The Switch Statement: Control Flow in Programming Languages
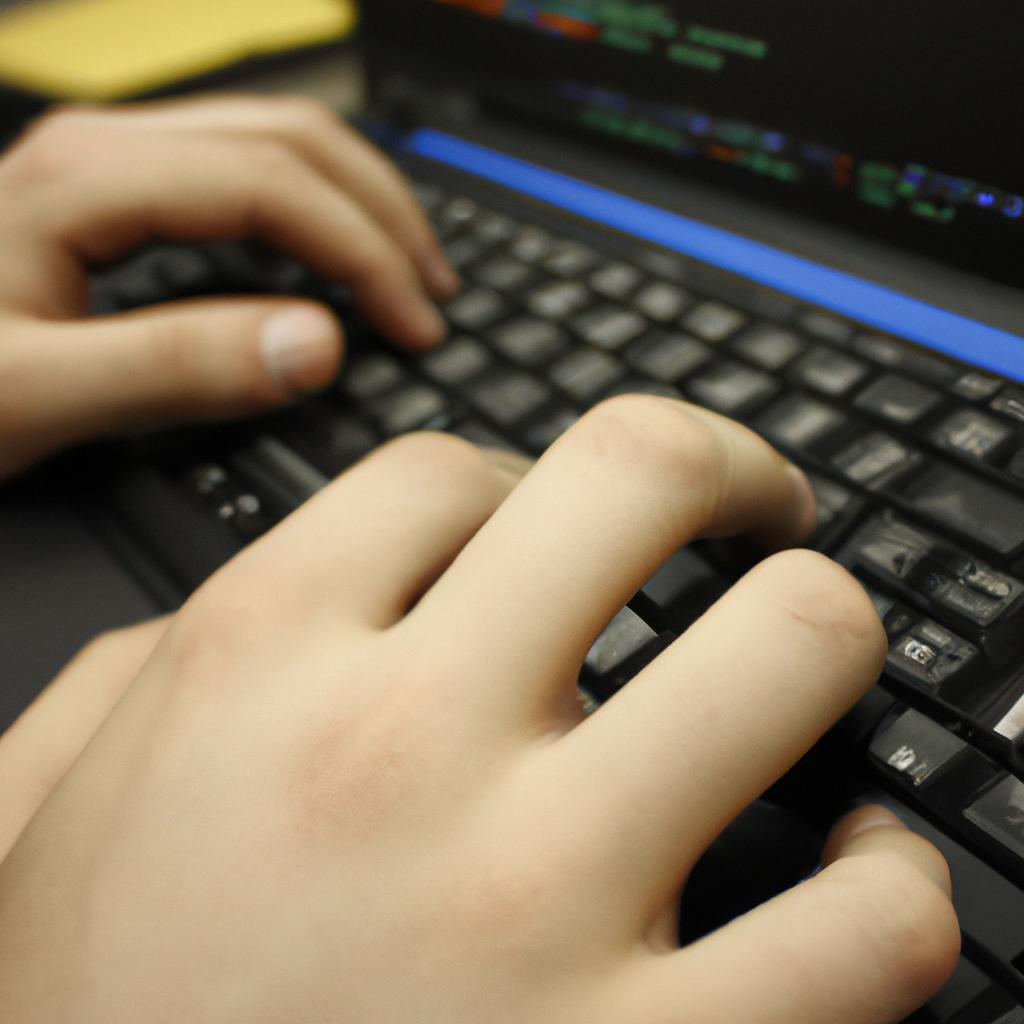
Control flow is an essential concept in programming languages, allowing developers to define the sequence of execution for a program. One powerful control flow mechanism widely used in many programming languages is the switch statement. The switch statement provides an alternative way to structure code execution based on different possible values of a single variable or expression. By comparing the value of the input against multiple cases, it directs the flow of control to specific sections of code accordingly.
To demonstrate the functionality and usefulness of the switch statement, let’s consider a hypothetical scenario involving a customer support system. Imagine a software application that receives user queries and categorizes them into different types such as technical issues, billing inquiries, or general feedback. In this case, we can utilize a switch statement to handle each type separately and execute distinct sets of instructions based on the nature of the query. This allows for more efficient and organized handling of various scenarios within the same program logic.
