The Power of the Try-Catch: Control Flow in Computer Programming Languages
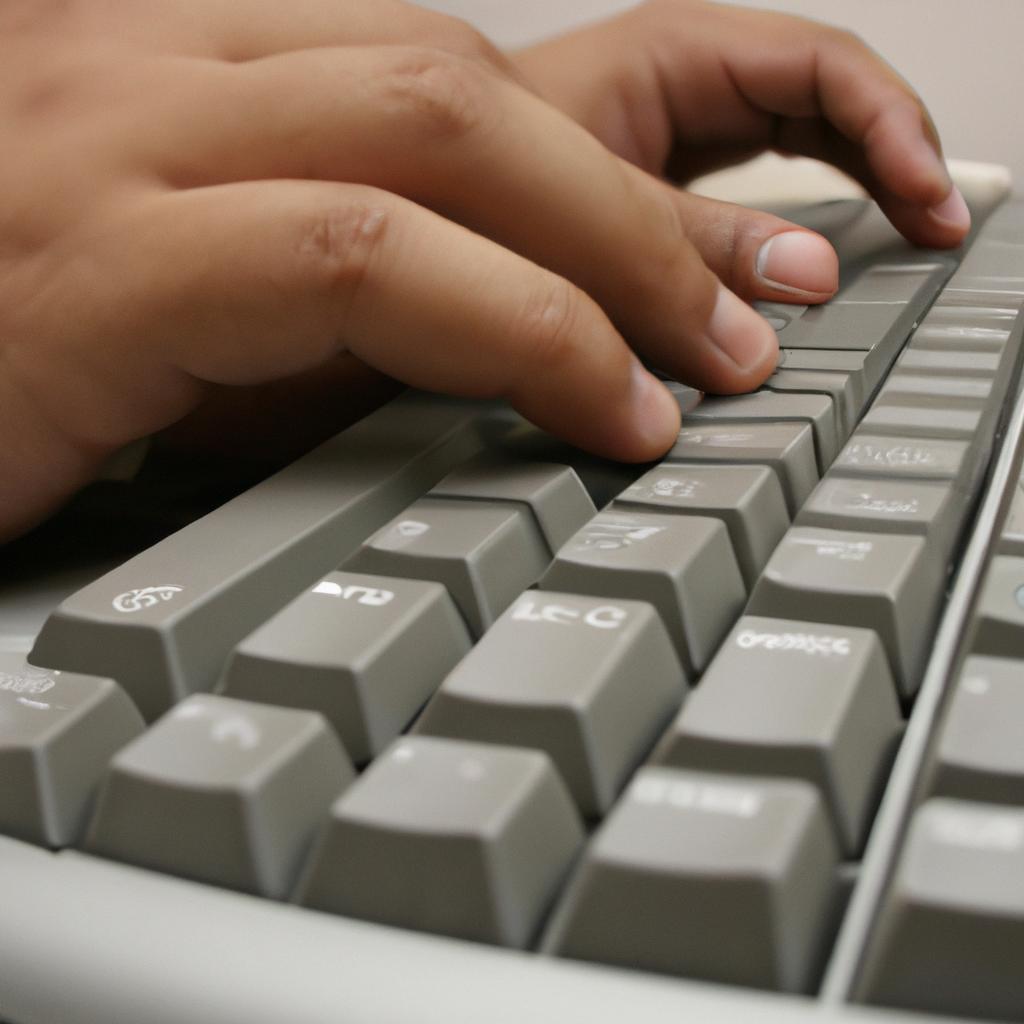
Control flow is a crucial aspect of computer programming languages that allows developers to direct the execution of code based on specific conditions or events. One powerful control structure commonly used in many programming languages is the “try-catch” block, which provides a means to handle and recover from exceptional situations during runtime. This article explores the power of the try-catch construct by examining its functionality, advantages, and real-world applications.
Consider a hypothetical scenario where a software application processes user input for authentication purposes. In this case, an exception may occur if the user enters invalid credentials or if there are any issues with connecting to the authentication server. By utilizing the try-catch mechanism, programmers can surround the potentially problematic code with a try block and define appropriate error handling procedures within catch blocks. This way, instead of abruptly crashing or producing incorrect results, the program gracefully handles exceptions by executing specific recovery actions or displaying meaningful error messages to users.
