Introduction to Data Types in Computer Programming Languages
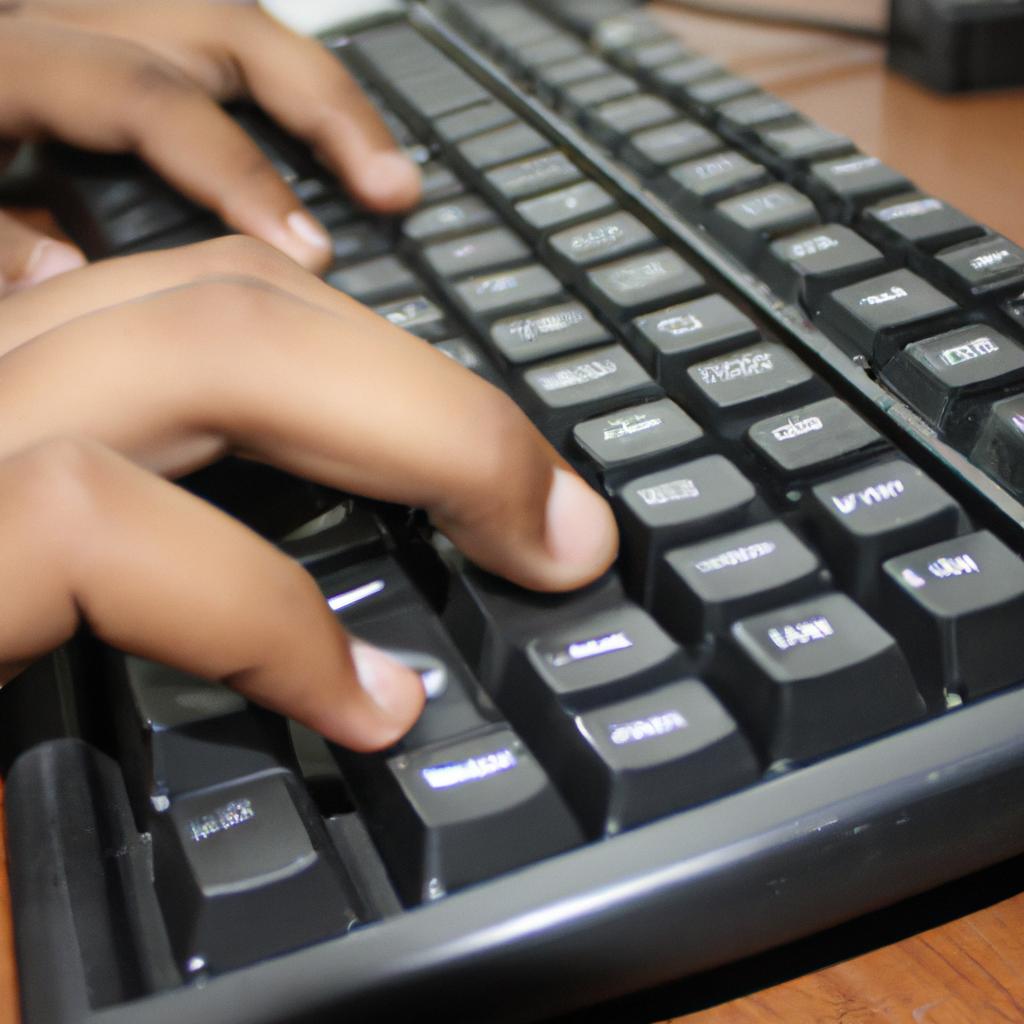
The utilization of data types is fundamental in computer programming languages as they define the nature and range of values that a variable can store. By assigning specific data types to variables, programmers ensure efficient memory allocation and precise manipulation of data during program execution. Understanding the concept of data types is crucial for aspiring programmers as it forms the foundation upon which more complex programming concepts are built. To illustrate this, consider a scenario where a programmer wants to create a simple calculator application. In order to perform arithmetic operations accurately, different data types such as integers, floating-point numbers, and characters need to be employed appropriately.
In computer programming, each programming language provides its own set of predefined data types that cater to various requirements. These data types play a significant role in determining how the code behaves and what kind of input or output can be processed by the program. For instance, integer data types enable storing whole numbers without decimal places while floating-point data types allow for decimal representation with greater precision. Additionally, character data type facilitates representing individual alphabets or symbols used in text processing tasks. The understanding and effective usage of these diverse data types not only contribute towards writing reliable and error-free programs but also enhance overall software development skills. This article aims to provide an introduction to different kinds of data types commonly used in programming languages:
-
Integer: This data type is used for storing whole numbers without any decimal places. It typically has a predetermined range of values it can store, depending on the specific programming language.
-
Floating-point: Also known as real numbers, this data type allows for representation of numbers with decimal places. Floating-point numbers have a higher precision than integers but may have limitations due to the way they are stored in memory.
-
Character: This data type is used to represent individual characters such as letters, digits, or symbols. Characters are typically stored using a character encoding system like ASCII or Unicode.
-
String: Strings are sequences of characters and are used to represent text or words in programming. They allow for manipulation and processing of textual data.
