Anonymous Functions: A Dive into Functions in Computer Programming Languages
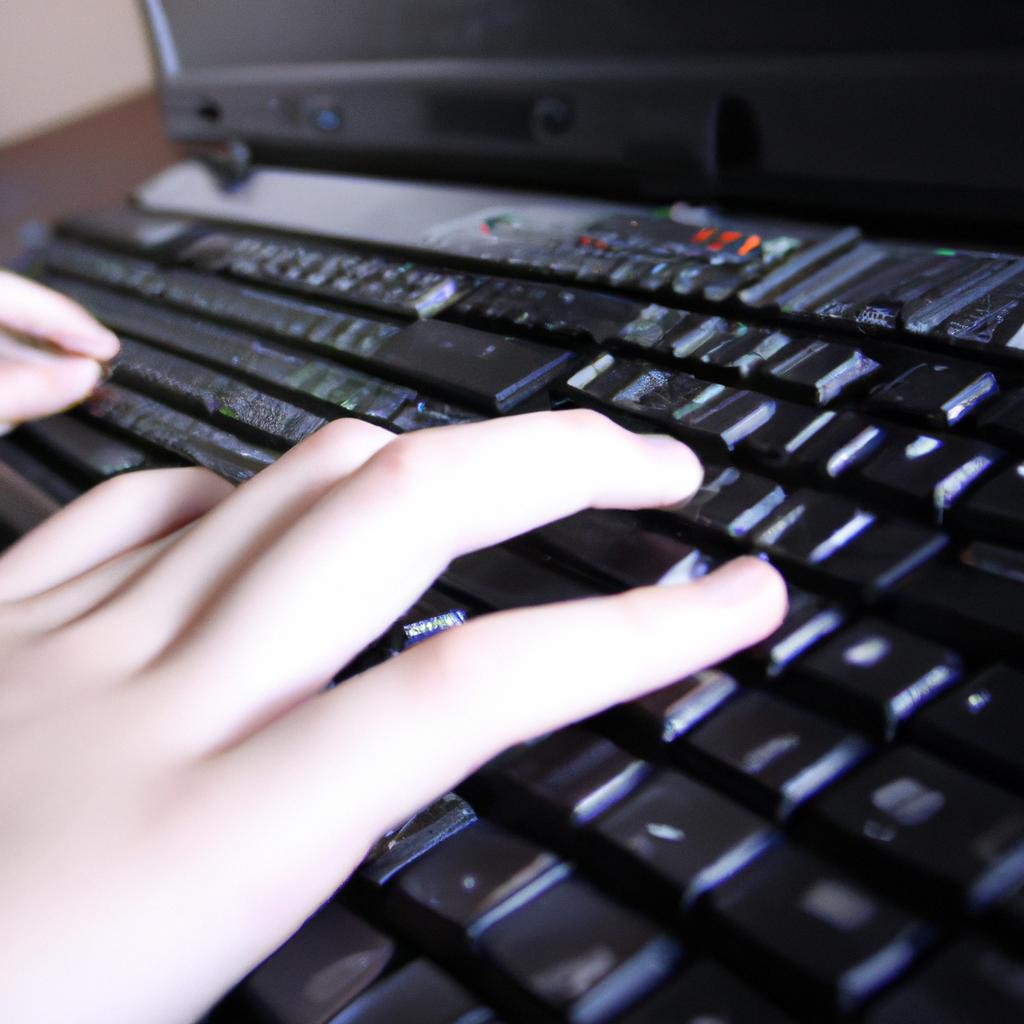
The usage of functions is an essential aspect of computer programming languages, allowing programmers to organize and structure their code efficiently. Among the various types of functions, anonymous functions stand out as a powerful tool that offers flexibility and convenience in certain scenarios. An anonymous function, also known as a lambda function or nameless function, does not have a specific identifier associated with it and can be defined inline within other expressions or passed as arguments to other functions.
