Control Flow Graphs: Program Control in Computer Programming Languages
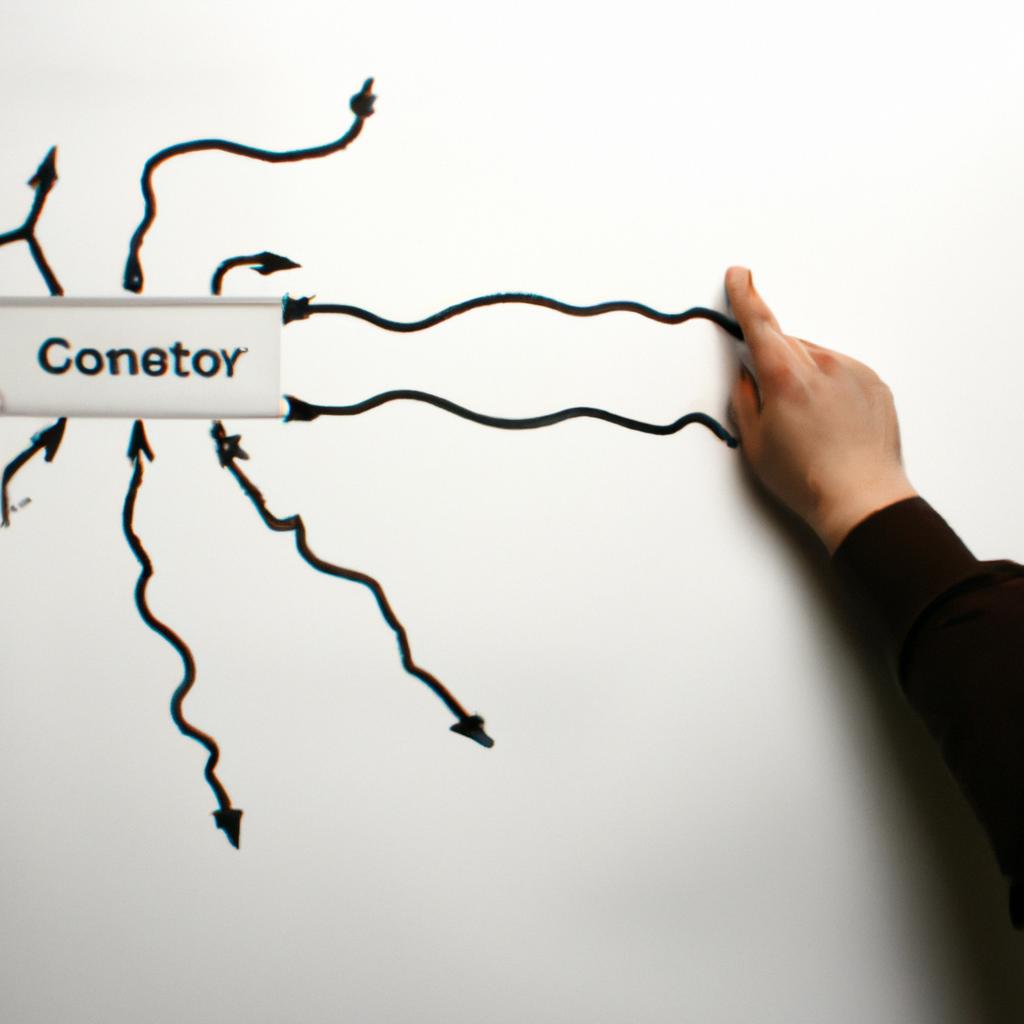
Control Flow Graphs (CFGs) play a crucial role in understanding and analyzing the program control flow in computer programming languages. CFGs provide a visual representation of how control flows through different statements and decision points within a program. By depicting the sequence of execution, branching, looping, and function calls, CFGs offer invaluable insights into the behavior and logic of programs. This article explores the significance of CFGs in computer programming languages and delves into their applications for program analysis and optimization.
