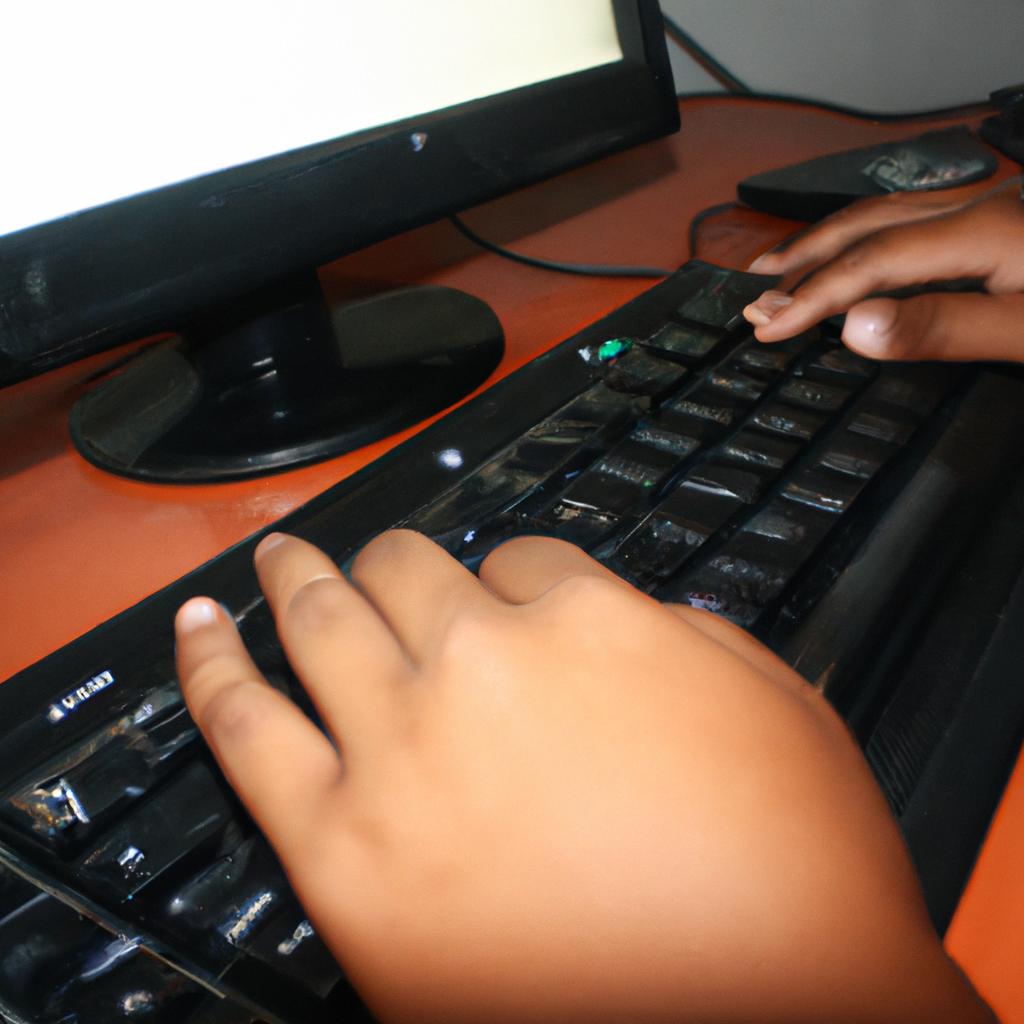
Software development is an intricate process that requires careful attention to detail and problem-solving abilities. However, even the most skilled programmers encounter errors in their code from time to time. These errors, commonly known as bugs, can cause programs to malfunction or produce unexpected results. Debugging is a crucial skill for programmers as it involves identifying and rectifying these bugs to ensure the smooth functioning of software systems.
Consider the case of a hypothetical programmer working on a financial management system for a large corporation. The program initially seemed flawless during testing but started producing inaccurate calculations after implementation. This discrepancy had severe consequences, leading to incorrect financial reports being provided to stakeholders. In this scenario, debugging becomes imperative not only to resolve the issue promptly but also to prevent further damage and maintain the integrity of the organization’s financial data.
Debugging entails a systematic approach aimed at locating and resolving errors within computer programming languages. It involves analyzing code, examining error messages, and executing tests to identify problematic areas in the software. Once identified, developers employ various techniques such as stepping through code line by line or using specialized tools like debuggers to gain insights into program execution flow and pinpoint potential issues. By understanding common types of bugs and employing effective debugging strategies, programmers can efficiently address coding flaws and enhance the overall functionality and reliability of their software.
