Conditionals in Computer Programming Syntax
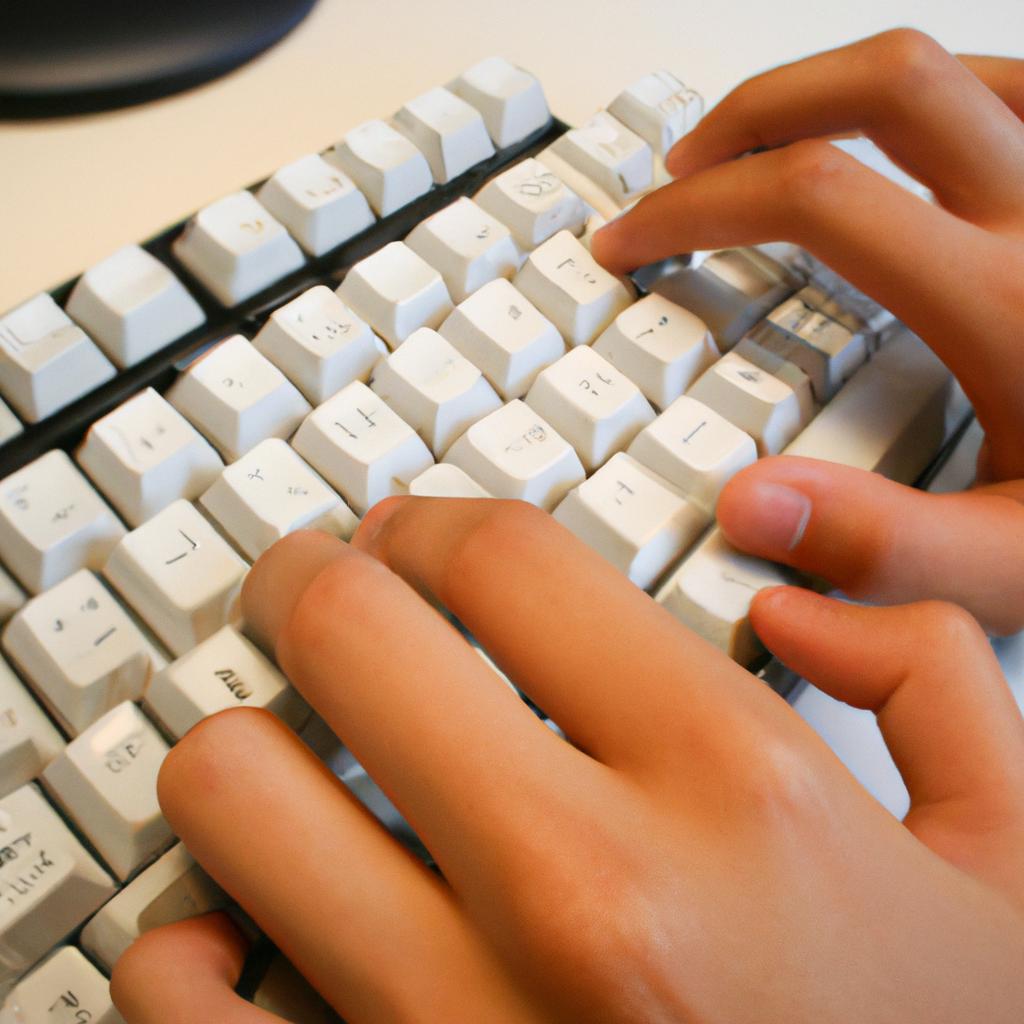
Conditionals play a crucial role in computer programming syntax, providing programmers with the ability to make decisions and control the flow of their code. These conditional statements allow programs to execute different sets of instructions based on certain conditions being met or not met. For example, consider a hypothetical scenario where a program needs to determine whether a user is eligible for an exclusive membership by checking their age. Using conditionals, the program can evaluate if the user’s age meets the required criteria, such as being above 18 years old, and then proceed accordingly.
