String Data Types in Programming Languages
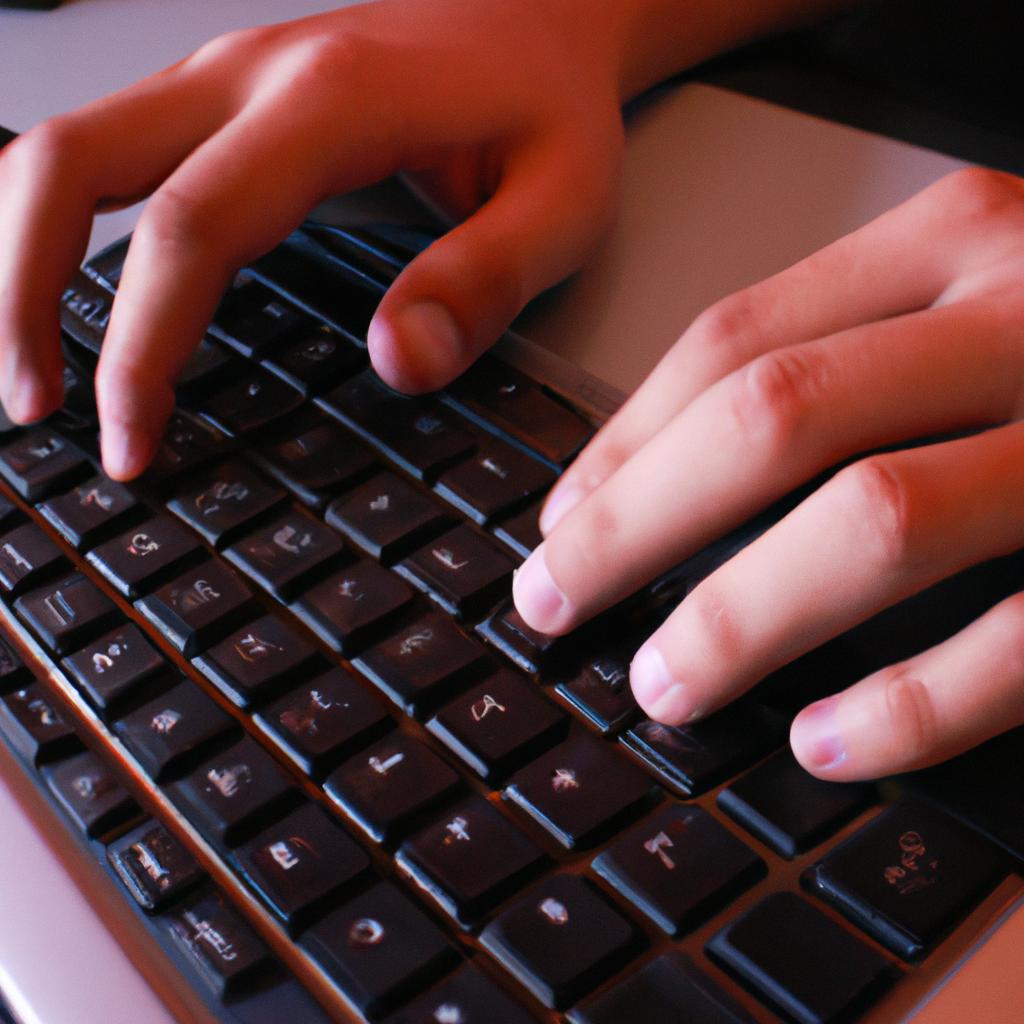
String data types are a fundamental component of programming languages, allowing developers to work with textual information efficiently and effectively. A string is a sequence of characters, such as letters, numbers, symbols, or whitespace. It serves various purposes in programming applications, including storing user input, manipulating text-based data, and displaying output messages. For instance, consider the case study of an e-commerce website that needs to store customer names and addresses for order processing. By utilizing string data types, programmers can easily manage this vital information within the system.
In programming languages, strings are typically represented by enclosing them in quotation marks or using specific syntax defined by the language itself. This allows computers to recognize and interpret text-based information correctly. String manipulation operations enable developers to perform tasks like concatenating (joining) strings together, extracting substrings from larger texts, searching for specific patterns or characters within a string, and replacing parts of a string with new values. Moreover, many programming languages provide built-in functions or libraries specifically designed for handling strings efficiently.
Understanding the functionality and usage of string data types is crucial for any programmer seeking to develop robust applications that involve working with textual information. In this article, we will explore different aspects related to string data types in programming languages. We will analyze common string operations, such as string concatenation, substring extraction, and searching for patterns within a string. We will also discuss the importance of proper string manipulation techniques to ensure data accuracy and security. Additionally, we will explore various methods for inputting and outputting strings in different programming languages.