The switch statement not only enhances readability but also improves overall performance by minimizing redundant evaluations compared to using multiple if-else statements. Understanding its syntax and capabilities is crucial for programmers when implementing complex decision-making structures in their programs. This article aims to explore how this control flow construct works across different programming languages, highlighting its features, benefits, and best practices to ensure efficient and maintainable code.
The syntax of the switch statement generally consists of a variable or expression being evaluated, followed by multiple case blocks that define different possible values. Each case block contains the value to be compared against and a set of statements to be executed if the comparison matches. Additionally, there can be an optional default case that executes when none of the specified cases match the input value.
One key feature of the switch statement is fall-through behavior, where execution continues to subsequent case blocks even after a match is found. To prevent fall-through, most programming languages provide mechanisms like break statements that exit the switch block once a match is made.
Best practices for using switch statements include:
- Enumerating all possible cases: Ensuring that every possible value is accounted for prevents unintended behaviors.
- Ordering cases efficiently: Placing frequently encountered cases at the beginning can optimize performance.
- Using default cases wisely: Default cases should handle unexpected or invalid inputs gracefully, providing appropriate error handling or logging.
- Considering readability: Breaking down complex logic into separate functions or utilizing helper variables can make switch statements easier to understand and maintain.
- Choosing appropriate data types: Some languages allow switch statements on various data types like integers, characters, strings, or enums. Choosing the correct type based on requirements improves code clarity and reliability.
In summary, understanding and effectively using the switch statement empowers programmers to create more structured and efficient control flow in their programs. Its ability to handle different scenarios based on specific values makes it a powerful tool for organizing code execution and improving overall program readability and performance.
Definition of a switch statement
A switch statement is a control flow mechanism commonly used in programming languages to execute different code blocks based on the value of an expression. This powerful construct allows for efficient and concise decision-making within programs, enhancing their flexibility and readability.
To illustrate its functionality, let’s consider a hypothetical scenario where we are developing a simple calculator application. In this case, we can use a switch statement to determine the operation to be performed based on the user’s input. For example, if the user enters ‘+’, ‘-‘, ‘*’, or ‘/’, the program will perform addition, subtraction, multiplication, or division respectively.
One notable advantage of using a switch statement is its ability to streamline complex conditional logic by providing multiple cases that correspond to specific values. This approach enhances code organization and reduces redundancy compared to using numerous if-else statements. Additionally, as each case is evaluated independently from others, it allows for more straightforward debugging and maintenance of software systems.
The benefits of utilizing switch statements can be summarized as follows:
- Efficiency: Switch statements offer faster execution times compared to lengthy chains of if-else statements since they directly map values to corresponding actions.
- Readability: By grouping related cases together, switch statements enhance code clarity and make it easier for developers to understand the intended functionality.
- Scalability: As new requirements arise or additional cases need to be considered, adding new options within a switch statement is relatively simple without requiring significant modifications.
- Error handling: Switch statements typically include a default case that handles unexpected inputs gracefully, reducing potential runtime errors and improving overall robustness.
Input | Operation |
---|---|
‘+’ | Addition |
‘-‘ | Subtraction |
‘*’ | Multiplication |
‘/’ | Division |
In summary, the switch statement provides programmers with an elegant method for implementing multiway decisions in their code. Its efficiency, readability, scalability, and error-handling capabilities make it a valuable tool in developing complex software systems. In the following section, we will explore the syntax of a switch statement to further understand its implementation details and usage within programming languages.
Syntax of a switch statement
Imagine you are working on a program that needs to perform different actions based on the value of a variable. You could use multiple if-else statements to achieve this, but as your code grows more complex, it becomes harder to read and maintain. This is where the switch statement comes in handy. It provides an elegant way to control flow in programming languages.