To illustrate the importance of CFGs, consider a hypothetical scenario where a software developer is tasked with debugging a complex piece of code that exhibits unexpected behavior. The developer can leverage CFGs to gain a comprehensive understanding of how control is flowing through various sections of the code. By visually inspecting the interactions between statements, loops, conditionals, and functions, the developer can identify potential issues such as dead code paths or unintended side effects resulting from incorrect sequencing or conditional evaluation. Furthermore, by analyzing the structure of the CFG, developers can make informed decisions about refactoring or optimizing code to enhance performance or maintainability.
In summary, Control Flow Graphs are an indispensable tool for comprehending program control flow in computer programming languages. With their ability to visualize execution sequences and decision-making processes, CFGs help developers understand the logic and behavior of programs. They enable identification of issues such as dead code paths or unintended side effects, aiding in debugging and troubleshooting efforts. Additionally, CFGs assist in making informed decisions about code refactoring and optimization to improve performance and maintainability. Overall, CFGs play a crucial role in program analysis and serve as a valuable tool for software developers.
What Are Control Flow Graphs?
Imagine you are a computer programmer tasked with developing a complex software application. As you begin writing the code, you realize that understanding how program control flows from one statement to another is crucial for ensuring the correctness and efficiency of your program. This is where control flow graphs come into play.
Control flow graphs provide a visual representation of how program control moves through different statements or blocks of code in a computer programming language. They depict the possible paths that can be taken during execution, helping programmers analyze and reason about their programs.
To better understand the significance of control flow graphs, let’s consider an example: designing a traffic light system for a busy intersection. By creating a control flow graph for this scenario, we can identify all possible states and transitions between them. This allows us to anticipate potential issues and ensure smooth traffic management by implementing appropriate logic in our code.
Incorporating various programming constructs such as conditional statements (if-else), loops (for/while), and function calls, control flow graphs allow programmers to gain insights into how their code behaves under different scenarios. Here are some key reasons why control flow graphs are widely used:
- Code comprehension: Visual representations aid programmers in understanding complex codebases more easily.
- Error detection: By analyzing the paths in a control flow graph, developers can identify potential areas where errors may occur.
- Optimization opportunities: Control flow graphs enable programmers to identify bottlenecks or inefficiencies within their code, leading to performance improvements.
- Testing strategies: The visualization provided by control flow graphs assists in formulating effective test cases that cover all possible execution paths.
Benefits of Control Flow Graphs |
---|
Enhances code comprehension |
Facilitates error detection |
Identifies optimization opportunities |
Enables efficient testing strategies |
Understanding these benefits highlights the importance of utilizing control flow graphs when working on any software development project.
Why Are Control Flow Graphs Important in Computer Programming?
Controlling the flow of a program is crucial in computer programming languages, as it determines how instructions are executed. Control flow graphs provide a visual representation of this control flow, offering programmers a valuable tool for understanding and analyzing their code. In this section, we will delve deeper into the significance of control flow graphs and explore their various applications.
To illustrate the importance of control flow graphs, let’s consider an example scenario involving a banking system. Imagine you are tasked with developing a software application that processes customer transactions in real-time. Without effective control flow management, errors or inconsistencies could occur during transaction processing, leading to financial losses or compromised data integrity. By utilizing control flow graphs, developers can gain insights into how different parts of the program interact and identify potential issues before deployment.
One significant benefit of using control flow graphs is their ability to enhance code readability and maintainability. With complex programs containing numerous branches and loops, understanding the overall structure becomes challenging without proper visualization. Control flow graphs serve as navigational aids, allowing programmers to analyze logical paths within their code more efficiently. This increased clarity helps reduce bugs by enabling easier identification of problematic areas.
Consider these emotional responses when working with control flow graphs:
- Relief: Programmers feel relieved knowing they have a clear overview of their code’s logic.
- Confidence: Seeing all possible execution paths provides confidence in handling edge cases effectively.
- Satisfaction: Being able to optimize performance through efficient loop structures brings satisfaction.
- Empowerment: The comprehensive analysis made possible by control flow graphs empowers programmers to make informed decisions regarding improvements or bug fixes.
