First-Class Functions: The Role in Computer Programming Languages
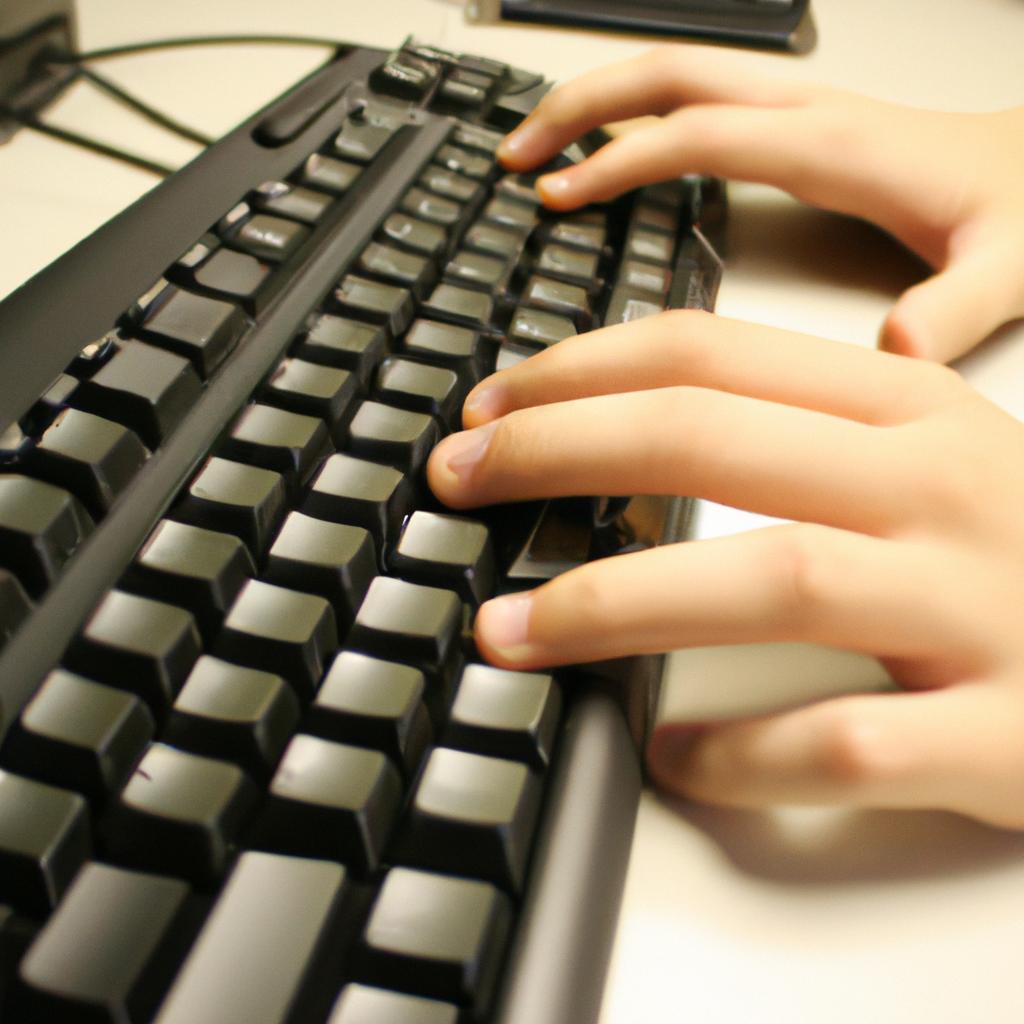
First-class functions play a pivotal role in computer programming languages, facilitating the implementation of advanced programming techniques and enabling greater flexibility and efficiency in code development. These functions are characterized by their ability to be assigned to variables, passed as arguments to other functions, and returned as values from other functions. By treating functions as first-class citizens within a programming language, developers gain access to powerful tools that can greatly simplify complex tasks.
To illustrate the significance of first-class functions, consider the following hypothetical scenario: a software developer is tasked with creating a program that processes a large dataset of customer information. The requirements stipulate that certain operations need to be performed on each record in the dataset, such as filtering based on specific criteria or applying transformations to selected fields. Without first-class functions, the developer would need to write separate blocks of code for each operation or resort to using repetitive control structures. However, by leveraging first-class function capabilities, the developer can define reusable functions that encapsulate these operations and pass them as arguments to higher-order functions responsible for iterating over the dataset. This not only reduces code duplication but also allows for more concise and modular program design.
Definition of First-Class Functions
In the world of computer programming languages, first-class functions play a crucial role in enabling programmers to write efficient and flexible code. Simply put, a first-class function refers to a function that can be treated as a value, allowing it to be assigned to variables, passed as arguments to other functions, and returned as values from other functions.
To illustrate this concept, let’s consider an example where we have a program that needs to sort a list of names alphabetically. Traditionally, one would define a sorting algorithm and pass the list of names as an argument. However, with the inclusion of first-class functions in programming languages like Python or JavaScript, we could instead treat the sorting algorithm itself as a parameter. This means that different sorting algorithms can be easily interchanged without modifying the main codebase.
