For Loop: Control Flow in Computer Programming Languages
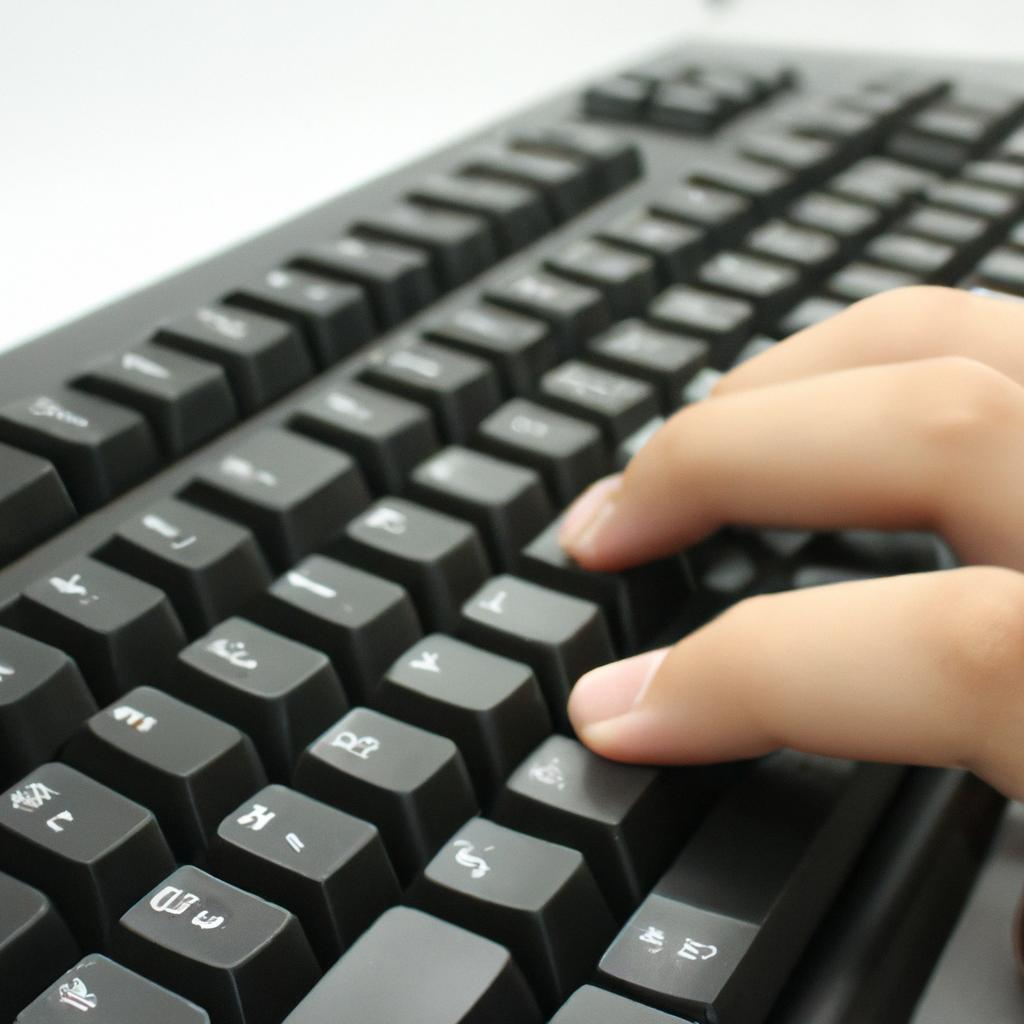
The for loop is a fundamental concept in computer programming languages that allows the repetition of a set of instructions. It provides control flow through iterative execution, making it an essential tool for automating repetitive tasks and processing large amounts of data. For example, imagine a scenario where a company needs to calculate the average sales per month over the past year. By using a for loop, programmers can iterate through each month’s sales data and accumulate the total sales, ultimately dividing it by twelve to obtain the desired result.