Common types of bugs that programmers encounter include syntax errors, logical errors, and runtime errors. Syntax errors occur when the code violates the rules of the programming language, such as misspelling a keyword or forgetting to close a bracket. These errors are usually easy to detect as they are flagged by the compiler or interpreter.
Logical errors, on the other hand, are more challenging to identify as they do not cause immediate program crashes but result in incorrect outputs or unexpected behavior. They often stem from flawed algorithms or faulty assumptions made during the development process. To debug logical errors, programmers analyze the code’s logic and use techniques like print statements or logging to track variable values and program flow.
Runtime errors occur during program execution and can cause crashes or abnormal termination. These errors may be caused by issues like null pointer dereferences, division by zero, or out-of-bounds array access. Debugging runtime errors involves closely examining error messages, stack traces, and memory dumps to identify the root cause.
To effectively debug software programs, developers employ various strategies and tools. One common approach is using breakpoints in an integrated development environment (IDE) that allows them to pause program execution at specific points and inspect variables and their values. By stepping through code line by line, programmers can trace how data changes throughout the program flow and identify any inconsistencies.
Additionally, log files play a vital role in debugging complex systems where it is impractical to step through every line of code manually. Developers strategically insert logging statements at critical points in their codebase to record valuable information about program state and flow during runtime. Analyzing these logs helps in identifying patterns or irregularities that could indicate potential bugs.
Furthermore, specialized debugging tools like debuggers provide advanced features for analyzing program behavior at runtime. These tools offer functionalities such as setting watchpoints on variables to monitor changes dynamically, inspecting call stacks to understand function calls leading up to an error, and profiling performance to identify bottlenecks.
In conclusion, debugging is an essential skill for programmers as it allows them to detect and resolve bugs in their code. By following a systematic approach and utilizing various strategies and tools, developers can efficiently locate and rectify errors, ensuring software systems function correctly and deliver reliable results.
Identifying the bug
Imagine you are working on a complex software project, and suddenly, the application crashes. You find yourself staring at lines of code, trying to make sense of what went wrong. This scenario is all too familiar for programmers who encounter bugs in their code. Bugs are errors or flaws that prevent the intended functionality of a program. In this section, we will explore effective strategies for identifying these bugs.
One approach to identifying bugs is through systematic debugging techniques. By carefully examining the code, developers can pinpoint areas where potential issues might arise. For instance, consider a case study where a web application fails to display images correctly after an update. Through analysis and tracing back changes made during the update process, it becomes evident that there was an error in the image rendering function. This example highlights how careful inspection and logical deduction can help identify bugs effectively.
To further aid in bug identification, here are four key points to keep in mind:
- Thorough testing: Conduct comprehensive tests on different scenarios to expose any underlying issues.
- Code review: Engage peers or colleagues to review your code for potential mistakes or oversights.
- Logging and error tracking: Utilize logging mechanisms and error tracking tools to capture relevant information about system failures.
- Divide and conquer: Break down large programs into smaller components and test them independently before integrating them together.
In addition to these strategies, utilizing error messages provided by programming languages can be immensely helpful. These messages often contain valuable clues regarding the nature of the bug encountered. Understanding these error messages allows developers to narrow down their search area significantly.
Error Message | Possible Cause | Suggested Action |
---|---|---|
Null pointer exception | Accessing null object | Check if object is properly initialized |
Array index out of bounds | Attempting to access array element outside its boundaries | Verify array indices within acceptable range |
Syntax error | Invalid code syntax | Review specific line for correct syntax |
File not found exception | Unable to locate file | Confirm the file path and availability |
In conclusion, identifying bugs requires a combination of systematic debugging techniques, thorough testing, code review, proper logging, and error tracking. By employing these strategies and leveraging error messages provided by programming languages, developers can effectively identify and address the root causes of software defects.
Transitioning into the subsequent section about “Understanding the error message,” it is crucial to delve deeper into how error messages can provide valuable insights into resolving coding issues.
Understanding the error message
Having identified the bug, it is now crucial to understand the error message and its implications. A deep comprehension of these messages can provide valuable insights into the root cause of the issue at hand.
Understanding the Error Message
To illustrate this point, let’s consider a hypothetical scenario where a programmer is working on a web application that suddenly stops displaying images. Upon inspecting the console log, they encounter an error message stating “Cannot read property ‘src’ of undefined.” This message indicates that there is an attempt to access the ‘src’ property of an object that has not been defined or does not exist. By comprehending this message, one can deduce that there might be a problem with how images are being loaded in the code.