The use of first-class functions brings several advantages for programmers:
- Code reusability: By treating functions as values, they can be reused across multiple parts of a program without duplicating code.
- Higher-order functions: First-class functions enable the creation of higher-order functions – those that take other functions as parameters or return them as results. This allows for greater abstraction and modularity in coding practices.
- Function composition: With first-class functions, it becomes possible to compose complex functionalities by combining simpler ones. This promotes code readability and maintainability.
- Dynamic behavior: The ability to assign functions to variables at runtime opens up opportunities for dynamic behavior within programs. It enables developers to adapt their software based on changing conditions or user inputs.
Advantages of First-Class Functions |
---|
Code reusability |
In summary, first-class functions provide programmers with powerful tools for creating more versatile and robust software systems. Their ability to treat functions as values facilitates code reuse, supports higher-order functionality, promotes function composition, and enables dynamic behavior. In the following section, we will delve into the specific benefits that first-class functions offer to developers in more detail.
Benefits of First-Class Functions
Having discussed the definition and significance of first-class functions in computer programming languages, we now turn our attention to their practical applications. To illustrate the versatility and power of this concept, let us consider an example scenario where first-class functions play a crucial role.
Example Scenario: Imagine a web application that allows users to interact with various data sources by performing operations such as filtering, sorting, and transforming the data. In this hypothetical situation, first-class functions prove invaluable as they enable developers to pass functions as arguments or return them from other functions effortlessly. By doing so, programmers can design flexible and reusable code components, enhancing both maintainability and scalability.
Use Cases of First-Class Functions:
First-class functions offer numerous benefits when it comes to solving real-world problems in software development. Here are some common use cases where leveraging first-class functions proves advantageous:
- Event handling: With first-class functions, event-driven programming becomes more straightforward. Developers can assign callback functions to events triggered by user interactions or system notifications.
- Higher-order functions: These allow for the composition of complex algorithms by combining smaller reusable function units. This results in cleaner code structure and improved modularity.
- Asynchronous execution: First-class functions facilitate asynchronous programming paradigms like callbacks or promises by enabling delayed execution or passing callbacks between different parts of a program.
- Functional programming paradigms: First-class functions form the foundation for functional programming approaches like map-reduce patterns, currying, or partial application.
The table below summarizes these key use cases along with their corresponding advantages:
Use Case | Advantage |
---|---|
Event handling | Enables interactive user experiences |
Higher-order funcs | Enhances code organization and modularization |
Asynchronous exec | Facilitates non-blocking I/O operations |
Functional prog | Promotes clean code and enables functional composition |
In conclusion, the versatility of first-class functions extends beyond theoretical concepts. By enabling developers to pass functions as arguments or return them from other functions, they prove invaluable in various real-world scenarios.
With an understanding of the use cases established, let us now delve into concrete examples showcasing how first-class functions are utilized across a range of programming disciplines.
Use Cases of First-Class Functions
Transitioning from the benefits of first-class functions, it is important to explore their practical applications in computer programming languages. To illustrate this, let us consider a hypothetical scenario where a software developer is building an e-commerce platform. The developer wants to implement a feature that allows customers to sort products by different criteria such as price, popularity, and customer ratings. This can be achieved using first-class functions.
Firstly, one benefit of first-class functions in this context is the ability to pass functions as arguments to other functions. In our example, the developer could create a sorting function that accepts another function as an argument – a comparison function. By passing different comparison functions (e.g., one for price, one for popularity), the programmer can dynamically change how the products are sorted without duplicating code.
Secondly, first-class functions enable developers to return functions from other functions. Continuing with our e-commerce example, imagine a situation where the users can customize their product sorting preferences. The developer could use first-class functions to create higher-order sorting functions that encapsulate different user preferences and return them accordingly.
Lastly, first-class functions facilitate the creation of anonymous or lambda functions on-the-fly within programs. These lightweight and disposable functions can be useful when writing concise code or performing quick calculations inline. For instance, if our imaginary e-commerce platform needs to calculate discounts based on various conditions like purchase history or membership status, anonymous functions could be employed to evaluate those conditions efficiently.
- Enhanced flexibility and adaptability
- Increased code reusability and maintainability
- Streamlined development process
- Empowerment for programmers to solve complex problems creatively
Additionally, we present a table showcasing some popular programming languages along with their support for first-class function features:
Language | Supports Function as Argument? | Supports Function as Return Value? | Allows Anonymous Functions? |
---|---|---|---|
Python | Yes | Yes | Yes |
JavaScript | Yes | Yes | Yes |
Java | No | No | No |
C++ | Yes | Yes | No |
In summary, first-class functions play a crucial role in modern computer programming languages. Their ability to be passed as arguments, returned from functions, and created on-the-fly brings numerous benefits to developers. The flexibility they provide allows for elegant and efficient code solutions, enhancing the overall development process.
