Function Composition: The Power of Composing Functions in Computer Programming Languages
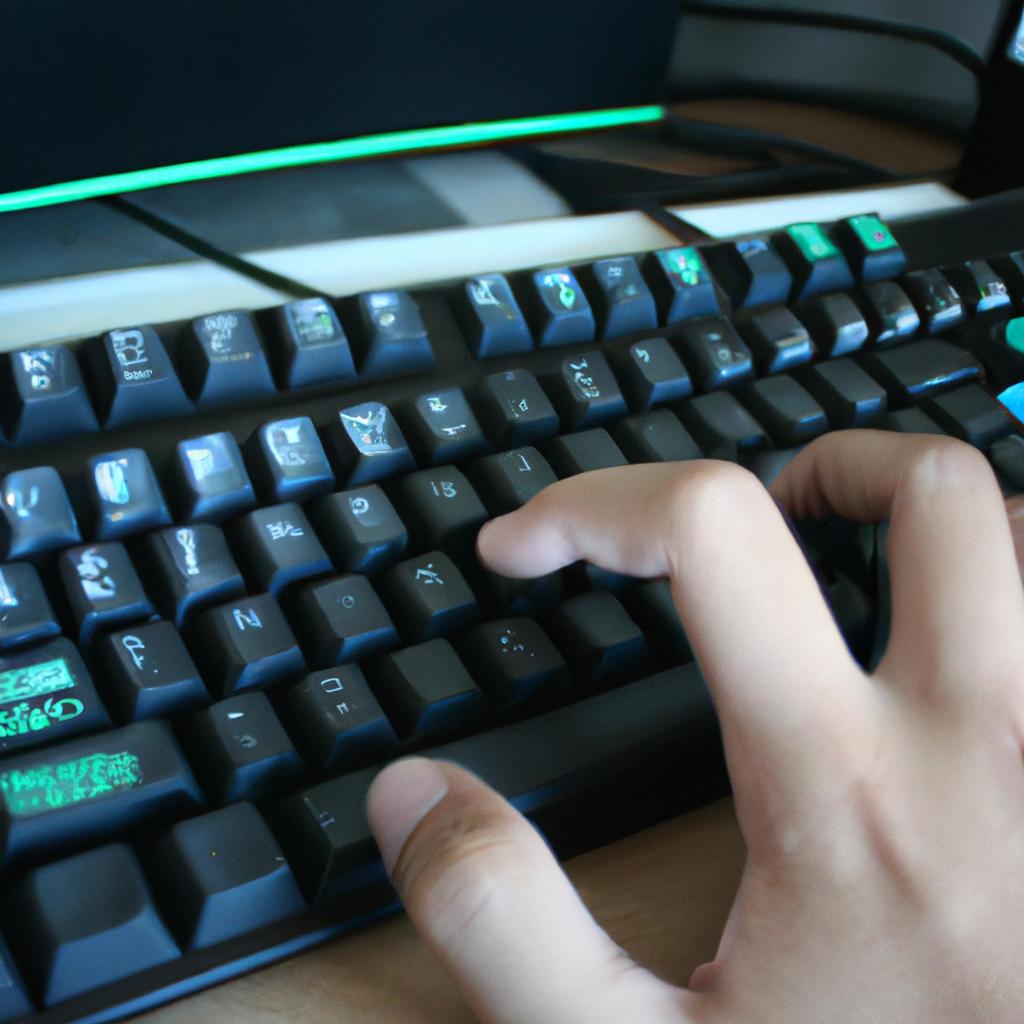
Function composition is a powerful concept in computer programming languages that allows for the combination of multiple functions to create more complex and efficient code. By taking the output of one function and using it as the input for another, developers can build intricate sequences of operations that streamline their programs. For instance, consider a hypothetical scenario where an e-commerce website needs to calculate the total cost of a customer’s purchase, including tax and discounts. Instead of writing separate functions for calculating tax and applying discounts, function composition enables the developer to combine these functionalities into a single streamlined process.