In computer programming, mastering the use of conditionals is essential for creating efficient and dynamic software solutions. By harnessing this powerful construct, programmers can create intelligent applications that respond intelligently to various scenarios. This article explores conditionals in computer programming syntax from both theoretical and practical perspectives. It delves into the foundations of conditional logic, discussing concepts such as boolean expressions and logical operators. Additionally, it highlights common types of conditionals used in popular programming languages including “if-else” statements, “switch-case” statements, and nested conditionals. Furthermore, this article will provide real-world examples and best practices for utilizing conditionals effectively within code structures to optimize performance and enhance functionality.
In conclusion, understanding how to implement conditional statements in computer programming is essential for creating dynamic and efficient software solutions. These conditionals allow programmers to make decisions and control the flow of their code based on certain conditions being met or not met. By evaluating boolean expressions and using logical operators, programmers can create intelligent applications that respond intelligently to various scenarios.
Common types of conditionals include “if-else” statements, which execute a block of code if a certain condition is true and another block of code if it is false. “Switch-case” statements provide multiple branches of execution based on the value of a variable or expression. Nested conditionals allow for more complex decision-making by incorporating multiple levels of conditional statements within each other.
To effectively use conditionals, programmers should consider best practices such as writing clear and concise conditional expressions, avoiding unnecessary nesting, and considering edge cases. By optimizing the use of conditionals in code structures, programmers can enhance functionality and improve performance.
Overall, mastering the use of conditionals in computer programming syntax is crucial for creating intelligent software solutions that can adapt to different scenarios and make informed decisions based on specific conditions.
if statements
if statements
If statements are an essential component of computer programming syntax that allow for decision-making within a program. They provide the ability to execute specific blocks of code based on certain conditions being met. To better understand their functionality, let us consider a hypothetical scenario: creating a program that determines whether a student has passed or failed an exam based on their score.
One example of how if statements can be used in this context is as follows:
score = 75
if score >= 70:
print("Congratulations! You have passed the exam.")
else:
print("Unfortunately, you have not reached the required passing score.")
To further illustrate the significance and impact of if statements, here are some key points to consider:
- Flexibility: If statements enable programmers to create dynamic programs capable of adapting to different scenarios.
- Efficiency: By utilizing conditional logic, unnecessary computations can be avoided, leading to more efficient code execution.
- Control flow: The use of if statements allows for branching paths and alternative actions within a program’s flow.
- Decision making: With if statements, decisions and choices can be made based on various criteria or inputs.
Criteria | Action |
---|---|
Score above 90 | Print “Excellent work!” |
Score between 80 and 89 | Print “Great job!” |
Score below 80 | Print “Keep up the good work.” |
In summary, if statements play a crucial role in computer programming by allowing developers to incorporate decision-making capabilities into their programs. These constructs contribute to greater flexibility, efficiency, control flow management, and informed decision making.
Transition Sentence:
Building upon the concept of if statements, else statements serve as an integral component in expanding decision-making capabilities within computer programming syntax.
else statements
Conditionals in computer programming syntax allow for the execution of certain code blocks based on specific conditions. Building upon the previous section on “if statements,” this section will explore another important aspect of conditionals: “else statements.”
Imagine a scenario where you have developed a program to determine whether a student has passed or failed an exam. In this case, the conditional statement would be written as follows:
if score >= 70:
print("Congratulations! You have passed the exam.")
else:
print("Unfortunately, you have not met the passing criteria.")
As demonstrated above, if the student’s score is equal to or greater than 70, the message “Congratulations! You have passed the exam.” will be displayed. However, if their score falls below that threshold, they will receive the message “Unfortunately, you have not met the passing criteria.”
To further understand else statements and their significance in programming, consider these key points:
- Else statements are used when there are alternative actions to be taken depending on whether a condition evaluates to true or false.
- They provide an opportunity for error handling and contingency plans within programs.
- An else statement can only exist alongside an if statement; it cannot stand alone.
- The code block following an else statement is executed only when all preceding if/elif (else-if) conditions evaluate to false.
Consider a situation where different discount rates apply based on customer loyalty levels. A table summarizing these discounts could be represented as follows:
Customer Loyalty Level | Discount Rate |
---|---|
Platinum | 20% |
Gold | 15% |
Silver | 10% |
Bronze | 5% |
By utilizing nested conditionals within your program, you can dynamically calculate and apply appropriate discounts based on each customer’s loyalty level. This approach allows for flexible and personalized pricing strategies tailored to individual customers.