Transitioning seamlessly into the subsequent section about “Examples of First-Class Functions in Programming Languages,” we delve further into practical use cases that demonstrate the power and versatility of this concept.
Examples of First-Class Functions in Programming Languages
Transitioning from the previous section exploring the concept of first-class functions, let us delve into the various use cases where these powerful features find application. To exemplify their versatility, consider a scenario where a web developer is building an e-commerce website that offers discounts and promotions to customers based on certain criteria. By utilizing first-class functions, the developer can create reusable code snippets that encapsulate different discount calculation algorithms. These functions can then be passed as arguments to other functions or stored in variables for later use.
One compelling advantage of using first-class functions is their ability to enhance code modularity and reusability. This flexibility allows developers to write concise and efficient programs by separating concerns and reducing duplication. Furthermore, first-class functions enable higher-order programming paradigms such as functional programming, allowing for elegant solutions to complex problems through composition and function chaining.
To emphasize the significance of first-class functions further, let’s explore some key benefits they bring to computer programming:
- Increased abstraction: First-class functions provide a level of abstraction that enables programmers to focus on high-level problem-solving rather than dealing with low-level implementation details.
- Code expressiveness: With the ability to pass functions as arguments or return them as values, developers can achieve more expressive code that accurately represents their intentions.
- Concurrency support: First-class functions facilitate concurrent programming by enabling the creation and manipulation of lightweight threads or processes within programs.
- Domain-specific language development: Leveraging first-class functions empowers developers to design domain-specific languages tailored specifically for particular problem domains, leading to more intuitive and readable code.
Now let us move forward to examine specific examples of how different programming languages implement and utilize first-class functions effectively.
[End paragraph]
Comparison of First-Class Functions in Different Programming Languages
In the previous section, we explored various examples of first-class functions in programming languages. Now, let’s delve deeper into the role and significance of these functions in computer programming.
To illustrate the practical implications of first-class functions, consider a hypothetical scenario where you are developing a web application that requires dynamic event handling. In this case, having first-class functions allows you to pass function references as arguments to other functions or store them within data structures. This flexibility enables you to create reusable code components for different events without duplicating your implementation logic.
First-class functions offer several advantages that enhance the efficiency and maintainability of software systems:
- Code modularity: With first-class functions, developers can encapsulate functionality within separate units that can be easily reused throughout their programs.
- Higher-order functions: By treating functions as values, higher-order functions become possible. These are functions that either take one or more functions as parameters or return a function as its result. This capability facilitates the creation of abstractions and design patterns.
- Asynchronous programming: First-class functions enable asynchronous programming paradigms by allowing callbacks to be passed around and executed at later stages when certain conditions are met.
- Functional programming: Functional programming languages rely heavily on first-class functions since they allow for operations like mapping, filtering, reducing, and composing on collections of data.
Now that we have examined the role and benefits of first-class functions in programming languages let us proceed to the next section: “Challenges and Limitations of First-Class Functions.” Understanding these challenges will provide insights into potential drawbacks programmers might face when utilizing this powerful feature in their applications.
Challenges and Limitations of First-Class Functions
In the previous section, we explored the concept of first-class functions and their significance in computer programming languages. Now, let us delve into a comparison of how different programming languages handle first-class functions. To illustrate this comparison, let’s consider an example scenario where developers are tasked with creating a web application that requires extensive use of first-class functions.
Firstly, in Python, a dynamically-typed language known for its simplicity and readability, first-class functions can be easily created using lambda expressions. These anonymous functions allow developers to define small chunks of code on-the-fly without needing to assign them to named variables. This flexibility enables programmers to pass these functions as arguments to other higher-order functions or store them as data structures like lists or dictionaries.
On the other hand, JavaScript offers powerful support for first-class functions due to its functional programming capabilities. Developers can create function literals and assign them to variables just like any other data type. Additionally, JavaScript provides built-in array methods such as map(), filter(), and reduce() which accept callback functions as arguments. This allows for concise and expressive code when manipulating arrays.
Meanwhile, in Java, a statically-typed object-oriented language, first-class functions have traditionally been less prominent. However, with the introduction of Java 8’s lambdas and functional interfaces, working with first-class functions became more practical. While not as flexible as Python or JavaScript due to its strict typing system, Java now supports passing behavior directly through method references or lambda expressions.
To summarize the comparison:
- Python: Offers simplicity and ease-of-use with lambda expressions.
- JavaScript: Provides robust functional programming capabilities and convenient array manipulation methods.
- Java: Has improved support for first-class functions through lambdas and functional interfaces.
This table presents a summary of the differences between these three languages regarding first-class function handling:
Language | Lambda Support | Functional Programming Capabilities |
---|---|---|
Python | Yes | Limited, but expressive with lambda syntax |
JavaScript | Yes | Extensive support for functional programming |
Java | Yes (since Java 8) | Improved, but limited due to static typing |
As we have seen, various programming languages differ in their approaches to handling first-class functions. The choice of language depends on the specific requirements and preferences of developers.