The ability to compose functions offers numerous advantages in software development. Firstly, it promotes modularity by breaking down complex tasks into smaller, reusable components. This modular approach enhances code readability and maintainability since each individual function can be tested independently before being combined with others. Additionally, function composition facilitates code reuse as existing functions can be easily repurposed or modified without affecting other parts of the program. This not only saves time but also reduces the risk of introducing errors when making changes to the codebase. Overall, understanding and utilizing function composition empowers programmers to design elegant solutions that are easier to understand, debug, and extend.
Why Function Composition is a Powerful Tool
Imagine you are a software developer tasked with creating a program that calculates the total revenue generated by an e-commerce platform. In order to accomplish this, you need to perform various calculations such as summing up sales figures, applying discounts, and calculating taxes. Each calculation requires multiple steps and involves different functions.
Function composition provides a solution to simplify complex computations like these. By combining smaller functions into larger ones, function composition allows for more efficient and concise code. For instance, instead of writing separate functions for each step in the revenue calculation process, you can compose them together to form a single function that performs all necessary operations.
The power of function composition lies not only in its ability to streamline code but also in its potential impact on productivity and maintainability. Here are some key benefits:
- Modularity: Function composition encourages modular design by breaking down complex tasks into smaller, reusable functions. This promotes code reusability and enables developers to focus on individual components without worrying about the overall logic.
- Readability: Composing functions enhances code readability by providing clear connections between different parts of the program. It allows programmers to express complex computations using simple function calls, making it easier for others (and future selves) to understand and modify the code.
- Flexibility: With function composition, you can easily customize or extend functionality by rearranging or replacing individual functions within the composed sequence. This flexibility enables developers to adapt their programs quickly to changing requirements or optimize specific aspects without rewriting large portions of code.
- Debugging: When an error occurs during execution, function composition simplifies debugging efforts since problems often originate from one particular function rather than the entire sequence. Isolating issues becomes easier when each small piece is testable individually before being composed together.
Benefits of Function Composition |
---|
Promotes modularity |
Enhances code readability |
Offers flexibility |
Simplifies debugging |
In summary, function composition is a powerful tool that enables developers to create more efficient and maintainable code. By breaking down complex tasks into smaller functions and combining them together, it enhances modularity, readability, flexibility, and ease of debugging. In the following section, we will delve deeper into understanding the basics of function composition and explore its practical applications in computer programming languages.
Understanding the Basics of Function Composition
Transitioning from the concept of why function composition is a powerful tool, let us delve into understanding its basics. To illustrate this, consider a hypothetical scenario where we have two functions: square
and double
. The square
function takes a number as input and returns its square, while the double
function takes a number as input and returns its double value. Now imagine that we want to apply these two functions consecutively on an input value of 3. By using function composition, we can achieve this by simply combining the square
and double
functions together in a new function.
Function composition allows for the creation of more complex functions by combining simpler ones. It involves chaining multiple functions together so that the output of one function becomes the input for another. This process enables developers to write reusable code components and build more concise programs.
To understand how function composition works, it’s important to grasp some key concepts:
- Input and Output: Each function within the composition has defined inputs and outputs.
- Order Matters: When composing multiple functions, pay attention to their order as it affects the final result.
- Multiple Compositions: Functions can be composed with other compositions to create even more intricate behavior.
- Pure Functions: For predictable results, it is crucial that each individual function used in composition follows the principles of being pure.
In summary, function composition provides programmers with a way to combine simple functions into more complex operations. It facilitates code reuse and promotes cleaner, more readable program structures.
Advantages of Function Composition |
---|
Code Reusability |
Modularity |
Readability |
Debugging Ease |
The benefits of function composition extend far beyond these advantages, as we will explore in the subsequent section. By understanding the basics and principles behind function composition, you can leverage this powerful technique to enhance your programming skills and develop more efficient software solutions.
Transitioning into the next section about “Benefits of Using Function Composition,” let us now delve deeper into how this approach brings added value to computer programming languages.