In computer programming, control flow refers to how statements are executed within a program. The for loop is one such construct that enables programmers to repeat a block of code multiple times with slight variations in its parameters. Its syntax consists of three parts: initialization, termination condition, and incrementation or decrementation step. This powerful mechanism allows efficient handling of complex tasks that require repeated computations or operations on collections of elements.
By understanding how to utilize the for loop effectively, programmers gain greater flexibility in their code implementation while optimizing efficiency. In this article, we will explore the intricacies of the for loop across various programming languages and discuss different use cases where it proves particularly useful. Additionally, we will delve into best practices and potential pitfalls associated with employing this control flow structure to ensure clean and error-free code.
When implementing a for loop, it is crucial to consider the initialization step. This part of the loop sets an initial value for the index variable that controls the iteration. It is essential to initialize this variable correctly to avoid unexpected behavior or infinite loops.
The termination condition determines when the loop will stop executing. It typically involves a comparison between the index variable and a specific value. The loop will continue as long as this condition evaluates to true. Care should be taken to ensure that the termination condition is appropriate for the task at hand, as an incorrect condition can lead to incorrect results or endless looping.
The incrementation or decrementation step updates the index variable after each iteration. This step allows programmers to control how the index changes between iterations, such as incrementing by one or decrementing by a specific value. It is vital to choose an appropriate step size that ensures the loop progresses towards its termination condition.
Programmers must also be aware of potential pitfalls when using for loops. One common mistake is modifying the index variable within the loop body, which can result in unexpected behavior or infinite looping. Additionally, care must be taken when working with collections or arrays inside a for loop to avoid accessing elements beyond their bounds.
Different programming languages may have slightly different syntax and features associated with for loops, but they generally follow similar principles. Some languages offer additional functionalities like iterating over ranges of values, stepping through collections automatically, or even using multiple variables in a single for loop construct.
In conclusion, understanding and effectively utilizing for loops are essential skills in computer programming. By mastering this control flow structure and following best practices, programmers can automate repetitive tasks, process large amounts of data efficiently, and create clean and robust code implementations
Definition of a For Loop
Definition of a For Loop
A for loop is a fundamental control structure in computer programming languages that allows the repeated execution of a block of code based on certain conditions. It provides an efficient and structured way to iterate through a sequence or collection, making it an indispensable tool for handling repetitive tasks in various programming scenarios.
To illustrate its functionality, consider a hypothetical scenario where we have a list of numbers and want to find the sum of all even values within the list. By using a for loop, we can easily achieve this goal by iterating over each element in the list, checking if it is divisible by 2, and accumulating the even values into our final result.
When discussing the advantages and importance of using for loops, several key points come to light:
- Efficiency: For loops provide an optimized method for executing repetitive code by eliminating redundant lines and reducing complexity.
- Flexibility: They enable developers to define specific iterations based on their requirements, such as incrementing or decrementing variables at custom intervals.
- Productivity: With their ability to automate repetitive tasks efficiently, for loops enhance overall productivity by saving time and effort.
- Readability: The structured nature of for loops enhances code readability, allowing other programmers to understand the logic more easily.
This table demonstrates how different programming languages utilize syntax variations while implementing for loops:
Language | Syntax |
---|---|
C | for (initialization; condition; iteration) |
Python | for item in iterable: |
Java | for (type variable : array/collection) |
JavaScript | for (variable; condition; expression) |
Moving forward, let us delve deeper into understanding the syntax of a for loop without any further delay.
Syntax of a For Loop
Building upon the definition of a for loop, let us now explore its syntax and understand how it controls the flow of execution in computer programming languages.
Syntax of a For Loop:
A for loop typically consists of three essential components: initialization, condition, and iteration. These elements work together to determine the number of times a set of instructions within the loop body will be executed. To illustrate this concept, consider the following hypothetical example:
for i in range(1, 6):
print("Iteration", i)
In this case, the for loop is initialized with i
starting at 1 and ending at 5 (inclusive). The condition i in range(1, 6)
evaluates to True as long as i
is within the specified range. After each iteration, the value of i
is incremented automatically by one due to the built-in mechanism provided by the language. As a result, “Iteration” followed by the current value of i
will be printed five times.
