Syntax: The Mechanics of Computer Programming Languages
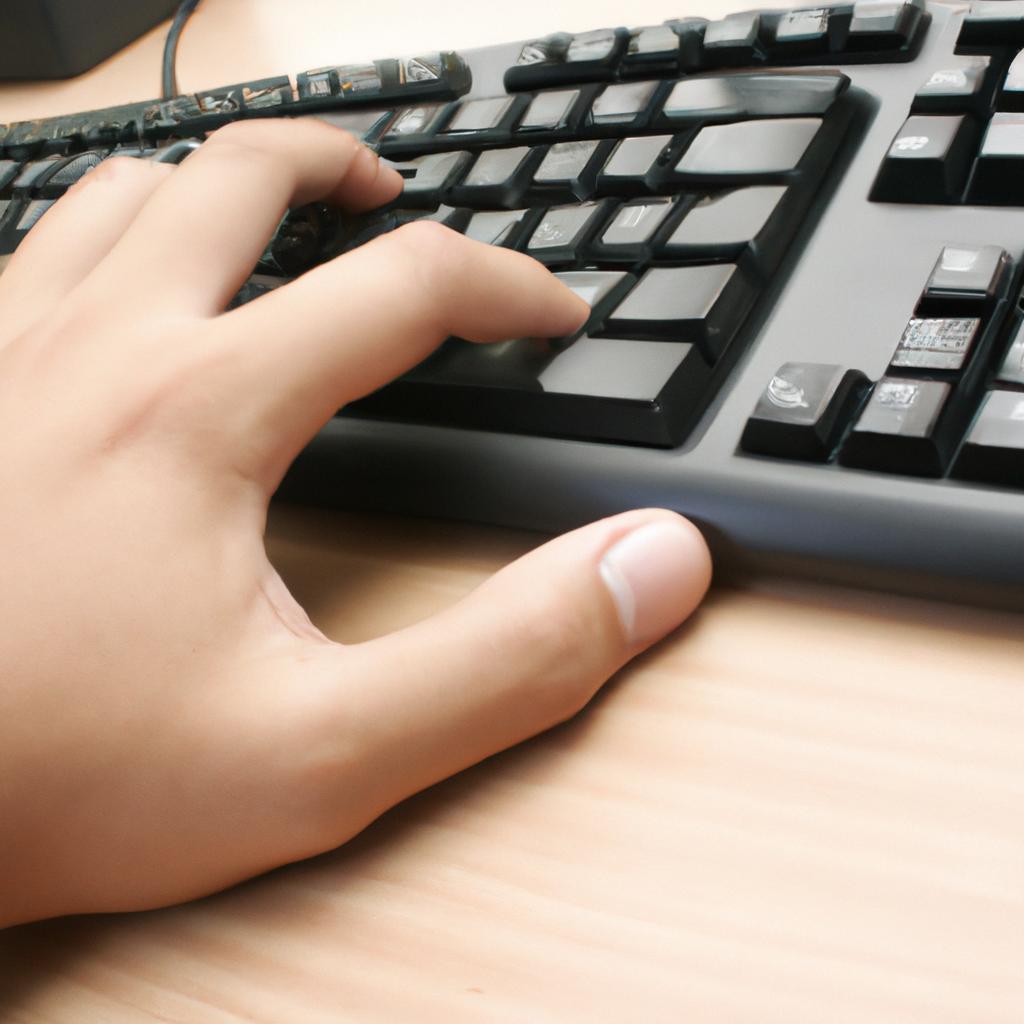
Syntax: The Mechanics of Computer Programming Languages
One cannot deny the fundamental role that syntax plays in computer programming languages. It is like a set of rules and guidelines that govern how programmers write their code, ensuring that it is both understandable to humans and executable by machines. Just as proper grammar allows us to effectively communicate our thoughts in natural language, mastering syntax empowers programmers to express complex algorithms and logical structures within the confines of a programming language.
To illustrate this point, let us consider a hypothetical scenario involving two novice programmers attempting to create a simple calculator program using different programming languages. Programmer A decides to use Python, while Programmer B opts for Java. Despite their similar logic and intention behind creating the calculator, they encounter various challenges due to differences in syntax between these two languages. While Programmer A effortlessly utilizes indentation and colons in Python to structure the code blocks, Programmer B struggles with semicolons and curly brackets required by Java. This example highlights how understanding and adhering to syntax principles directly impacts the readability and functionality of a program, regardless of its complexity or purpose.
In this article, we will delve into the intricate world of syntax in computer programming languages. We will explore its significance, examine common syntactical elements found across different programming languages, discuss best practices for writing clean and maintainable code, and provide tips for effectively troubleshooting syntax errors. By the end of this article, you will have a deeper understanding of how syntax influences the structure and behavior of computer programs, enabling you to become a more proficient programmer.