-
Boolean: A boolean data type can only take one of two possible values – true or false. Booleans are often used in conditional statements and logical operations.
-
Array: An array is a collection of elements of the same data type that are stored together under a single variable name. Arrays provide a convenient way to store and access multiple values efficiently.
-
Structs/Classes: These data types allow programmers to define their own custom complex types by combining different variables and functions into a single entity. Structs and classes help organize related data and behavior into reusable components.
-
Enumerations: Enumerations (enums) provide a way to define named constants within a program, making code more readable and maintainable.
These are just some common examples of data types found in programming languages, but there might be additional specialized data types available depending on the language being used.
Overview
Imagine a scenario where you are tasked with developing a software application that requires storing and manipulating different types of data. To accomplish this, it is crucial to have a solid understanding of data types in computer programming languages. Data types define the kind of values that can be assigned to variables and determine the operations that can be performed on them.
One common example to illustrate the importance of data types is working with numeric values. Consider a situation where you need to calculate the average grades of students from multiple subjects. If the grades were stored as strings instead of numerical values, performing mathematical calculations such as addition or division would not yield accurate results. This highlights the significance of selecting appropriate data types for specific scenarios.
To further emphasize their relevance, let’s explore some key aspects related to data types:
- Flexibility: Different programming languages offer varying degrees of flexibility when it comes to defining and using data types.
- Memory Management: The choice of data type affects memory allocation and usage within a program.
- Error Prevention: Proper use of data types helps detect potential errors early in development by enforcing rules about variable assignments and operations.
- Efficiency: Selecting an appropriate data type can improve efficiency by optimizing storage space and execution time.
Programming Language | Example Data Types | Memory Usage |
---|---|---|
C | int, float, char | Low |
Java | int, double, String | Moderate |
Python | int, float, str | High |
Understanding these aspects allows programmers to make informed decisions regarding which data type suits their needs best. In the subsequent section, we will delve into primitive data types—fundamental building blocks used in various programming languages—to gain deeper insights into their characteristics and applications.
By comprehending how different programming languages handle various data types and considering factors like flexibility, memory management, error prevention, and efficiency, programmers can effectively leverage the power of data types to develop robust and optimized software solutions. In the following section, we will explore primitive data types in more detail, building upon this foundation.
Primitive Data Types
Building upon the understanding gained from the previous section’s overview, let us now delve into the realm of primitive data types in computer programming languages. By examining their characteristics and usage, we can better comprehend how these foundational components form the building blocks of complex programs.
Primitive Data Types:
In order to grasp the concept of primitive data types, consider a scenario where you are designing a software application for an e-commerce platform. One crucial aspect would be storing information about products such as their name, price, quantity available, and customer reviews. In this context, each piece of information would fall under a specific data type that determines its representation and behavior within the program.
- Efficient memory utilization: Primitive data types allow for optimal use of system resources by allocating just enough space necessary to store values.
- Speed optimization: The simplicity inherent in working with primitive data types facilitates faster execution of operations on these values.
- Platform independence: These basic data types are typically defined consistently across different programming languages and platforms, ensuring compatibility and portability.
- Enhanced performance: Utilizing appropriate primitive data types helps reduce computational complexity and enhances overall program efficiency.
To provide a clearer overview, refer to the following table showcasing some commonly used primitive data types along with their respective ranges:
Data Type | Range | Description |
---|---|---|
int | -2^31 to (2^31)-1 | Used for whole numbers |
float | 3.4 x 10^-38 to 3.4… | Represents decimal numbers |
char | ‘A’ to ‘Z’, ‘a’ to ‘z’ | Stores single characters |
boolean | true or false | Represents logical values |
Understanding the fundamental aspects surrounding various primitive data types forms a solid foundation for programming. It enables developers to effectively manage and manipulate data within their applications, ultimately leading to more robust software solutions.
As we have now explored the intricacies of primitive data types, let us move on to examining composite data types and how they enable programmers to handle more complex structures in computer programming languages.
Composite Data Types
Let’s take a moment to explore composite data types in computer programming languages. Just like primitive data types, which we discussed earlier, composite data types are an essential component of programming and play a crucial role in organizing and manipulating complex sets of information.
To better understand this concept, let’s consider an example. Imagine you’re developing a project management application that tracks tasks assigned to different team members. Each task has various attributes such as the title, description, due date, and assigned person. In this scenario, you could create a composite data type called “Task” that encapsulates all these attributes into one cohesive unit. By doing so, you can easily manage and manipulate multiple tasks within your program.
Now let’s delve into the characteristics of composite data types:
- Complexity: Unlike primitive data types that represent simple values like integers or characters, composite data types allow programmers to combine multiple variables or elements together.
- Abstraction: With composite data types, programmers can abstract real-world entities by representing them as collections of related attributes or properties.
- Flexibility: Because composite data types can hold multiple pieces of information simultaneously, they offer flexibility when it comes to storing and accessing complex structures.
- Hierarchy: Often, composite data types can be organized hierarchically where smaller components form larger ones. This hierarchical structure allows for efficient organization and manipulation of intricate datasets.