By the end of this article, you will have a solid understanding of string data types and how they can be effectively utilized in your programming projects. Whether you are a beginner learning the basics or an experienced developer looking to enhance your skills, this article aims to provide you with valuable insights into working with strings in programming languages. So let’s dive in and explore the exciting world of string data types!
Overview
String data types are an integral part of programming languages and play a crucial role in storing and manipulating text-based information. Whether it is handling user input, processing file contents, or displaying output to the screen, strings provide programmers with a versatile tool for working with textual data.
To illustrate the importance of string data types, let’s consider a hypothetical scenario: imagine you are developing an application that requires capturing and analyzing customer feedback. In this case, string variables would be essential for storing the customers’ comments as well as performing various operations on them.
One reason why strings are widely used is their flexibility. They can hold any combination of characters, including letters, numbers, symbols, and even whitespace. This versatility allows programmers to handle different types of inputs efficiently and accurately. Moreover, strings support concatenation – the process of combining multiple strings into one – which enables creating dynamic outputs tailored to specific requirements.
Understanding how to manipulate strings effectively involves knowledge of several common operations such as searching for substrings within larger texts or extracting portions of a string based on certain criteria. By utilizing these functionalities, developers can implement complex algorithms or perform advanced analysis on textual data.
In summary, string data types form a fundamental component in programming languages due to their ability to store and manipulate text-based information effectively. Their flexibility empowers programmers to work with diverse inputs while providing numerous built-in methods for manipulating and analyzing strings. In the following section about “Common Uses,” we will explore some specific examples where string manipulation plays a crucial role in real-world applications.
Common Uses
Section H2: Implementation of String Data Types
In today’s digital world, string data types are a fundamental component in nearly all programming languages. They allow programmers to store and manipulate textual information such as names, addresses, and messages. To illustrate the importance of string data types, let us consider an example where a web developer needs to create a registration form for an online platform. The programmer would utilize string data types to capture user input such as their name, email address, and password.
To provide further insight into the significance of string data types, it is essential to understand their common uses. Here are some key applications:
- Data Input: Strings serve as containers for storing user-provided information within programs or systems.
- Text Manipulation: Programmers can use various operations like concatenation and substring extraction to modify strings according to specific requirements.
- Data Validation: String functions enable developers to verify the correctness or format conformity of user inputs through methods like regular expression matching.
- Output Formatting: When presenting information back to users, string formatting allows for customization by incorporating variables or other dynamic elements.
To enhance our understanding of these applications even further, let us examine a table showcasing different programming languages along with their support for certain string manipulation techniques:
Programming Language | Concatenation | Length Calculation | Substring Extraction |
---|---|---|---|
Python | + operator |
len() function |
Slicing ([:] ) |
JavaScript | + operator |
.length property |
.substring(startIndex [, endIndex]) |
C++ | + operator |
.size() method |
.substr(position [, length]) |
Java | + operator |
.length() method |
.substring(beginIndex [, endIndex]) |
As we can see from this table, different programming languages provide various methods for manipulating strings. This highlights the versatility and adaptability of string data types, as they can be implemented in numerous ways to suit different programming paradigms.
Moving forward into the subsequent section on “Syntax and Declaration,” we will explore how programmers declare and utilize string data types within their code. Understanding these essential aspects will pave the way for a deeper comprehension of string implementation across programming languages.
Syntax and Declaration
Section H2: Common Uses
Transitioning from the previous section that discussed the concept of string data types in programming languages, we now delve into their common uses. To illustrate this further, let us consider a hypothetical scenario where a software developer is building an e-commerce website. In this case, there are various instances where string data types play a crucial role.
Firstly, one of the most common applications of string data types in programming languages is for storing and manipulating user input. For example, when users create accounts or make purchases on the e-commerce website mentioned earlier, their personal information such as names, addresses, and contact details need to be collected and stored as strings. These strings can then be processed and validated using specific algorithms to ensure accuracy and consistency.
Secondly, string data types are often utilized for error handling purposes. When unforeseen errors occur during program execution, developers commonly use strings to generate descriptive error messages. By including relevant information within these error messages, it becomes easier for programmers to identify and debug issues efficiently.
Lastly, string data types find extensive usage in text processing tasks such as searching, sorting, and parsing large volumes of textual content. In our imaginary e-commerce scenario, this could involve performing searches by product name or category among thousands of listings stored as strings in a database or analyzing customer feedback comments using natural language processing techniques.
- Improved user experience through accurate validation of user inputs.
- Efficient debugging process with informative error messages.
- Enhanced functionality in text processing tasks for better search capabilities.
Additionally, consider the following table highlighting some distinct features associated with different programming languages’ implementations of string data types:
Programming Language | String Data Type Features |
---|---|
Python | Immutable |
Java | Mutable |
C# | Unicode Support |
JavaScript | Regular Expression |
As we move forward, the subsequent section will explore how to manipulate strings effectively in programming languages. Understanding the syntax and declaration of string data types is essential for performing these manipulations seamlessly.