Benefits of Using Function Composition
Now, let’s delve deeper into this concept and uncover its various benefits.
To illustrate the potential of function composition, consider an example scenario where you have two functions: calculateTax()
and applyDiscount()
. The calculateTax()
function takes an input price and calculates the tax amount based on a given percentage. On the other hand, the applyDiscount()
function applies a discount to a given price value. By composing these two functions together, we can create a new function that first calculates the tax and then applies the discount to produce the final price.
The benefits of using function composition are numerous:
- Code Reusability: Function composition allows us to reuse existing functions by combining them in different ways to perform complex operations without having to rewrite code from scratch.
- Modularity: Composing functions promotes modularity as each individual function performs a specific task or computation. This modular approach enhances code readability and maintainability.
- Flexibility: With function composition, we can easily modify or extend functionality by adding or removing intermediate steps within the composed sequence of functions.
- Efficiency: Function composition often leads to more efficient code execution since it avoids unnecessary variable assignments and reduces redundancy.
Benefit | Description |
---|---|
Code Reusability | Allows for reuse of existing functions |
Modularity | Enhances code readability and maintainability |
Flexibility | Enables easy modification or extension of functionality |
Efficiency | Leads to more efficient code execution |
By employing function composition, developers gain access to these advantages which ultimately contribute towards writing cleaner, reusable, and optimized code. In our next section, let’s explore some common mistakes that programmers should avoid when utilizing function composition techniques.
Transitioning into Common Mistakes to Avoid in Function Composition, it is crucial to be aware of potential pitfalls that can hinder the effectiveness of your code.
Common Mistakes to Avoid in Function Composition
Section H2: Common Mistakes to Avoid in Function Composition
Transitioning from the previous section on the benefits of using function composition, it is crucial to be aware of common mistakes that programmers often make when working with this powerful technique. By understanding these pitfalls and learning how to avoid them, developers can harness the full potential of function composition effectively.
To illustrate one such mistake, consider a hypothetical scenario where a programmer wants to calculate the average age of a group of individuals. They define two functions – getAges
, which retrieves an array of ages from a given dataset, and calculateAverage
, which calculates the mean value of an array. In their excitement to compose these two functions for efficiency, they accidentally pass the entire dataset directly into calculateAverage
. As a result, instead of receiving an array of ages as intended, calculateAverage
receives unrelated data and produces incorrect results.
To prevent missteps like this, here are some common mistakes to watch out for in function composition:
- Incorrect order: Careless arrangement of composed functions can lead to erroneous outputs. It is essential to ensure that functions are composed in the correct order based on their dependencies.
- Incompatible input/output types: Composing functions with incompatible input or output types will result in type errors during execution. Developers should carefully validate whether the inputs and outputs align correctly before composing functions.
- Not handling error cases: Neglecting error-handling scenarios within individual functions might propagate unexpected behavior throughout compositions. Properly accounting for possible errors ensures robustness and reliability.
- Overlooking performance implications: While function composition offers code reuse and conciseness advantages, excessive nesting or unnecessary combinations can negatively impact performance. Considering computational complexity is vital when composing complex functionality.
By avoiding these common mistakes through careful planning and attention to detail, developers can optimize their use of function composition for efficient code design and implementation.
With an understanding of both the benefits and potential pitfalls of function composition, the subsequent section will delve into advanced techniques that can further enhance its use in computer programming languages. Transitioning seamlessly into these advanced concepts, programmers can unlock even greater possibilities for their code.
Next section: H2 – Advanced Techniques for Function Composition
Advanced Techniques for Function Composition
Having discussed the common mistakes to avoid in function composition, it is now time to explore some advanced techniques that can further enhance the power and flexibility of composing functions within computer programming languages.
To illustrate these advanced techniques, let’s consider a hypothetical scenario where we are developing an e-commerce application. Our goal is to create a streamlined checkout process by composing various functions to handle different aspects of the transaction flow. By leveraging these advanced techniques, we can achieve greater efficiency and maintainability in our codebase.
