Control Structures: Syntax of Computer Programming Languages
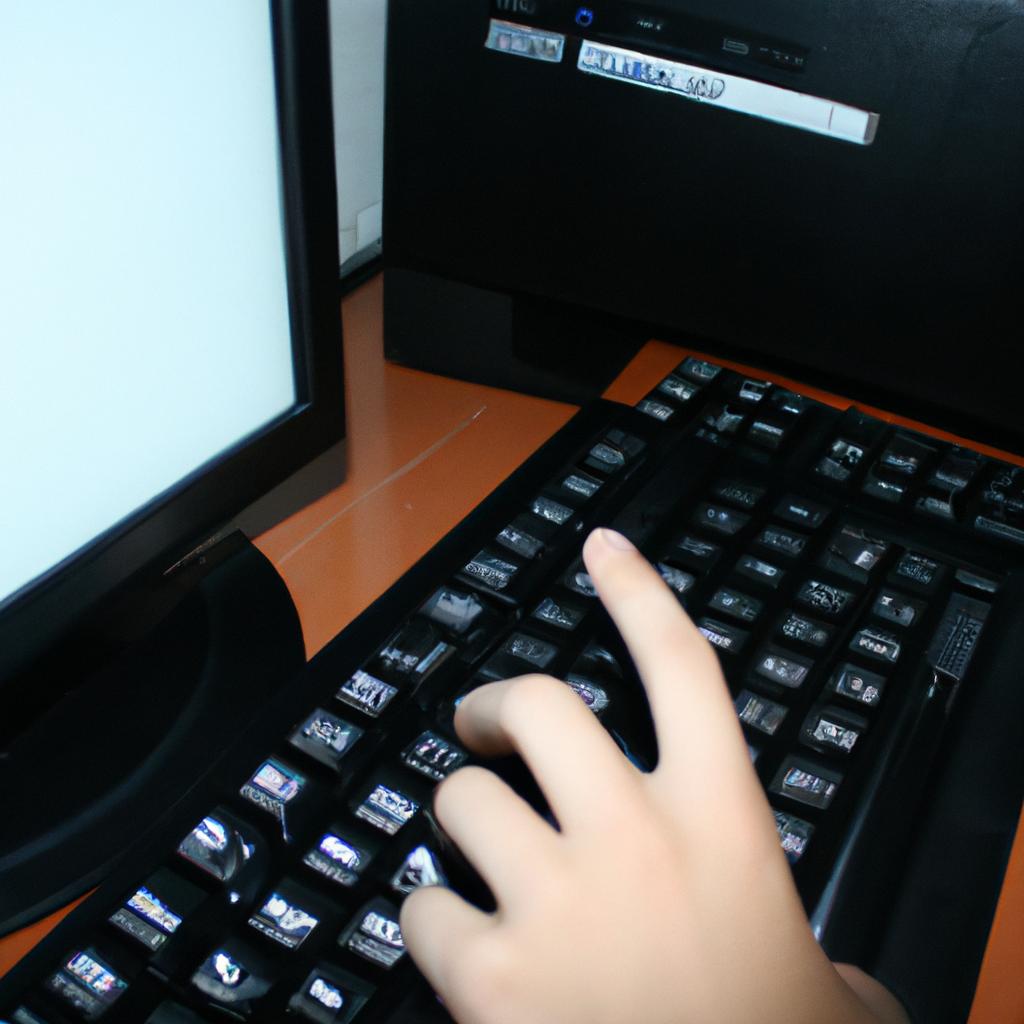
Control structures are an essential component of computer programming languages, dictating the flow and execution of instructions within a program. These structures enable programmers to create complex algorithms and logic by providing mechanisms for decision-making, iteration, and branching. For instance, consider a scenario where a software developer is tasked with designing a program that simulates a traffic light system at an intersection. The control structures in the programming language would allow the programmer to define how the lights change based on specific conditions such as time intervals or sensor inputs.
In computer programming, syntax refers to the set of rules that dictate how code should be written in order to be correctly understood and executed by a computer. Control structures form an integral part of this syntax, enabling programmers to effectively communicate their intentions through logical constructs. By utilizing control structures such as if-else statements, loops, and switch-case statements, programmers can manipulate the flow of execution within their programs based on certain conditions or criteria. This article aims to explore various types of control structures found in different programming languages, highlighting their syntax differences and discussing best practices for their usage. Furthermore, it will delve into common pitfalls associated with control structure implementation and provide guidelines for writing clean and efficient code using these constructs.
Decision Making Structures
One of the fundamental concepts in computer programming languages is decision making structures. These structures allow programmers to control the flow of execution based on certain conditions or criteria. For example, consider a program that calculates whether a student has passed an exam or not. By using decision making structures, the program can evaluate the student’s score and determine if it meets the passing threshold.
To better understand decision making structures, let us examine some key characteristics:
- Condition: A condition is a logical expression that evaluates to either true or false. It serves as the basis for deciding which path of execution should be followed.
- Branching: Depending on the outcome of the condition evaluation, different paths of execution are taken. This branching mechanism allows programs to dynamically adapt their behavior.