The try-catch construct not only enables better control over program execution but also enhances code reliability and maintainability. With proper utilization of try-catch blocks, developers can identify potential errors early on and take necessary measures to prevent them from causing catastrophic failures. Furthermore, this control structure promotes modularity by allowing different sections of code to have separate error handling mechanisms. This means that developers can isolate and handle specific types of exceptions in different catch blocks, making it easier to understand and maintain the codebase.
In addition to error handling, the try-catch construct can be used for other purposes such as resource management. For example, if a program needs to open a file or establish a network connection, it can encapsulate these operations within a try block. If any exception occurs during the execution of these operations, the corresponding catch block can be used to release the acquired resources properly and perform necessary cleanup tasks.
Real-world applications of try-catch blocks are vast and diverse. They are commonly used in web development frameworks to handle errors related to database interactions, network requests, or user input validation. In mobile app development, try-catch blocks are utilized to gracefully handle exceptions arising from API calls or device-specific issues. Moreover, in scientific computing or numerical analysis fields, try-catch constructs are employed to manage potential errors when dealing with complex calculations or simulations.
Overall, the try-catch construct is a powerful tool that allows developers to control and handle exceptional situations effectively. By using this control flow mechanism appropriately in their code, programmers can ensure better program reliability, maintainability, and user experience by gracefully recovering from errors instead of crashing abruptly.
Basics of error handling
Basics of Error Handling
Error handling is a crucial aspect of computer programming that allows developers to identify and address issues within their code. By effectively managing errors, programmers can enhance the reliability and stability of their applications. To illustrate the importance of error handling, consider a hypothetical scenario: imagine a banking application where users are able to transfer funds between accounts. Without proper error handling mechanisms in place, an unexpected glitch during this transaction process could result in financial losses or discrepancies for both the bank and its customers.
- Enhanced User Experience: Gracefully handling errors helps prevent application crashes or abrupt terminations, providing users with a smoother and more reliable experience.
- Improved Debugging: Properly managed exceptions allow developers to gather valuable information about potential bugs or logical flaws in their code, making it easier to diagnose and fix issues.
- Maintainability: Implementing robust error handling practices contributes to cleaner and more maintainable codebases by promoting modularization and separation of concerns.
- Security Enhancement: Effective error handling strategies not only aid in identifying security vulnerabilities but also help mitigate risks associated with malicious attacks or unauthorized access attempts.
In addition to these advantages, understanding how different programming languages handle errors is fundamental for any developer aiming to create resilient software solutions. The following table provides an overview of how three popular programming languages approach error management:
Programming Language | Approach |
---|---|
C | Utilizes return codes and conditional statements such as if…else for error detection and recovery. Exceptional cases may be handled using setjmp/longjmp constructs. |
Java | Implements try-catch blocks allowing for precise exception handling at runtime. Developers can catch specific exceptions thrown during program execution and take appropriate actions accordingly. |
Python | Employs try-except blocks enabling selective exception capture based on specific error types. It also supports the use of finally blocks, allowing for necessary cleanup operations to be performed regardless of whether an exception occurred or not. |
In summary, error handling is a fundamental aspect of computer programming that plays a vital role in creating reliable and robust software solutions. By implementing effective error management techniques, developers can enhance user experience, ease debugging processes, improve code maintainability, and bolster application security. Understanding how different programming languages handle errors provides programmers with the necessary knowledge to employ appropriate error handling mechanisms in their projects.
Transitioning into the subsequent section about “Understanding the try-catch statement,” we delve deeper into one particular error handling mechanism employed by many modern programming languages.
Understanding the try-catch statement
The Basics of Error Handling in computer programming languages set the foundation for understanding the power of the try-catch statement. Now, let’s delve deeper into this control flow mechanism and explore its significance in managing errors effectively.
Imagine a scenario where you are developing an e-commerce website that processes user transactions. An error occurs during payment processing due to unexpected input from the user. Without proper error handling, this could lead to a system crash or incorrect transaction data being stored. However, by utilizing the try-catch statement, you can gracefully handle such exceptions and prevent these undesirable consequences.
The try-catch statement is a fundamental construct in many programming languages that allows developers to define a block of code (try block) where potential errors may occur. It provides an opportunity to catch and handle those errors through exception handling mechanisms defined within a separate block (catch block). This ensures that even when errors arise, they do not disrupt the entire program execution but rather allow for controlled responses.
When implementing error handling using try-catch statements, several key benefits emerge:
- Robustness: By catching exceptions at appropriate points in your code, you can safeguard against crashes or unexpected behavior caused by specific error conditions.