Emotional Responses |
---|
Relief |
Confidence |
Satisfaction |
Empowerment |
Furthermore, employing tables can be advantageous when presenting information related to control flow graphs. Take the following table as an example:
Key Components | Description | Example |
---|---|---|
Nodes | Represent individual program statements or blocks | Assignment statement |
Edges | Depict the control flow between nodes | Conditional branch |
Entry Point | The starting point of the program | Main function |
Exit Point | Marks the termination of the program’s execution path | Return statement |
In summary, control flow graphs play a vital role in computer programming by visualizing and managing the flow of instructions within a program. They improve code readability, facilitate error detection, and empower programmers to make informed decisions for optimization.
Key Components of Control Flow Graphs
Control Flow Graphs in Computer Programming Languages: Understanding the Basics
Imagine a scenario where you are designing a program that simulates an online shopping experience. The program needs to navigate through different stages, such as displaying products, adding items to the cart, and processing payments. To effectively understand and analyze the flow of this program, control flow graphs come into play. Control flow graphs provide visual representations of how control flows within a program, enabling programmers to comprehend the sequence of statements and make informed decisions about its design.
To better grasp the significance of control flow graphs, let’s consider an example. Suppose we have a simple function that calculates the factorial of a given number using recursion. By representing this function using a control flow graph, we can easily visualize how the execution proceeds at each step. This visualization aids not only in understanding how the algorithm works but also in identifying potential issues or inefficiencies.
Understanding why control flow graphs are important requires recognizing their key components:
- Nodes: These represent individual blocks of code or statements within a program.
- Edges: They signify the possible transitions between nodes based on logical conditions or procedural requirements.
- Entry Point: It denotes the starting point from which the execution begins.
- Exit Points: These indicate termination points in the program execution.
Now let us explore some emotional aspects related to control flow graphs:
-
Markdown bullet list:
- Gain greater clarity and insight into complex programs
- Detect potential bugs or errors more efficiently
- Facilitate collaboration among developers by providing a shared visual representation
- Improve overall software quality and maintainability
-
Markdown table:
Benefits Emotional Response Enhanced Understanding Confidence Efficient Bug Detection Relief Improved Collaboration Satisfaction Higher Software Quality Trust
In conclusion, understanding control flow graphs is crucial for effective program design and analysis. By visually representing the sequence of statements and transitions within a program, these graphs offer programmers valuable insights into its behavior. In the subsequent section, we will explore different types of control flow graphs and their applications in computer programming languages.
Types of Control Flow Graphs
Now that we have discussed the key components of control flow graphs, let us delve into the various types of control flow graphs commonly used in computer programming languages. To illustrate this, consider a hypothetical scenario where a software developer is designing an algorithm to determine whether a given number is prime or not. The algorithm will involve multiple conditional statements and loops to efficiently check for divisibility. This example will help us understand how different types of control flow graphs can be employed.
There are several types of control flow graphs that can be utilized based on the complexity and structure of a program. These include:
-
Sequence Control Flow: In this type, each statement is executed sequentially from top to bottom without any branching or looping constructs. It represents a linear execution path with no decision points.
-
Selection Control Flow: Also known as conditional control flow, it involves making decisions based on certain conditions. Conditional statements such as ‘if’, ‘else if’, and ‘switch’ are used to create branches in the graph, allowing different paths to be taken depending on the evaluation of these conditions.
-
Iteration Control Flow: This type incorporates looping constructs like ‘while’, ‘for’, and ‘do-while’. It enables repeated execution of a block of code until a specific condition is met or until all iterations are completed.
-
Jump Control Flow: Jump statements such as ‘goto’ or exceptions disrupt the normal sequential execution by transferring control to a specific location within the program. They allow for non-linear execution paths and are often considered less structured than other types.
To better comprehend these concepts, refer to the table below which summarizes the characteristics of each type:
Type | Description |
---|---|
Sequence | Linear execution path with no branching or looping |
Selection | Decisions made based on specified conditions |
Iteration | Repeated execution of a block of code until certain conditions are met or all iterations end |
Jump | Control transferred to specific locations within the program |
By understanding and utilizing these different types of control flow graphs, software developers can effectively analyze and optimize their programs. In the subsequent section, we will explore the advantages of using control flow graphs in computer programming languages, highlighting how they enhance program comprehension and assist in error detection and debugging.