Let’s consider a hypothetical scenario: you are developing a game in which players can choose from four characters – Warrior, Mage, Archer, or Rogue. Depending on the character chosen by the player, different abilities need to be assigned for gameplay. Here is how a switch statement would simplify this process:
switch (character) {
case "Warrior":
assignAbilities("sword swing", "shield block");
break;
case "Mage":
assignAbilities("fireball", "frost nova");
break;
case "Archer":
assignAbilities("bow shot", "rapid fire");
break;
case "Rogue":
assignAbilities("backstab", "stealth");
break;
}
Now let’s delve into why the switch statement is valuable:
- Clarity: Using a switch statement makes your code more readable and easier to understand, especially when dealing with multiple cases.
- Efficiency: Unlike if-else statements, a switch statement allows direct jumping to the matching case without evaluating each condition sequentially, resulting in faster execution.
- Simplicity: With its concise syntax, a switch statement reduces unnecessary repetition and simplifies coding tasks.
- Maintainability: By grouping related cases together within one construct, modifications become less error-prone and future updates require minimal effort.
Character | Abilities |
---|---|
Warrior | Sword SwingShield Block |
Mage | FireballFrost Nova |
Archer | Bow ShotRapid Fire |
Rogue | BackstabStealth |
In summary, the switch statement is an invaluable tool in programming languages. It provides a clear and efficient way to handle different cases based on the value of a variable.
How a Switch Statement Works
To understand how a switch statement operates, it’s essential to grasp its basic principles and behavior. By utilizing this control flow structure, you can streamline your code and make it more intuitive for both developers and end-users.
Now let’s delve into the inner workings of a switch statement without getting too technical.
How a switch statement works
The previous section discussed the syntax of a switch statement, highlighting its structure and how it is written in programming languages. Now, let’s delve into understanding how a switch statement functions within the context of control flow.
To illustrate this concept further, consider an example where we are building a simple calculator program. Our program receives user input for two numbers and an operator (+, -, *, or /). We want to perform different operations based on the given operator using a switch statement.
When executed, the program would evaluate the value of the operator provided by the user. The switch statement then compares this value against various cases defined within its construct. Each case represents a specific operation: addition for ‘+’, subtraction for ‘-‘, multiplication for ‘*’, and division for ‘/’.
Here are some key points about how a switch statement operates:
- The evaluation starts with checking if any of the cases match the value being tested.
- If a matching case is found, its corresponding block of code will be executed.
- After executing that particular code block, execution continues until reaching either the end of the switch statement or encountering another break keyword.
- If no matches are found among all cases, an optional default case can be included as a fallback option to execute when none of the preceding cases match.
Now let’s take a closer look at these characteristics of a switch statement through a table:
Case | Operation |
---|---|
‘+’ | Addition |
‘-‘ | Subtraction |
‘*’ | Multiplication |
‘/’ | Division |
This table provides an overview of our calculator program’s implementation using a switch statement. It shows each possible case alongside their respective operations.
In summary, utilizing a switch statement allows programmers to handle multiple conditional branches efficiently and elegantly. By evaluating values against different cases and performing corresponding actions accordingly, programs become more flexible and modularized.
Benefits of using a switch statement
In the previous section, we explored how a switch statement works in various programming languages. Now, let’s delve into the benefits that using a switch statement can bring to developers. To illustrate these advantages, consider the following hypothetical example:
Suppose you are developing a program for an e-commerce website that offers different discount options based on customer loyalty levels. With hundreds or even thousands of customers accessing your site simultaneously, efficiently managing this logic becomes crucial. By utilizing a switch statement, you can simplify and streamline your code, resulting in cleaner and more maintainable software.
There are several key benefits associated with using switch statements:
- Readability: A well-implemented switch statement allows for clear and concise code organization. It provides an intuitive way to handle multiple conditions by grouping related cases together.
- Efficiency: Switch statements often outperform alternative control flow structures like if/else chains when dealing with many possible outcomes. They utilize jump tables or indexed lookup mechanisms to efficiently locate the appropriate branch without iterating through each condition sequentially.
- Ease of maintenance: As programs evolve over time, it is common for requirements to change or new functionality to be added. When using a switch statement, modifying existing logic or introducing new cases is relatively straightforward due to its modular structure.