In the subsequent section on “nested conditionals,” we will explore how to further enhance your programming skills by incorporating multiple levels of conditional statements, enabling even more complex decision-making within your code. So let’s delve deeper into this fascinating topic and uncover the power of nested conditionals.
nested conditionals
Conditionals are an essential aspect of computer programming syntax, allowing programmers to execute different sets of code based on specific conditions.
Nested conditionals refer to the use of one conditional statement within another. This technique enables programmers to define multiple levels of decision-making within a program. For instance, consider a hypothetical e-commerce website that offers discounts based on customer loyalty tiers. In such a scenario, nested conditionals can be employed to determine both the customer’s eligibility for a discount and which tier they belong to. By nesting these conditionals, the program can systematically evaluate various criteria before executing relevant actions.
To illustrate further, let’s explore some key considerations when working with nested conditionals:
- Complexity: While nested conditionals allow for more precise control over program flow, it is important not to overly complicate the logic. Excessive nesting may lead to convoluted code that becomes difficult to read and maintain.
- Efficiency: Properly organizing nested conditionals can enhance program efficiency by reducing unnecessary evaluations. Careful structuring ensures that only relevant conditions are evaluated while bypassing others if certain prerequisites are not met.
- Testing: Due diligence should be exercised during testing when dealing with nested conditionals. Each level must be examined thoroughly to ensure all possible scenarios have been accounted for and correctly implemented.
- Documentation: Clear documentation plays a vital role in maintaining complex programs involving nested conditionals. Well-documented code facilitates collaboration among developers and aids future troubleshooting or modifications.
Criterion | Advantages | Challenges |
---|---|---|
Complexity | Provides precise control | May result in complexity |
Efficiency | Optimizes program flow | Requires careful planning |
Testing | Ensures thorough checks | Requires comprehensiveness |
Documentation | Facilitates collaboration | Requires clear explanations |
In summary, nested conditionals are an integral part of programming syntax that allows for multi-level decision-making. While they provide greater flexibility and control over program flow, it is crucial to strike a balance between complexity and readability. By considering factors such as efficiency, testing, and documentation when working with nested conditionals, programmers can create robust and maintainable code.
Transitioning into the subsequent section about “switch statements,” let us now explore another method used in computer programming to handle multiple conditions efficiently.
switch statements
Nested conditionals in computer programming syntax provide a way to incorporate multiple levels of decision-making within a program. By nesting one conditional statement inside another, programmers can create more complex logic flows that respond to various different scenarios. For example, consider the case study of a banking application that needs to determine whether a customer is eligible for a loan based on their income and credit score. The first level of the nested conditional could check if the customer’s income meets a minimum threshold, and if true, proceed to the second level where the credit score is evaluated. This approach allows for granular control over program execution.
When working with nested conditionals, it is important to keep certain considerations in mind:
- Code readability: As complexity increases with each additional level of nesting, it becomes crucial to maintain code clarity. Using proper indentation and commenting can help make nested conditionals easier to understand.
- Debugging challenges: With multiple layers of conditions interacting with each other, identifying errors or unexpected behavior can become more difficult. It is essential to test all possible combinations of conditions thoroughly during development.
- Performance impact: Nested conditionals may introduce overhead due to increased branching and evaluation processes. Careful consideration should be given when designing programs that heavily rely on nested conditionals to ensure optimal performance.
To illustrate these points further, consider the following hypothetical scenario involving an e-commerce website’s checkout process:
Scenario | Conditions | Action |
---|---|---|
Item out of stock | Check inventory status | Display “Out of Stock” message |
Invalid coupon code | Validate coupon code against database | Display “Invalid Coupon” alert |
Insufficient funds | Calculate total cost vs user’s account balance | Display “Insufficient Funds” notification |
Incorrect shipping address | Verify entered address format & availability | Prompt user for correct address |
Despite some potential challenges, nested conditionals can be a powerful tool in the programmer’s toolkit when used judiciously and with care. By organizing logic into multiple layers, developers gain flexibility to handle complex decision-making scenarios efficiently.