- A for loop provides an efficient way to repeat a block of code without duplicating it multiple times.
- It allows programmers to iterate over collections such as lists or arrays and perform operations on each element individually.
- By utilizing counters or iterators, complex patterns can be created that execute specific actions based on certain conditions.
- The flexibility offered by for loops makes them indispensable in automating repetitive tasks and implementing algorithms efficiently.
Advantages | Disadvantages | Use Cases |
---|---|---|
Simplifies repetitive tasks | Limited control over iteration | Processing array elements |
Enhances code readability | May cause infinite loops | Iterating through file lines |
Reduces code duplication | Requires careful management | Generating sequences |
Final Paragraph:
Embracing simplicity and efficiency, for loops have become a fundamental construct in programming languages. Their ability to control the flow of execution and repeat code based on specific conditions makes them valuable tools for developers.
[Next Section: Initialization in a For Loop]
Initialization in a For Loop
Moving on from the syntax of a for loop, we now delve into the concept of initialization. Understanding how to properly initialize a for loop is crucial in ensuring its effectiveness and efficiency.
To illustrate the importance of initialization, let’s consider an example scenario where we have a program that needs to calculate the average temperature over a certain period of time. We are given an array containing daily temperature readings for ten consecutive days. The goal is to find the sum of all temperatures and then divide it by ten to obtain the average temperature.
When initializing a for loop, there are several key aspects to consider:
-
Variable declaration: Before entering the for loop, variables used within it should be declared appropriately. In our temperature calculation example, we would need two variables; one to hold the sum of temperatures (let’s call it ‘sumTemp’) and another for iterating through each element in the array (‘i’ for index).
-
Assignment of initial values: Once declared, these variables must be assigned their initial values before executing the loop. In our case, ‘sumTemp’ should be set initially as zero since no temperatures have been added yet, while ‘i’ would typically start at zero or one depending on programming language conventions.
-
Iteration condition: A logical expression determining when the iteration should stop must be defined. In this context, we want our loop to iterate until ‘i’ reaches nine (representing ten elements in total). If ‘i’ exceeds this value, the loop will terminate.
-
Incremental statement: Finally, an incremental statement specifies how much ‘i’ should change with each iteration. Typically, it increments by one using either ‘+=’ or ‘++’. This ensures that every element in the array is accessed sequentially during each iteration.
By understanding these principles and applying them correctly when initializing a for loop, programmers can effectively control flow within their programs.
Now that we have established how initialization sets the stage for a smoothly running for loop, let us delve into the condition that governs its execution.
Condition in a For Loop
Building upon the concept of initialization in a for loop, we now delve into understanding the condition that plays a crucial role in controlling the flow of execution. A solid grasp on this aspect enables programmers to efficiently navigate through their code and execute specific actions based on predetermined conditions.
Condition in a For Loop:
To illustrate how conditions work within a for loop, let’s consider an example scenario involving a program designed to calculate the sum of all even numbers between 1 and 10. In this case, the condition would be set as “i <= 10,” where ‘i’ represents the variable used to iterate through each number within the specified range. When this condition is evaluated as true, the code block associated with the for loop will be executed; otherwise, it will terminate.
- Conditions provide control: The use of conditions allows programmers to introduce logical decision-making processes into their programs. By defining appropriate conditions within a for loop, different paths can be taken based on varying circumstances or user input.
- Flexibility with operators: Within these conditions, developers have access to various comparison operators such as less than (<), greater than (>), equal to (==), and not equal to (!=). These operators allow intricate comparisons and open up avenues for more complex conditional statements.