- Debugging: Exception details captured within catch blocks aid in identifying the root cause of errors, making it easier to debug and fix issues efficiently.
- User experience: Effective error handling improves the overall user experience by providing meaningful feedback about encountered problems instead of abrupt program termination.
- Maintainability: The separation between normal execution logic and exceptional cases achieved by try-catch statements enhances code maintainability as it promotes clear organization and isolation of error-handling routines.
Benefit | Description |
---|---|
Robustness | Prevents crashes or undesired behavior caused by specific error conditions. |
Debugging | Captures exception details aiding in efficient identification and resolution of errors. |
User experience | Enhances user satisfaction by providing informative feedback instead of abrupt program termination. |
Maintainability | Promotes clear organization and isolation of error-handling routines, improving code maintainability in the long run. |
Understanding the power and versatility of the try-catch statement sets a solid foundation for effective error handling in programming languages. However, it is crucial to know how to handle specific exceptions when they occur. In the following section, we will explore different strategies for dealing with various types of exceptions, enabling developers to tailor their error handling mechanisms accordingly.
Handling specific exceptions
In the previous section, we explored the concept of the try-catch statement and its significance in controlling the flow of computer programming languages. Now, let us delve deeper into the practical application of this powerful mechanism by understanding how it enables programmers to handle specific exceptions.
Consider a hypothetical scenario where a program is designed to read data from a file. In this case, an exception may occur if the file does not exist or cannot be accessed. By utilizing try-catch blocks, developers can catch these specific exceptions and execute predefined error-handling code instead of abruptly terminating the program.
To illustrate further, let’s take a look at some key benefits that arise from handling specific exceptions:
- Enhanced error detection: By explicitly catching specific exceptions, programmers gain valuable insights into potential issues that might otherwise go unnoticed during runtime.
- Improved user experience: When errors are gracefully handled using try-catch statements, users are less likely to encounter unexpected crashes or cryptic error messages. This fosters trust and enhances overall usability.
- Efficient debugging process: The ability to target specific exceptions allows for more efficient debugging since developers can focus their attention on troubleshooting areas relevant to the encountered error.
- Modularized codebase: Handling different types of exceptions within separate catch blocks promotes modularization and separation of concerns in software development.
Exception Type | Description | Emotion evoked |
---|---|---|
NullReferenceError | Occurs when attempting to access a null object reference | Frustration |
FileNotFoundException | Thrown when trying to access a non-existent file | Disappointment |
DivideByZeroException | Raised when dividing an integer by zero | Confusion |
OutOfMemoryError | Indicates insufficient memory available for allocation | Anxiety |
In summary, incorporating specific exception handling through try-catch blocks empowers programmers to anticipate, manage, and respond appropriately to potential errors. By doing so, they can enhance error detection, improve user experience, streamline the debugging process, and promote modularized codebases.
Moving forward, we will explore an advanced technique known as nesting try-catch blocks that further expands the capabilities of exception handling within computer programming languages.
Nesting try-catch blocks
Handling specific exceptions is a crucial aspect of error handling in computer programming languages. Once an exception is thrown, it needs to be caught and handled appropriately to ensure the smooth execution of the program. However, there are situations where multiple types of exceptions can occur within a block of code, each requiring different handling mechanisms. This is where nesting try-catch blocks come into play.
Imagine a scenario where you have written a piece of code that reads data from a file and performs some calculations on it. While reading the file, two possible exceptions can occur: FileNotFoundException if the file does not exist, or IOException if there is an error while reading the file. To handle these exceptions effectively, you can nest one try-catch block inside another. In this case, the outer try block catches any potential FileNotFoundException, while the inner try block handles the IOException.
Nesting try-catch blocks provides developers with greater control over error handling by allowing them to handle specific exceptions at different levels of code execution. Here are some key benefits:
- Improved readability: By encapsulating related pieces of code within nested try-catch blocks, it becomes easier for other developers to understand how different exceptions are being handled.
- Granular error reporting: Nested try-catch blocks enable developers to capture specific exception details at various stages of program execution. This information helps in generating meaningful error messages or logging stack traces for debugging purposes.
- Fine-grained exception handling: With nested try-catch blocks, developers can define distinct recovery strategies based on different exceptional scenarios encountered during program execution.
- Enhanced fault tolerance: The ability to handle multiple types of exceptions efficiently ensures that even if certain parts of the code fail due to unexpected errors, the rest of the application continues running smoothly.