- Multiple Conditions: Decision making structures also support multiple conditions by utilizing logical operators such as AND and OR. This enables more complex decision-making scenarios.
- Default Path: In cases where none of the specified conditions are met, a default path can be defined to handle any exceptional situations.
To illustrate these concepts further, consider the following table representing a simplified decision-making process for determining eligibility for a scholarship:
Criteria | Eligible | Not Eligible |
---|---|---|
GPA | 3.5 or above | Below 3.5 |
Extracurriculars | Active participant | No involvement |
Financial Need | Demonstrated | Not demonstrated |
By evaluating each criterion against specific thresholds, this decision-making structure determines whether an applicant is eligible for a scholarship or not. The use of tables like this enhances readability and helps visualize complex logic in programming.
In summary, decision making structures play a crucial role in computer programming languages by allowing programs to make choices based on predefined conditions. They enable programmers to create dynamic and adaptive applications by controlling the flow of execution through various branches. Understanding these structures and their characteristics is essential for developing efficient and robust software solutions.
Moving forward, let us explore another important aspect of control structures: looping structures. These structures provide the ability to repeat a set of instructions multiple times, further enhancing the flexibility and power of programming languages.
Looping Structures
By utilizing these constructs, programmers can repeat a set of instructions multiple times until a certain condition is met. This allows for more efficient and flexible programming, as complex tasks can be automated and executed with ease.
Looping structures are particularly useful when dealing with repetitive tasks or data processing that requires iteration. For instance, consider a scenario where a software engineer needs to analyze large datasets containing customer information. Using a loop structure, they can iterate over each record in the dataset and perform specific calculations or operations on them. This not only saves time but also ensures accuracy and consistency throughout the process.
To better understand the mechanics of looping structures, let’s explore their key characteristics:
- Iteration control variable: A variable that keeps track of the current iteration number.
- Loop condition: An expression that determines whether to continue iterating based on specified conditions.
- Loop body: A block of code enclosed within curly braces that contains the instructions to be repeated.
- Loop increment/decrement: Specifying how the iteration control variable should change after each iteration.
Characteristic | Description |
---|---|
Iteration Control Variable | Keeps track of the current iteration number |
Loop Condition | Determines whether to continue iterating |
Loop Body | Contains instructions to be repeated |
Loop Increment/Decrement | Specifies how the iteration control variable changes |
Using looping structures enhances both efficiency and flexibility in computer programming by automating repetitive tasks and streamlining data processing. These constructs allow developers to save time and ensure accurate execution while handling large datasets or performing iterative operations. In our subsequent section about “Conditional Statements,” we will discuss another fundamental aspect of control structures that complements looping structures seamlessly.
Moving forward from understanding looping structures, we now shift our focus to conditional statements. These constructs enable programmers to make decisions based on certain conditions, allowing for dynamic and adaptable program execution. By incorporating conditional statements into their code, developers can create logic pathways that respond intelligently to varying inputs or situations without the need for constant manual intervention.
Conditional Statements
Building upon the concept of looping structures, we now delve into the syntax and functionality of conditional statements. By employing these control structures in computer programming languages, developers can execute specific sets of code based on certain conditions. This section will explore how conditional statements allow for decision-making within programs.
Conditional statements enable programmers to create branches in their code, where different paths are followed depending on whether a condition is true or false. To illustrate this, let’s consider a hypothetical scenario involving an e-commerce website. When a customer adds items to their shopping cart, the program needs to determine if they qualify for any discounts based on factors such as purchase amount or membership status. If the customer qualifies for a discount, the program would apply it; otherwise, it would proceed with regular pricing calculations.
To implement conditional logic effectively, programmers utilize various constructs commonly found in most programming languages:
- IF statement: This construct checks if a given condition is true and executes a block of code only if the condition evaluates to true.
- ELSE statement: Used in conjunction with an IF statement, this construct provides an alternative block of code that is executed when the initial condition is not met.
- ELSE IF statement (also known as ELIF or ELSE IF ladder): Similar to an IF statement but allows multiple conditions to be evaluated sequentially until one matches.
- SWITCH statement: Designed specifically for multi-way selections, this construct enables efficient execution of different blocks of code based on distinct values or cases.
- Empowers developers by allowing them to make dynamic decisions within their programs
- Enhances user experience through personalized interactions and tailored responses
- Improves efficiency by eliminating unnecessary computations or actions
- Enables error handling and exception management
Table showcasing examples:
Condition | Action |
---|---|
User logged in | Display personalized content |
Purchase total > $50 | Apply 10% discount |
Item quantity > 10 | Display “Bulk discount” |
Age < 18 | Restrict access to content |
As programmers gain proficiency in using conditional statements, they unlock the ability to create more sophisticated and interactive programs. The next section will explore another crucial type of control structure known as iteration statements, which allows for repeating a set of code multiple times until a specified condition is met or no longer holds true.
Now turning our attention to iteration statements…
Iteration Statements
From the previous section on conditional statements, we now delve into the world of iteration statements. Imagine a scenario where you are tasked with developing a program that calculates the average grade for a class of students. As you begin writing the code, you realize that manually inputting each student’s grade would be time-consuming and impractical. Here is where iteration statements come to your rescue.