One powerful technique is partial application, which allows us to fix certain arguments of a function while leaving others open for later use. This enables us to create specialized versions of existing functions without duplicating their entire implementation. For example, in our e-commerce application, we could define a partially applied function that takes a fixed payment method as an argument and returns a new function capable of processing payments with that specific method. This approach promotes code reuse and makes our overall system more modular.
Another technique worth exploring is currying, which involves transforming a function that takes multiple arguments into a series of functions each taking only one argument. Currying enhances composability by enabling us to easily chain together smaller functions into more complex ones. In our e-commerce application, we could curry the validation step of the checkout process so that each individual validation rule becomes its own separate function. These smaller functions can then be composed together in any desired order or combination, making it easier to reason about and modify the validation logic.
Lastly, higher-order functions play a crucial role in advanced function composition techniques. A higher-order function is one that either takes another function as an argument or returns a function as its result. They provide great flexibility when it comes to combining and manipulating functions at runtime. In our e-commerce application, we could utilize higher-order functions to dynamically generate different checkout flows based on user preferences or business rules.
- Increased code reusability and modularity
- Enhanced composability for complex logic
- Greater flexibility in creating dynamic workflows
- Improved maintainability and readability of the codebase
Emotional Table:
Technique | Benefits | Example |
---|---|---|
Partial Application | Code reuse, modularity | const processCreditCard = paymentProcessor('credit_card'); |
Currying | Composability, readability | const validateAddress = curry(validationRules.address); |
Higher-order Functions | Dynamic workflows, flexibility | const checkoutFlowGenerator = (preferences) => generateCheckoutFlow(preferences); |
In summary, by employing advanced techniques such as partial application, currying, and higher-order functions, programmers can elevate the power of function composition within computer programming languages. These techniques offer benefits like increased code modularity, enhanced composability, greater flexibility in creating dynamic workflows, improved maintainability, and more readable code.
Real-World Examples of Function Composition
Section: The Benefits of Function Composition
In the previous section, we explored advanced techniques for function composition, showcasing its versatility and power in computer programming languages. Now, let us delve deeper into the practical benefits that arise from composing functions. To illustrate these advantages, consider a hypothetical scenario where we are developing a web application that requires complex data processing.
Imagine we have two separate functions: one to fetch data from an external API, and another to transform and filter this retrieved data. By employing function composition, we can seamlessly combine these two functions into a single unit of code. This not only enhances code readability but also improves maintainability by encapsulating related functionality within a single entity.
The following bullet point list highlights some key benefits of utilizing function composition:
- Modularity: Function composition allows developers to break down complex tasks into smaller, more manageable units. Each individual function performs a specific task or transformation on the data, making it easier to understand and test.
- Reusability: Composing functions promotes reusability as independent units can be used across multiple projects or scenarios without duplication of code.
- Flexibility: With function composition, developers gain flexibility in modifying or extending existing functionality. By rearranging or adding new composed functions, they can adapt their codebase to accommodate evolving project requirements.
- Debugging Ease: When errors occur during execution, function composition helps pinpoint the problematic area quickly since issues are isolated within individual functions rather than being scattered throughout the entire codebase.
To further emphasize the benefits mentioned above, consider the table below which compares traditional imperative programming with functional programming through the lens of function composition:
Traditional Imperative Programming | Functional Programming with Function Composition | |
---|---|---|
Code Structure | Long sequences of instructions | Modular and reusable units |
Data Handling | Mutating variables | Immutable data structures |
Side Effects | Common, leading to unpredictable behavior | Limited and controlled side effects |
In conclusion, function composition empowers developers with a range of advantages when designing and implementing software systems. By breaking down complex tasks into smaller functions and composing them together, code becomes more modular, easier to debug, and adaptable to changing requirements. These benefits contribute to improved code maintainability, reusability across projects, and overall developer productivity.