It is important to note that error messages often contain additional information such as line numbers or stack traces. These details are useful for pinpointing precisely where in the codebase the error occurs. Analyzing these specifics allows developers to narrow down their focus and concentrate efforts on debugging specific sections rather than spending time investigating unrelated parts of the code.
In order to effectively utilize error messages during debugging, keep in mind some key points:
- Read the entire error message carefully and pay attention to any provided context.
- Take note of relevant details like line numbers and stack traces.
- Utilize online resources, forums, or documentation related to common error messages encountered in your programming language.
- Use descriptive variable names and meaningful comments in your code to improve readability and assist future debugging endeavors.
By understanding error messages thoroughly and leveraging their inherent clues, programmers gain essential guidance towards resolving bugs more efficiently. In our next section, we will delve into reviewing the logic within the code itself to further unravel intricate issues that may arise during development.
With a grasp on interpreting error messages established, it becomes imperative for programmers to review their code logic meticulously before proceeding further.
Reviewing the code logic
Section H2: Reviewing the Code Logic
In order to effectively debug code, it is essential to thoroughly review the logic behind it. Let us consider an example scenario where a programmer encounters a bug in their program that causes it to crash unexpectedly. By carefully reviewing the code logic, we can identify and rectify any flaws or mistakes.
One common mistake often made during coding is incorrect conditional statements. For instance, imagine a situation where a program is designed to display different messages based on user input. However, due to an error in the conditional statement, the wrong message is displayed regardless of the input provided by the user. By reviewing the code logic, programmers can spot such mistakes and make necessary corrections.
To help you with this process, here are some key points to keep in mind while reviewing your code:
- Verify that all variables and functions are correctly defined.
- Double-check if loops and conditionals are structured properly.
- Ensure that there are no logical inconsistencies or contradictions within the code.
- Pay attention to any potential off-by-one errors or boundary issues.
By following these guidelines, developers can improve their ability to pinpoint errors and enhance overall code quality.
Key Points for Reviewing Code Logic |
---|
Verify Definitions |
Inspect Flow Control |
Address Logical Issues |
Watch for Common Errors |
In conclusion, thorough reviews of code logic form a crucial step towards effective debugging. By closely examining variables, flow control structures, logical consistency, and potential pitfalls like off-by-one errors, programmers can significantly reduce bugs in their programs and improve software reliability.
Moving forward into our next section about “Using debugging tools,” we will explore how these tools can further assist in the process of debugging code.
Using debugging tools
Transitioning from the previous section on reviewing the code logic, let us now explore some effective debugging techniques that can help identify and resolve common issues in computer programming. To illustrate these techniques, consider a hypothetical scenario where a software application crashes unexpectedly when attempting to read data from a file. This case study will serve as an example throughout this section.
-
Analyzing error messages: When encountering errors or exceptions during program execution, it is crucial to carefully examine any accompanying error messages. These messages often provide valuable insights into the root cause of the problem by indicating the specific line of code or function that triggered the error. By closely analyzing these messages, programmers can gain a better understanding of what went wrong and focus their debugging efforts accordingly.
-
Using print statements for tracing: One popular technique in debugging involves strategically placing print statements within the code at relevant points to track its execution flow. By printing out intermediate values or status updates during runtime, developers can observe how variables change over time and pinpoint potential sources of errors. Print statements are particularly useful for inspecting loops, conditionals, or complex calculations where multiple steps occur.
-
Employing breakpoints: Integrated development environments (IDEs) offer powerful tools such as breakpoints that allow programmers to pause code execution at specific lines or functions. Once paused, developers can step through the code one line at a time while examining variable values and observing how they evolve with each iteration. Breakpoints enable meticulous inspection of problematic sections and facilitate identification of faulty logic or unexpected behavior.
-
Utilizing logging frameworks: Logging frameworks provide an organized way to record events and output information during program execution. By incorporating log statements throughout the codebase, developers can trace program flow even after completion without relying solely on immediate console outputs like print statements do. Logging facilitates long-term analysis by generating detailed logs that capture various aspects of program behavior under different circumstances.