Iteration statements, also known as loops, allow us to repeat a block of code multiple times until a certain condition is met or for a specified number of iterations. Let us consider an example using a hypothetical grading system: You have stored the grades of 50 students in an array named “grades.” To calculate their average, instead of individually accessing each element in the array, you can use an iteration statement such as a “for” loop to iterate through all the elements and sum them up.
To better understand how iteration statements work, let us explore some key concepts:
- Initialization: Before entering the loop, you initialize any required variables or conditions.
- Condition: The loop executes repeatedly until this condition evaluates to false.
- Increment/Decrement: After executing each iteration within the loop body, there might be an increment or decrement operation performed on one or more variables.
Now let’s look at how iteration statements can enhance our understanding by considering four key benefits they provide:
- Efficiency: By automating repetitive tasks, iterations save valuable time and effort.
- Scalability: Iteration statements allow programs to handle large amounts of data without manual intervention.
- Flexibility: With different types of loops available (such as for loops and while loops), programmers can choose the most suitable option for their specific needs.
- Error Prevention: Using loops helps minimize human error caused by manual repetition.
In addition to these points, it is worth noting that iteration statements play a crucial role in controlling flow within computer programs. In our subsequent section on control flow, we will explore how both conditional and iteration statements work together to create dynamic programs that respond intelligently to different scenarios. So now, let us proceed with an in-depth exploration of control flow within computer programming languages.
Control Flow
Iteration Statements allow for the repetition of a block of code based on certain conditions. These statements are an important part of control structures in computer programming languages, as they enable developers to execute specific instructions multiple times without having to manually repeat them. To better understand how iteration statements work, let’s consider a hypothetical example.
Imagine you are developing a program that calculates the average grade of students in a class. You have stored all the grades in an array called “grades.” Using an iteration statement such as a “for” loop, you can iterate through each grade in the array and calculate their sum. Once you have obtained the sum, you can divide it by the total number of grades to find the average.
When working with iteration statements, there are several key points to keep in mind:
- Termination condition: A termination condition determines when the iteration should stop. It is crucial to specify this condition correctly; otherwise, your program may enter an infinite loop.
- Loop variable: The loop variable is used to track the progress of each iteration. It is typically initialized before entering the loop and updated after every iteration.
- Control flow: Control flow refers to the order in which statements are executed within a program. Within an iteration statement, control flow jumps back to the beginning if the termination condition has not been met yet.
- Nested iterations: Iteration statements can be nested inside one another, allowing for more complex repetitive tasks. However, care must be taken while implementing nested loops to ensure efficient execution and prevent potential errors.
Terminology | Description |
---|---|
Termination Condition | Determines when the iteration should stop |
Loop Variable | Tracks progression during each iteration |
Control Flow | Order in which statements are executed |
Nested Iterations | Multiple iterations within one another |
In summary, understanding and utilizing iteration statements effectively is essential for controlling repetitive tasks in computer programming languages. By specifying a termination condition, using a loop variable to track progress, understanding control flow, and implementing nested iterations when necessary, developers can achieve efficient execution of repetitive code blocks.
Execution Order
Control Structures: Syntax of Computer Programming Languages
Transitioning from the previous section on control flow, let us now delve into the syntax of computer programming languages. To better understand how control structures shape the execution of programs, consider the following example: Suppose you are developing a weather application that provides real-time updates to users. Depending on their location and preferences, different parts of your code will be executed to retrieve relevant data and display it in a user-friendly manner.
Control structures play a vital role in directing the flow of program execution by determining which sections of code should be executed under specific conditions. They enable programmers to make decisions, iterate over collections of data, and execute certain blocks of code repeatedly until a given condition is met. Here are some key points regarding control structures:
- Conditional Statements: These allow for decision-making within programs by evaluating logical expressions and executing different sets of instructions based on their truth value.
- Loops: Iteration is made possible through loops, which enable repetitive execution of a block of code until a specified condition is no longer satisfied.
- Branching Statements: These statements provide mechanisms for altering normal program flow by jumping to specific locations within the code or terminating its execution altogether.
- Exception Handling: Control structures also facilitate error handling by allowing developers to catch and handle exceptions gracefully, preventing abrupt termination of the program.
To illustrate these concepts further, consider the table below showcasing examples of control structures commonly found in various programming languages:
Language | Conditional Statement Example | Loop Example | Branching Statement Example |
---|---|---|---|
Python | if temperature > 30: |
for i in range(5): |
break if count >= maxCount |
JavaScript | if (age < 18) |
while(i<10) |
return false; |
Java | if (score >= passingScore) |
for(int i=0; i<5; i++) |
System.exit(1); |
C++ | if (num % 2 == 0) |
do { ... } while(condition) |
goto end; |
In conclusion, control structures serve as the building blocks of program flow and execution in computer programming languages. By incorporating conditional statements, loops, branching statements, and exception handling mechanisms, developers can create programs that respond intelligently to different scenarios. Understanding the syntax and utilization of these constructs is crucial for effectively implementing algorithms and solving complex problems through code.