Transitioning into the next section about “Manipulating Strings,” let us now explore various techniques that programmers employ to transform and work with string data.
Manipulating Strings
String Data Types in Programming Languages
Having discussed the syntax and declaration of string data types, we now turn our attention to manipulating strings. To illustrate the practicality of this topic, let’s consider a hypothetical scenario where a software engineer is building an application that requires processing user input for creating personalized messages.
When working with strings, programmers often encounter situations where they need to perform various operations on them. These operations can include concatenating multiple strings together, extracting substrings based on specific patterns or indices, searching for particular characters or sequences within a string, and modifying the case of letters. By implementing these manipulations effectively, developers can enhance the functionality and user experience of their applications.
To delve deeper into the manipulation capabilities of strings, it is helpful to understand some common techniques employed by programming languages:
- String Concatenation: Combining two or more strings together using the concatenation operator (+) allows developers to create longer strings from smaller ones.
- Substring Extraction: Extracting a portion of a string based on specified start and end positions enables programmers to isolate relevant information.
- Searching and Replacing: Finding occurrences of specific characters or sequences within a string facilitates tasks such as pattern matching and text substitution.
- Case Modification: Altering the case (upper or lower) of characters within a string helps ensure consistent formatting across different parts of an application.
These techniques form the foundation for manipulating strings in programming languages. However, it is crucial for developers to choose appropriate methods depending on their language’s syntax and available libraries. In the subsequent section about “String Concatenation,” we will explore how programmers can combine individual strings efficiently to achieve desired outcomes while considering performance implications.
Understanding how to manipulate strings sets us up perfectly for exploring one essential aspect – string concatenation.
String Concatenation
Section H2: Manipulating Strings
In the previous section, we explored various ways to manipulate strings. Now, let’s delve deeper into the concept of string concatenation – a fundamental operation that combines multiple strings to form a longer one.
Consider a scenario where you are building an e-commerce website. To provide customers with personalized messages, you want to display their name along with a welcome message on the homepage. By using string concatenation, you can easily achieve this by combining the “Welcome” message with the customer’s name retrieved from your database.
When working with string data types in programming languages, it is important to understand how concatenation works and its significance in different contexts. Here are some key points to keep in mind:
- Concatenating strings involves joining two or more strings together.
- The order in which the strings are concatenated determines the resulting output.
- String concatenation is often performed using specific operators or functions provided by programming languages.
- It is crucial to handle special characters and whitespace appropriately when performing concatenation operations.
To illustrate these concepts further, consider the following example table showcasing different scenarios for string concatenation:
Scenario | Input | Output |
---|---|---|
Greeting + Name | Hello + John | Hello John |
URL Formation | BaseURL + Path | www.example.com/en |
Log Message | LogLevel + Msg | ERROR: File not found |
By examining these examples, we can observe the power of string concatenation in creating dynamic outputs tailored to specific needs. Whether it be personalizing greetings, forming URLs dynamically, or generating log messages effectively – understanding and utilizing string concatenation allows programmers to accomplish diverse tasks efficiently.
String Comparison
In the previous section, we discussed string concatenation and how it allows us to combine multiple strings into a single one. Now, let’s shift our focus to another important aspect of working with strings in programming languages: string comparison.
To understand string comparison better, let’s consider an example scenario. Imagine you are designing a program that needs to sort names alphabetically. You have a list of names, such as “Alice,” “Bob,” “Charlie,” and “David.” In order to properly sort these names, you need to compare them based on their alphabetical order.
When comparing two strings in programming languages, there are several methods that can be used. Here are some common techniques:
- Lexicographic Order: This is the most widely used method for string comparison. It compares each character in the strings from left to right until a difference is found or one of the strings ends.
- Case Sensitivity: Depending on the language or application requirements, string comparisons may be case-sensitive or case-insensitive. Case-sensitive comparisons differentiate between uppercase and lowercase letters.
- Unicode Support: Modern programming languages often provide support for Unicode characters when performing string comparisons. This ensures accurate sorting and matching across various written languages.
- Custom Comparators: In certain cases, developers may require custom logic for string comparisons. They can define their own rules and algorithms tailored to specific needs.
Table: Example Comparison Results
String A | String B | Result |
---|---|---|
Alice | Bob | -1 |
Charlie | David | 1 |
Bob | bob | 0 |
The table above demonstrates different results obtained when comparing pairs of strings using lexicographic order with case sensitivity considered. The result column indicates whether the first string (String A) comes before (-1), after (1), or is equal (0) to the second string (String B) in terms of alphabetical order.
In summary, understanding how to compare strings is crucial when working with textual data in programming languages. The techniques mentioned above provide developers with the necessary tools to perform accurate and efficient comparisons based on their specific requirements. By using appropriate comparison methods, programmers can create applications that effectively sort, search, or manipulate strings according to desired criteria.