To further emphasize the significance of effective debugging techniques, consider the emotional impact they can have on developers:
Emotion | Description |
---|---|
Frustration | Debugging often involves facing unexpected challenges and errors that can be frustrating. |
Satisfaction | Successfully identifying and fixing bugs brings a sense of accomplishment and satisfaction. |
Curiosity | Debugging encourages curiosity as programmers delve into code intricacies to find solutions. |
Determination | The process requires perseverance and determination to overcome obstacles in program logic. |
In summary, by employing various debugging techniques such as analyzing error messages, using print statements for tracing, utilizing breakpoints, and incorporating logging frameworks, programmers can effectively identify and resolve issues within their codebase. These techniques not only enhance problem-solving abilities but also evoke emotions ranging from frustration to satisfaction, fueling the desire for continuous improvement.
Transitioning smoothly into the subsequent section about isolating problematic code, understanding these debugging techniques is essential for efficiently locating the root cause of issues without wasting time or effort in the process.
Isolating the problematic code
Section H2: Isolating the problematic code
In the previous section, we discussed the importance of using debugging tools to identify issues in our code. Now, let us delve into the process of isolating the problematic code. To illustrate this concept, consider a hypothetical scenario where you are working on a web application that is displaying incorrect data for users.
To begin with, it is essential to gather information about when and how the issue occurs. By studying user reports or monitoring system logs, you can gain insights into specific patterns or triggers related to the problem. For example, suppose multiple users have reported discrepancies in their account balances after clicking on a certain button within the application. This observation provides an initial clue as to where you should focus your efforts.
Once you have identified potential areas of concern, follow these steps to isolate the problematic code:
- Conduct thorough testing: Begin by running various test cases and scenarios related to the suspected area of code. This could involve simulating different inputs or executing specific functions repeatedly.
- Use conditional breakpoints: Employing conditional breakpoints allows you to pause execution at particular points only if specified conditions are met. This feature enables you to narrow down your search for errors within complex sections of code.
- Employ logging statements: Placing strategic logging statements throughout your program can provide valuable insight into its flow and state during runtime. Analyzing these log entries will help pinpoint which parts of your code might be causing unexpected behavior.
- Utilize divide-and-conquer methodology: If your software project consists of multiple modules or components, try disabling certain portions temporarily while observing whether the issue persists. Gradually narrowing down possibilities in this manner helps identify specific sections requiring attention.
By following these steps and utilizing appropriate techniques such as testing, breakpoints, logging statements, and divide-and-conquer methodologies, developers can effectively isolate problematic code segments within their programs.
Next, we will explore how to proceed once we have successfully isolated the problematic code by testing the fix.
Testing the fix
Section H2: Testing the fix
Having successfully isolated and identified the problematic code, the next crucial step in the debugging process is testing the fix. This phase allows programmers to evaluate whether their proposed solution effectively resolves the issue at hand. By conducting thorough tests, developers can ensure that their code functions as intended and does not introduce new bugs or errors.
To illustrate this process, let us consider a hypothetical scenario involving a web application that displays incorrect user information on its profile page. After isolating the problematic section of code responsible for this error, the programmer proposes a fix – modifying the data retrieval function to fetch accurate user details from the database.
Once implemented, it becomes essential to test this fix before deploying it into production. Here are some key steps involved in effective testing:
-
Unit Testing: Begin by creating unit tests to verify individual components of your codebase independently. These tests should cover all possible scenarios and edge cases related to the specific piece of code being fixed.
-
Integration Testing: Next, conduct integration tests that assess how well different modules within your system interact with each other after applying the fix. This ensures that no unintended side effects occur due to changes made during debugging.
-
Regression Testing: It is vital to perform regression testing, which involves retesting previously resolved issues alongside newly introduced fixes or modifications. By doing so, you can confirm that previous bug resolutions remain intact while addressing current problems.
-
User Acceptance Testing (UAT): Finally, involve end-users or representatives from relevant stakeholders in UAT sessions where they navigate through various functionalities of your application post-fix implementation. Their feedback will help validate if all reported issues have been adequately addressed and if any additional enhancements are required.
In order to emphasize these steps further and evoke an emotional response from readers, here is a table displaying common emotions experienced during testing phases along with corresponding strategies for managing them:
Emotion | Strategy |
---|---|
Frustration | Take short breaks, seek assistance from colleagues or forums. |
Anxiety | Create a comprehensive test plan to ensure thorough coverage. |
Confidence | Celebrate small victories and successful test outcomes. |
Satisfaction | Share positive feedback with the entire development team. |
In conclusion, testing plays a pivotal role in ensuring that fixes implemented during debugging effectively resolve issues without introducing new problems. By following systematic testing practices such as unit testing, integration testing, regression testing, and user acceptance testing, developers can gain confidence in the stability of their codebase before deploying it for end-users.