Advantages of Using Control Flow Graphs
Control flow graphs are essential tools in computer programming languages that help visualize and analyze the program’s control flow. There are several types of control flow graphs, each serving a specific purpose in understanding and optimizing software systems.
One common type is the structured control flow graph, which represents programs with sequential execution paths. In this type of graph, nodes depict individual statements or blocks of code, while edges represent the flow between them. For example, consider a simple program that calculates the factorial of a number. The structured control flow graph for this program would consist of nodes representing input validation, iterative calculations, and output display.
Another type is the conditional control flow graph, which incorporates decision-making structures such as if-else statements or switch cases. This graph allows programmers to identify different execution paths based on conditions within the program. It can be particularly useful when analyzing complex algorithms with multiple branching points.
Additionally, there exist exceptional control flow graphs that capture error handling mechanisms like try-catch blocks or exception propagation. These graphs assist developers in understanding how exceptions propagate through their programs and enable effective debugging.
To better understand the benefits of using control flow graphs:
- They enhance code comprehension by providing a visual representation of program structure.
- They aid in identifying potential logic errors or inefficiencies during code review.
- They facilitate test case generation by revealing all possible execution paths.
- They support optimization efforts by pinpointing areas where performance enhancements can be made.
Advantages of Using Control Flow Graphs |
---|
Improved Code Comprehension |
Increased Efficiency in Optimization Efforts |
In summary, various types of control flow graphs serve as valuable tools for analyzing and optimizing software systems. Structured control flow graphs depict sequential executions, while conditional ones handle decision-making structures. Exceptional control flow graphs focus on error handling mechanisms. By leveraging control flow graphs, programmers can enhance code comprehension, improve debugging capabilities, generate efficient test cases, and optimize their programs effectively.
Moving forward to the next section about “Best Practices for Creating Control Flow Graphs,” we will delve into techniques that ensure accurate representation of program control in these graphical structures.
Best Practices for Creating Control Flow Graphs
Section H2: ‘Best Practices for Creating Control Flow Graphs’
Transitioning from the previous section on the advantages of using control flow graphs, it is important to understand the best practices involved in creating these graphical representations. By following established guidelines, developers can ensure that their control flow graphs are effective and accurately convey program control in computer programming languages.
To illustrate this, let’s consider a hypothetical scenario where a team of software engineers is working on developing a complex banking application. In order to enhance code comprehension and simplify debugging processes, they decide to create a control flow graph to represent the program logic. The example demonstrates how employing best practices can greatly benefit development efforts.
When constructing a control flow graph, several key recommendations should be considered:
- Maintain simplicity: Keep the graph as simple as possible by avoiding unnecessary complexity. Use high-level abstractions to present an overview of the program’s structure rather than diving into minute details.
- Clearly define nodes and edges: Ensure that each node represents a distinct action or decision point within the program, while edges depict the directional flow between these nodes.
- Label nodes appropriately: Use meaningful labels for each node to provide clarity and aid understanding during analysis and debugging stages.
- Utilize appropriate tools: Make use of specialized software or libraries designed specifically for generating control flow graphs efficiently and accurately.
By adhering to these best practices, developers can experience numerous benefits when working with control flow graphs:
Benefits | Description |
---|---|
Improved readability | Control flow graphs offer a visual representation that enhances code comprehension, making it easier for programmers to understand complex programs at a glance. |
Enhanced debugging | Identifying bugs becomes more efficient due to the intuitive nature of control flow graphs; errors are often more readily spotted through visual inspection compared to manual code analysis. |
Streamlined collaboration | Control flow graphs serve as a common language among team members, facilitating effective communication and collaboration during the development process. |
Code optimization | Analyzing control flow graphs can reveal potential areas for code optimization, enabling developers to improve program efficiency and performance. |
In summary, creating control flow graphs according to best practices is crucial in accurately representing program control in computer programming languages. By maintaining simplicity, labeling nodes appropriately, and utilizing suitable tools, developers can reap numerous benefits such as improved readability, enhanced debugging capabilities, streamlined collaboration, and opportunities for code optimization.