Complexity | Abstraction | Flexibility | Hierarchy | |
---|---|---|---|---|
+ | ✔️ | ✔️ | ✔️ | ✔️ |
In conclusion, understanding composite data types is vital for any programmer aiming to work with complex systems or applications. These constructs enable us to organize vast amounts of information efficiently while maintaining abstraction and flexibility.
Transitioning to the next section, let’s now move on to exploring numeric data types in computer programming languages.
Numeric Data Types
Having explored the concept of composite data types, we now turn our attention to numeric data types. These data types play a fundamental role in computer programming languages and are used to represent numbers and perform mathematical operations.
Numeric Data Types:
To illustrate the significance of numeric data types, let’s consider a hypothetical scenario where you are developing an application that tracks financial transactions for a small business. In this case, you would need to store numerical values such as prices, quantities, and totals. Using appropriate numeric data types ensures accurate calculations and efficient memory utilization.
- Ensures precise calculations by defining the range and precision of numbers.
- Facilitates optimization of code execution by specifying memory requirements.
- Enables compatibility across different platforms and programming languages.
- Enhances readability and maintainability through clear representation of numerical values.
Table: Common Numeric Data Types
Data Type | Description | Range |
---|---|---|
int | Represents whole numbers | -2,147,483,648 to 2,147,483,647 |
float | Stores single-precision decimals | ±1.175494351E-38 to ±3.402823466E+38 |
double | Holds double-precision decimals | ±2.2250738585072014E−308 to ±1.7976931348623157E+308 |
decimal | Maintains high precision decimals | up to 28 significant digits |
By utilizing these various numeric data types effectively within your programming language, you can ensure accuracy while performing computations or managing financial information. The next section will delve into character data types which enable manipulation and storage of textual information without compromising efficiency or integrity.
Character Data Types
Introduction to Data Types in Computer Programming Languages
Numeric data types provide a foundation for storing and manipulating numerical values in computer programming languages. Building upon this, character data types allow programmers to work with individual characters or strings of characters. Now, let’s delve further into the concept of character data types.
Consider the following example: In a text-based game, a player encounters different non-player characters (NPCs) throughout their journey. Each NPC has its own unique name represented as a string of characters. By using character data types, developers can store and manipulate these names within the game’s code, enabling interactions between players and NPCs.
Character data types offer several advantages when working with textual information. Here are some key points to consider:
- Flexibility: Character data types allow for the storage of single characters or entire strings, facilitating versatile programming solutions.
- String Manipulation: With built-in functions specifically designed for character manipulation, programmers can easily modify and extract specific portions of strings.
- Internationalization: Character data types support multilingual applications by accommodating various alphabets, special symbols, and diacritical marks.
- Input Validation: When accepting user input, utilizing character data types enables validation checks on expected formats or lengths.
Name | Age | Occupation |
---|---|---|
John Doe | 32 | Software Engineer |
Jane Smith | 28 | Graphic Designer |
David Johnson | 45 | Data Analyst |
This table represents a fictional database containing personal information about individuals. The “Name” column demonstrates the use of character data types to store names as text values. This allows for efficient sorting and searching operations based on alphabetical order or partial matches.
Moving forward from our discussion on character data types, we now transition to exploring Boolean data types—the fundamental building blocks for logical expressions and decision-making in programming languages. By understanding the concept of Boolean data types, programmers gain the ability to create conditional statements that drive program flow and execution.
Boolean Data Types
Section III: Numeric Data Types
In the previous section, we discussed character data types and their significance in computer programming languages. Now, let us delve into another fundamental aspect of data types – numeric data types. Consider a scenario where you are developing a financial application that calculates interest rates for different investment options. To perform accurate calculations, it is crucial to understand how numeric data types work.
Numeric data types represent numbers in various formats and allow programmers to perform mathematical operations on them. These data types can be categorized into integer and floating-point types:
-
Integer Data Types:
- Integers represent whole numbers without decimal places.
- Examples include
int
,short
, andlong
in programming languages like C++, Java, or Python. - They are commonly used when dealing with quantities that do not require fractional values.
-
Floating-Point Data Types:
- Floating-point numbers accommodate both whole and fractional parts.
- The most common floating-point type is
float
, which typically uses 32 bits to store values. - Another widely-used type is
double
, which provides higher precision using 64 bits.
Understanding these distinctions between integer and floating-point numeric data types is essential for ensuring accuracy while performing calculations within a program.
To highlight the importance of choosing the appropriate numeric data type, consider this example case study involving an e-commerce platform’s inventory management system:
Product Name | Quantity Available |
---|---|
Laptop | 45 |
Smartphone | 128 |
Tablet | 76 |
Imagine mistakenly defining the quantity available as an integer instead of a floating-point number. This oversight would prevent precise tracking of partial units or fractions during stock updates—leading to inaccurate stock levels displayed on the website.
By correctly utilizing numeric data types suited to each circumstance, such errors can be avoided, ensuring the integrity of data and reliable program execution.
In summary, numeric data types in computer programming languages play a crucial role in handling numerical values. By understanding the distinctions between integer and floating-point types, programmers can make informed decisions when choosing appropriate data types for their applications. This knowledge ensures accurate calculations and promotes robustness within software systems.