To illustrate the significance of anonymous functions, let us consider a hypothetical scenario where a software developer is tasked with creating a program that sorts a list of student grades in ascending order. Traditionally, this task would require defining a separate named function for sorting purposes. However, by utilizing anonymous functions, the programmer can create concise and efficient code without cluttering the overall structure of the program. This example highlights how understanding and effectively employing anonymous functions can greatly enhance the development process in computer programming languages. In this article, we will delve into the concept of anonymous functions in-depth, exploring their syntax, applications, advantages, and potential challenges faced when working with them. By gaining insight into these aspects, readers will gain a comprehensive understanding of how to leverage the power of anonymous functions in their own programming endeavors.
Understanding Anonymous Functions
Anonymous functions, also known as lambda functions or nameless functions, are an essential aspect of computer programming languages. These functions play a crucial role in enhancing code readability and flexibility by allowing developers to define and use functions without explicitly naming them. To better grasp the concept of anonymous functions, consider the following example:
Imagine you are working on a project that requires sorting a list of names alphabetically. Traditionally, one would create a separate function named “sort_names” and call it whenever needed. However, with anonymous functions, you can achieve the same result more concisely and elegantly.
To illustrate this further, let us explore some key characteristics of anonymous functions:
- Flexibility: Unlike regular named functions, which require declaration before usage, anonymous functions can be defined at runtime and used immediately.
- Code Readability: By eliminating the need for explicit function declarations, anonymous functions allow programmers to focus on the logic directly within their code blocks.
- Encapsulation: Anonymous functions encapsulate small pieces of functionality into reusable units while avoiding cluttering the main code structure.
- Scope Management: With anonymous functions, variables from outer scopes can be accessed seamlessly within their scope.
Flexibility | Code Readability | Encapsulation | Scope Management |
---|---|---|---|
Enhances | Simplifies | Promotes | Facilitates |
development process | understanding | modular coding approach | access to parent scopes |
In conclusion,
Moving forward into our exploration of the benefits of using anonymous functions will shed light on how they contribute to efficient coding practices and improved program performance.
Benefits of Using Anonymous Functions
In the previous section, we explored the concept of anonymous functions and their significance in computer programming languages. Now, let us delve deeper into the benefits that arise from using these powerful tools.
To illustrate the practicality of anonymous functions, consider a scenario where you are developing a web application that requires sorting user data based on different criteria such as name, age, or registration date. By utilizing anonymous functions, you can easily create custom sorting algorithms specific to each criterion without having to write separate named functions for each case. This flexibility allows for more efficient code organization and promotes reusability.
One significant benefit of anonymous functions is their ability to enhance code readability and maintainability. The use of concise inline expressions reduces clutter and makes it easier to understand the purpose of certain operations within larger code blocks. Moreover, since these functions are defined locally within another function or expression block, they do not pollute the global namespace with unnecessary identifiers. This encapsulation helps prevent naming conflicts and improves overall code quality.
Anonymous functions also enable developers to implement higher-order functionality by treating them as first-class citizens. They can be assigned to variables, passed as arguments to other functions, or returned as results from function calls. This versatility empowers programmers to employ functional programming paradigms effectively, enabling cleaner and more expressive code solutions.
- Increased efficiency through custom sorting algorithms.
- Enhanced readability leading to better comprehension.
- Improved maintainability due to reduced clutter.
- Empowerment for functional programming practices.
Benefit | Description |
---|---|
Increased Efficiency | Custom sorting algorithms streamline data processing tasks |
Enhanced Readability | Concise code structure facilitates understanding |
Improved Maintainability | Reduced clutter enhances ease of maintenance |
Empowered Functional Programming Practices | Flexibility for adopting functional programming concepts |
As we have seen, embracing anonymous functions brings numerous advantages to computer programming. Their flexibility, improved code organization, and support for functional programming paradigms make them a valuable tool in the developer’s toolbox. In the subsequent section, we will explore common use cases where anonymous functions shine and further highlight their practicality in real-world scenarios.