Moving forward, we will explore another useful construct in computer programming syntax known as switch statements. These statements provide an alternative approach for handling multiple conditions and offer advantages over nested conditionals in certain situations.
ternary operators
Conditionals in Computer Programming Syntax
However, there are other conditional constructs in computer programming syntax that offer more flexibility and expressiveness. One such construct is the ternary operator. The ternary operator allows for concise conditional expressions by evaluating a condition and returning one value if true, or another if false. For example:.
result = (x > 10) ? "greater than 10" : "less than or equal to 10";
This statement assigns the string “greater than 10” to the variable result
if x
is greater than 10; otherwise, it assigns the string “less than or equal to 10”. The use of the ternary operator can make code more compact and readable.
When working with conditionals in computer programming, it’s important to consider some key points:
- Condition evaluation: The conditions used in conditional statements are typically evaluated using relational operators (e.g.,
<
,>
,==
) or logical operators (e.g.,&&
,||
). It is crucial to understand how these operators work and their precedence rules. - Nested conditionals: Conditional statements can be nested within each other to handle complex decision-making scenarios. However, excessive nesting can lead to code that is difficult to read and maintain.
- Code readability versus performance: While expressive conditionals can enhance code clarity, they may also impact performance. Complex conditions or excessive branching can introduce overheads that affect program execution speed.
- Error handling: Proper error handling should be implemented when dealing with conditionals. Unexpected inputs or unforeseen conditions should be accounted for through appropriate exception handling mechanisms.
In summary, switch statements and ternary operators are two examples of powerful tools available in computer programming syntax for implementing conditionals. By understanding their usage and considering key points such as condition evaluation, nested conditionals, code readability versus performance trade-offs, and error handling, programmers can effectively utilize these constructs to write efficient and robust code.
Moving forward, we will explore another important concept in computer programming: short-circuit evaluation. This mechanism allows for the optimization of logical expressions by evaluating only the necessary parts of a compound condition.
short-circuit evaluation
Conditionals are a fundamental aspect of computer programming syntax that allow for the execution of different code blocks based on specified conditions.
Short-circuit evaluation is a mechanism employed by many programming languages to optimize conditional statements. It allows for efficient evaluation of complex expressions by stopping the evaluation process as soon as the final result can be determined without evaluating all parts of the expression. For example, consider an if statement with two conditions connected using logical AND (&&) operator: if (condition1 && condition2)
. If condition1 evaluates to false, there is no need to evaluate condition2 since both conditions must be true for the overall expression to return true. This optimization technique saves computational resources and improves program efficiency.
To better understand how short-circuit evaluation works, let’s take an imaginary scenario where a user attempts to log into a system. The login function checks whether the username exists in the database and then verifies if the entered password matches the stored one. If either condition fails, access is denied. Short-circuit evaluation comes into play here when executing these consecutive checks – if the username does not exist, there is no need to proceed with verifying the password.
The benefits of employing short-circuit evaluation in computer programs include:
- Improved performance: By avoiding unnecessary evaluations, programs run faster and consume fewer computational resources.
- Enhanced readability: Short circuiting allows developers to express complex logic more concisely and intuitively.
- Error prevention: Short-circuiting reduces potential errors caused by attempting to evaluate invalid or undefined values unnecessarily.
- Code optimization: Developers can rely on short-circuits to handle exceptional cases efficiently within their codebase.
Benefit | Description |
---|---|
Improved Performance | Faster execution due to reduced computation time |
Enhanced Readability | More concise and intuitive expression of complex logical operations |
Error Prevention | Decreased likelihood of errors caused by unnecessary value evaluations |
Code Optimization | Efficient handling of exceptional cases within the program |
In summary, short-circuit evaluation is a powerful concept in computer programming that optimizes conditional statements. By stopping the evaluation process as soon as the final result can be determined, it improves performance, enhances code readability, prevents errors, and allows for efficient code optimization. Incorporating this technique into programming practice enables developers to write more efficient and robust software systems.