- Enhanced developer experience: The use of switch statements can contribute positively to developer experience by reducing cognitive load through clearer code readability and improved efficiency.
To further emphasize these points visually, let’s take a look at the table below showcasing some comparative elements between switch statements and other control flow structures:
Switch Statements | If/Else Chains | Polymorphism | |
---|---|---|---|
Readability | High | Moderate | High |
Efficiency | Fast | Variable | Variable |
Maintenance Ease | Easy | Moderate | Moderate |
Developer Experience | Positive | Variable | Positive |
As we can see, switch statements offer advantages in terms of readability, efficiency, ease of maintenance, and developer experience when compared to alternative control flow structures. However, it is important to acknowledge that switch statements also have limitations which we will explore further in the next section.
Transitioning into the subsequent section about “Limitations of switch statements,” let’s now examine some aspects where switch statements may fall short in certain scenarios.
Limitations of switch statements
Section H2: Limitations of switch statements
While the switch statement is a valuable tool in programming languages, it also has its limitations. Understanding these limitations can help programmers make informed decisions about when and how to use this control flow mechanism effectively.
One limitation of the switch statement is that it can only be used with discrete values or specific conditions. For example, if we have a program that needs to handle a wide range of continuous values, such as temperature readings from a sensor, using a switch statement would not be appropriate. In such cases, other control flow mechanisms like conditional statements or loops may be more suitable.
Another limitation is that the case labels within a switch statement must be unique and constant at compile-time. This means that dynamic or runtime-generated values cannot be used as case labels. For instance, if we want to process user input and perform different actions based on their choices, we cannot directly use a switch statement since the case labels are determined during compilation.
Furthermore, nested switch statements can become complex and difficult to read and maintain. When dealing with multiple levels of nesting, it may be more practical to restructure the code using alternative control flow structures like if-else statements or object-oriented design patterns.
In summary, while the switch statement provides an efficient way to handle multiple branches of execution based on discrete values or conditions, its usage should consider certain limitations. Programmers need to carefully evaluate whether the nature of their problem aligns well with the capabilities offered by the switch statement before incorporating it into their code.
Examples of switch statements in different programming languages will further illustrate how this construct can be utilized effectively for various scenarios.
Examples of switch statements in different programming languages
Imagine a scenario where you are working on a large-scale software project that involves processing different types of data and making decisions based on specific conditions. In such cases, the switch statement becomes an invaluable tool for controlling the flow of execution in programming languages. This section will explore the advantages of utilizing switch statements, highlighting their efficiency and flexibility.
Efficiency:
One advantage of using switch statements is their ability to improve code efficiency by reducing redundant comparisons. Unlike if-else chains, which evaluate each condition sequentially until a match is found, switch statements use jump tables or computed gotos to directly access the correct branch of code based on the input value. This approach can significantly reduce the time complexity when dealing with multiple conditional checks.
Flexibility:
Another benefit of switch statements lies in their versatility across different programming languages. While the syntax may vary slightly between languages, the fundamental concept remains consistent. Switch statements provide developers with a structured and readable way to handle multiple possible outcomes within a single control structure. Additionally, they allow for easy extensibility as new cases can be added without modifying existing code.
- Simplifies complex decision-making processes.
- Enhances code readability and maintainability.
- Encourages adherence to coding best practices.
- Boosts developer productivity and reduces errors.
Table – Comparison between if-else chains and switch statements:
If-else Chains | Switch Statements | |
---|---|---|
Readability | Can become convoluted when handling many conditions | Provides a concise structure for handling branches |
Code Duplication | May lead to duplicated code | Allows for reusable case blocks |
Efficiency | Requires sequential evaluation | Utilizes direct access through jump tables |
Extensibility | Modifications require changes at every relevant point | New cases can be added without altering existing code |
In summary, switch statements offer several advantages in terms of efficiency and flexibility. They streamline complex decision-making processes, improve code readability and maintainability, encourage adherence to coding best practices, and enhance developer productivity. By utilizing jump tables or computed gotos, switch statements reduce redundant comparisons and provide a more efficient alternative to if-else chains. Additionally, their consistent structure across different programming languages allows for seamless integration into various projects.