Common Use Cases for Anonymous Functions
Example:
To better understand the practical implications of using anonymous functions, let’s consider a hypothetical scenario in which you are developing a web application that requires sorting an array of objects based on different criteria. Instead of writing separate named functions for each sorting criterion, you can utilize anonymous functions to create dynamic and reusable code.
Implementation considerations for anonymous functions can vary depending on the programming language and specific use case. Here are some key factors to keep in mind:
-
Scope Management:
Since anonymous functions do not have their own names, it is important to handle scope correctly when utilizing them within larger programs. Properly managing variables and closures ensures that the anonymous function has access to all necessary data without causing conflicts or memory leaks. -
Readability and Maintainability:
While anonymous functions can offer succinct solutions, they may also introduce complexity if used excessively or improperly formatted. Balancing conciseness with readability is crucial for maintaining code quality and facilitating future modifications or debugging efforts. -
Performance Optimization:
Anonymous functions generally incur a slight performance overhead due to their dynamic nature compared to their named counterparts. However, this impact is often negligible unless used extensively in computationally intensive tasks such as loops or recursion. Profiling tools can help identify potential bottlenecks and optimize critical sections where required. -
Error Handling:
When errors occur within an anonymous function, proper error handling becomes essential for effective debugging and graceful program execution flow. Ensuring robust exception management helps catch any unexpected issues within these functions promptly and provides meaningful feedback to aid troubleshooting processes.
- Enhances flexibility by allowing dynamic implementation
- Promotes code reuse through concise expressions
- Increases developer productivity by reducing boilerplate code
- Enables more elegant solutions by supporting higher-order programming techniques
Pros | Cons |
---|---|
Code reusability | Potential readability issues |
Concise syntax | Slight performance overhead |
Dynamic implementation | Scope management challenges |
Supports higher-order programming techniques | Potential error handling complexities |
In the context of anonymous functions, understanding these implementation considerations is crucial for leveraging their power effectively. In the subsequent section, we will explore the syntax and provide examples to further illustrate how anonymous functions can be utilized in different programming languages.
With a solid foundation on the implementation aspects of anonymous functions, let’s now delve into their syntax and examine practical code snippets to better comprehend their usage.
Syntax and Examples of Anonymous Functions
Transitioning from the previous section, where we explored common use cases for anonymous functions, let us now delve into the syntax and examples of these powerful tools. To illustrate their practicality, consider a hypothetical scenario wherein a software developer is creating a program that requires sorting an array of integers in ascending order.
The flexibility of anonymous functions allows developers to pass them as arguments to other functions or assign them directly to variables. This feature can greatly simplify code by eliminating the need to define separate named functions for specific operations. For instance, our developer could make use of an anonymous function within a built-in “sort” function, specifying the criteria for ordering the integers. By using this approach, they effectively eliminate the need for writing additional custom sorting functions, resulting in more concise and maintainable code.
To further emphasize the advantages offered by anonymous functions, here are some notable benefits worth considering:
- Code reusability: Anonymous functions allow programmers to reuse blocks of code without having to create separate named functions.
- Encapsulation: By encapsulating functionality within an anonymous function, it becomes easier to manage complex logic or perform tasks within restricted scopes.
- Flexibility: The ability to define and use anonymous functions on-the-fly provides developers with greater flexibility when solving problems or implementing specific algorithms.
- Improved readability: When used judiciously, incorporating well-named anonymous functions can enhance code readability and make it more intuitive.
Advantages of Anonymous Functions |
---|
Code Reusability |
Encapsulation |
Flexibility |
Improved Readability |
In conclusion, understanding how to utilize anonymous functions enables programmers to write more efficient and elegant code. They offer numerous benefits such as improved code reusability, encapsulation of functionality, increased flexibility, and enhanced readability. By leveraging these advantages, developers can create programs that are easier to maintain and modify as needed.
Now that we have explored the syntax and benefits of anonymous functions, let us examine the differences between anonymous functions and named functions in more detail.