Exception Type | Handling Strategy |
---|---|
FileNotFoundException | Display an appropriate message indicating that the requested file was not found and prompt the user to provide a valid file name. |
IOException | Log the error details, attempt to recover if possible (e.g., by retrying the operation), and notify the user of the failure with an appropriate message. |
In summary, nesting try-catch blocks allows for targeted exception handling in computer programming languages. By encapsulating related code snippets within nested structures, developers can effectively handle multiple types of exceptions at different levels of program execution. This approach enhances readability, enables granular error reporting, facilitates fine-grained exception handling, and improves overall fault tolerance.
Moving forward, we will explore another essential aspect of exception handling: the role of the finally block.
The role of finally block
The Power of the Try-Catch: Control Flow in Computer Programming Languages
Nesting try-catch blocks allows programmers to handle exceptions at different levels within their code. By enclosing a block of code with a try statement, followed by one or more catch statements, developers can effectively manage errors and ensure smooth execution even when unexpected situations arise. Let’s consider an example to illustrate the significance of nesting try-catch blocks.
Imagine you are developing a banking application that includes a function for transferring funds between accounts. Within this function, there is a nested try-catch block structure. The outer try block encompasses the entire transfer process, while inner try blocks isolate specific operations such as deducting funds from the sender’s account and adding them to the receiver’s account. If any exception occurs during these individual steps, they will be caught and handled within the corresponding catch block without disrupting the overall flow of the transaction. This ensures data integrity and prevents potential financial discrepancies.
While nesting try-catch blocks offers flexibility in handling exceptions, it is essential to understand some best practices for effective error management:
- Keep catch blocks concise: Each catch block should focus on handling a specific type of exception rather than trying to capture all possible errors.
- Avoid excessive nesting: While nesting try-catch blocks can provide granular control over error-handling, too much nesting can make code difficult to read and maintain.
- Provide meaningful error messages: When catching exceptions, it is crucial to include informative error messages that enable users or other developers to understand what went wrong.
- Always log exceptions: Logging caught exceptions helps in troubleshooting issues later on by providing valuable insights into system behavior during runtime.
Best Practices for Effective Error Management |
---|
– Keep catch blocks concise |
– Avoid excessive nesting |
– Provide meaningful error messages |
– Always log exceptions |
In summary, through proper use of nested try-catch blocks, developers can ensure the resilience and stability of their applications. By handling exceptions at different levels, errors can be managed effectively without compromising the overall flow of the program.
Next section: Best practices for using try-catch
Best practices for using try-catch
In the previous section, we explored how try-catch blocks are used to handle exceptions in computer programming languages. Now, let’s delve into the important role played by the finally block within this exception handling mechanism.
To illustrate its significance, consider a hypothetical scenario where a program is attempting to read data from a file. In this case, an exception might occur if the file does not exist or cannot be accessed due to permission restrictions. By using a try-catch-finally construct, developers can ensure that any resources opened during execution (such as file handles) are properly closed and released back to the operating system regardless of whether an exception occurs or not.
The finally block is executed unconditionally after the completion of the try and catch blocks. Its purpose is to provide a reliable way to clean up resources and perform necessary tasks before exiting the code block. This guarantees that even if an exception is thrown and caught, critical operations like closing database connections or releasing memory will still take place.
Now, let’s explore some best practices for using the try-catch-finally construct:
- Keep try blocks focused: It is generally recommended to include only the minimum amount of code within a try block that could potentially throw an exception. This improves readability and makes debugging easier.
-
Handle specific exceptions: Instead of catching all exceptions with a generic
catch
statement, it is considered good practice to catch specific exceptions individually. This allows for more targeted error handling logic. - Avoid excessive nesting: While nesting multiple try-catch blocks may seem like a solution at first glance, it can make code harder to understand and maintain. Whenever possible, aim for flatter structures.
- Use finally sparingly: Although powerful, overusing finally blocks can lead to cluttered code. Only use them when necessary for resource cleanup or other crucial tasks.
By following these best practices, developers can ensure that their exception handling code is efficient, maintainable, and robust.
Pros of Using Finally Blocks | Cons of Using Finally Blocks |
---|---|
Ensures resource cleanup | Can introduce additional complexity |
Guarantees critical tasks are executed | May make the code harder to read and understand |
Provides a reliable way to handle exceptions gracefully | Overuse can lead to cluttered code |
In summary, the finally block plays a vital role in ensuring proper control flow during exception handling. By understanding its purpose and adhering to best practices, developers can effectively manage exceptions while maintaining clean and readable code.