- Boolean expressions: Conditions in for loops usually involve boolean expressions which evaluate to either true or false. This binary outcome aids in determining whether certain actions should be performed or skipped during program execution.
- Conditional branching: Through well-crafted conditions, programmers can influence the flow of logic by employing concepts like if…else statements, switch cases, or nested loops. These structures enable dynamic decision-making capabilities within programs.
Operator | Description |
---|---|
< | Less than |
> | Greater than |
== | Equal to |
!= | Not equal to |
In conclusion, understanding how conditions operate within a for loop is essential to effectively control the flow of execution in computer programming languages. By utilizing logical comparisons and boolean expressions, programmers can create dynamic programs that respond to different scenarios. In the subsequent section about “Iteration in a For Loop,” we will explore how iterations interact with conditions to execute repetitive tasks efficiently.
Moving forward, let’s explore the concept of iteration within a for loop and its significance in executing repeated actions seamlessly.
Iteration in a For Loop
Having discussed the importance of conditions in a for loop, we now turn our attention to understanding iteration in this control flow structure.
Iteration is a fundamental aspect of programming that allows us to repeatedly execute a set of instructions until a specific condition is met. In the context of a for loop, iteration refers to the process of executing a block of code multiple times based on the defined parameters. To illustrate this concept, let’s consider an example:
Suppose we have an array containing the names of students in a class. We want to print each student’s name using a for loop. The for loop would iterate over each element in the array and execute the desired code (in this case, printing the name) until all elements have been processed.
To better understand how iteration works within a for loop, let’s explore some key characteristics:
- Control variable initialization: Before starting the loop, we initialize a control variable with an initial value.
- Condition evaluation: At the beginning of each iteration, the condition associated with the for loop is evaluated. If it evaluates to true, the code inside the loop executes; otherwise, the loop terminates.
- Code execution: Once inside the loop, any statements or operations specified within its body are executed.
- Control variable update: After completing one iteration, we update/control variable according to certain criteria – commonly incrementing or decrementing its value by a fixed amount.
By carefully managing these aspects during iteration, programmers can create powerful algorithms that perform complex tasks efficiently and effectively.
Benefits of Using For Loops
Iteration is an essential concept in computer programming, allowing for the repetition of a set of instructions. One common way to implement iteration is through the use of a for loop, which provides a controlled flow and efficient execution within programming languages. In this section, we will explore the control flow characteristics of for loops and their significance in computer programming.
To better understand the utility of for loops, let’s consider an example where a program needs to calculate the sum of all numbers from 1 to 100. Without using a loop construct, one would need to manually write out each number and add them together—a tedious and time-consuming process. However, by employing a for loop, programmers can achieve this task with just a few lines of code. The for loop allows us to define initialization parameters, condition statements, and update clauses that control how many times the block of code inside the loop should execute.
The benefits of using for loops are numerous:
- Efficiency: For loops provide an efficient means of performing repetitive tasks since they allow developers to specify precise conditions under which the loop should continue executing or terminate.
- Maintainability: By encapsulating repeated actions within a single block of code, for loops contribute to cleaner and more maintainable programs. This makes it easier for other programmers (and even future versions of oneself) to comprehend and modify the code if necessary.
- Readability: The structure and syntax provided by for loops make code more readable compared to alternative methods like while loops or do-while loops.
- Error reduction: With proper implementation of looping constructs like break or continue statements within a for loop, potential errors such as infinite looping or skipping iterations can be minimized.
Control Flow Characteristics | Significance |
---|---|
Initialization | Initialize variables before entering the loop |
Condition | Define when the loop should continue executing |
Update | Specify any changes made at each iteration |
In summary, for loops provide a powerful and efficient way to control the flow of execution in computer programming languages. By defining initialization parameters, condition statements, and update clauses, developers can easily perform repetitive tasks with minimal code. The benefits of using for loops include improved efficiency, maintainability, readability, and error reduction. Understanding how to utilize this looping construct is crucial for programmers aiming to write clean and effective code.