Differences between Anonymous Functions and Named Functions
Section 3: Use Cases and Benefits of Anonymous Functions
Consider a scenario where you are developing a web application that requires sorting an array of user data based on different criteria such as age, name, or location. In this case, you can use anonymous functions to create custom sorting algorithms without the need for defining separate named functions for each criterion. This flexibility allows you to easily adapt your code to various requirements.
One of the key benefits of using anonymous functions is their ability to improve code readability and maintainability. By encapsulating smaller blocks of logic within anonymous functions, the main code becomes more concise and easier to understand. Additionally, these encapsulated functions can be reused in different parts of the program without cluttering up the namespace with unnecessary function declarations.
Using anonymous functions provides several advantages:
- Flexibility: The dynamic nature of anonymous functions enables developers to easily modify behavior at runtime by passing them as arguments or assigning them to variables.
- Closures: Anonymous functions have access to variables defined in their surrounding scope, even after those variables go out of scope. This concept known as closures helps prevent data leakage and improves memory efficiency.
- Event handling: Anonymous functions are commonly used in event-driven programming paradigms for handling events triggered by user actions like button clicks or mouse movements.
- Asynchronous operations: When working with asynchronous tasks such as making API calls or performing calculations in separate threads, anonymous functions allow developers to define callback behaviors inline instead of creating additional named function definitions.
To further illustrate the usefulness of anonymous functions, consider the following table showcasing some common scenarios where they are commonly employed:
Scenario | Description |
---|---|
Sorting Algorithms | Custom sort order based on varying criteria |
Filtering Data | Selecting specific elements from a collection based on conditions |
Callback Functions | Executing specific logic upon completion of asynchronous tasks |
Event Listeners | Handling user interactions such as clicks, keypresses, or form submissions |
In summary, anonymous functions serve as powerful tools in computer programming languages. They offer flexibility, improve code readability and maintainability, enable dynamic behavior modification, facilitate event handling and asynchronous operations.
Next Section: Best Practices for Working with Anonymous Functions
Best Practices for Working with Anonymous Functions
In the previous section, we examined the distinctions between anonymous functions and named functions. Now, let us delve further into the concept of anonymous functions and explore some best practices for working with them in computer programming languages.
To provide a practical example, consider a scenario where you are developing a web application that requires sorting an array of user names alphabetically. With anonymous functions, you can easily accomplish this task by using higher-order functions like Array.prototype.sort()
in JavaScript or similar constructs in other programming languages. By passing an anonymous function as an argument to sort()
, you can define custom sorting logic without explicitly declaring a separate named function.
When working with anonymous functions, it is essential to keep certain best practices in mind:
- Code readability: While anonymity provides flexibility, it should not compromise code clarity. Ensure that your anonymous functions have clear and concise logic so that fellow developers can understand their purpose.
- Modularity: Break down complex tasks into smaller, reusable units by encapsulating related functionality within individual anonymous functions. This promotes modularity and enhances code maintainability.
- Scoping considerations: Understand how scoping works when dealing with variables inside anonymous functions. Be cautious about variable hoisting and closures to avoid unintended side effects.
- Testing and debugging: Anonymous functions can sometimes make testing and debugging more challenging due to their lack of explicit naming. Consider providing descriptive comments or leveraging debugging tools to aid in these processes.
Pros | Cons |
---|---|
Encourages modular approach | Reduced reusability |
Cleaner syntax | Difficulty in error tracing |
Enhanced code organization | Limited visibility |
By adhering to these best practices, programmers can leverage the power of anonymous functions effectively while minimizing potential drawbacks. Incorporating such guidelines ensures maintainable codebases that facilitate collaborative development efforts across various programming languages and paradigms.
In summary, anonymous functions offer a flexible approach for encapsulating logic within computer programming languages. By understanding their distinctions from named functions and following best practices, developers can harness the benefits of anonymity while ensuring code readability, modularity, scoping considerations, and effective testing and debugging techniques.