Firstly, let us understand what syntax actually refers to in the context of programming languages. Syntax encompasses the rules and conventions that dictate the correct arrangement and usage of symbols, keywords, and expressions within a programming language. These rules define the grammar of the language – just as sentences follow grammatical rules in natural languages, code must adhere to syntactical rules in order to be interpreted correctly by computers.
Each programming language has its own unique syntax, which may vary significantly from one another. However, there are also common syntactical elements that can be found across multiple languages. These include:
- Variables: Used to store and manipulate data values.
- Functions: Blocks of reusable code that perform specific tasks.
- Control structures: Constructs like conditionals (if-else statements) and loops (for loops, while loops) used to control program flow.
- Operators: Symbols or words that perform operations on data (e.g., arithmetic operators like +,-,*).
- Comments: Text annotations within code that are ignored by compilers or interpreters but serve as notes for programmers.
Understanding these common elements is crucial in learning new programming languages because they form the building blocks upon which more complex programs are constructed.
To write code that is both readable and maintainable, it is essential to follow best practices when it comes to syntax. Here are some tips:
- Consistency: Stick to a consistent style throughout your codebase by following established conventions such as indentation patterns and naming conventions.
- Clarity: Write clear and descriptive variable names, function names, and comments to make your code easier for others (and your future self) to understand.
- Organization: Use whitespace and proper indentation to structure your code into logical blocks, making it more readable and maintainable.
- Documentation: Document your code by adding comments or using tools like docstrings to explain the purpose and usage of functions, classes, and modules.
Despite our best efforts, syntax errors are a common occurrence when writing code. When faced with such errors, it is important to effectively troubleshoot them. Here are some strategies:
- Understand error messages: Carefully read the error message provided by the compiler or interpreter, as it often provides valuable clues about what went wrong.
- Review recent changes: If you recently made modifications to your code, focus on those areas first as they are likely the source of the error.
- Check for missing or misplaced symbols: Syntax errors often occur due to missing parentheses, quotation marks, or other symbols that disrupt the expected structure of the code.
- Use an integrated development environment (IDE): IDEs often include features like automatic syntax highlighting and real-time error checking that can help identify and fix syntax errors quickly.
In conclusion, understanding syntax is a fundamental aspect of computer programming languages. By following syntactical rules and best practices, programmers can create clean and maintainable code that is both understandable to humans and executable by machines. Through studying common syntactical elements and effective troubleshooting techniques, programmers can become proficient in multiple programming languages and tackle increasingly complex programming challenges.
Foundations of Programming
Programming languages serve as the foundation for computer programs, enabling developers to communicate instructions effectively with computers. Understanding the syntax—the mechanics of programming languages—is essential for success in this field. To illustrate its significance, consider a hypothetical scenario where a programmer is tasked with developing a weather application. By utilizing syntax rules, they can write code that fetches real-time weather data from an API and presents it to users in a user-friendly format.
To grasp the importance of mastering programming language syntax, let us explore four key reasons why it plays such a critical role:
- Precision: Syntax enforces precision by defining how commands must be structured within a program. Just like grammar governs sentence structure in spoken language, syntax serves as the rulebook for writing correct code.
- Clarity: Properly following syntax guidelines enhances code readability, making it easier for programmers and collaborators to understand what each line of code does.
- Efficiency: Adhering to syntactic conventions allows compilers or interpreters—software responsible for translating human-readable code into machine-executable instructions—to process programs more efficiently and accurately.
- Consistency: A consistent coding style ensures that different parts of a program look and function similarly throughout, promoting maintainability and reducing errors caused by inconsistencies.
Consider the following table highlighting some commonly used programming languages along with their respective paradigms:
Language | Paradigm | Example Use Case |
---|---|---|
Python | Object-oriented | Data analysis and scientific computing |
C | Procedural | Operating systems development |
JavaScript | Event-driven | Web applications |
Haskell | Functional | Mathematical computations |
In summary, understanding the foundations of programming involves grasping the intricacies of syntax within various programming languages. Precise adherence to these rules not only ensures accurate communication with computers but also enhances code clarity, efficiency, and consistency.
Transitioning seamlessly from syntax to the subsequent section on “Building Blocks of Code,” we can now explore how these foundational elements come together to form functional programs.
Building Blocks of Code
Building upon the foundational concepts covered in the previous section, let us delve into the essential components that form the building blocks of code. By understanding these fundamental elements, programmers can construct robust and efficient programs. To illustrate this, consider a hypothetical scenario where a software developer is tasked with creating a program to sort a large dataset containing customer information.
The first crucial aspect to comprehend when working with programming languages is variables. Variables act as containers for storing data values that may change during program execution. In our case study, the developer might create variables such as “name,” “age,” and “address” to hold different pieces of customer information. By assigning values to these variables, the programmer can manipulate and access specific data points within their application.
Another vital concept in coding is control structures. These structures enable programmers to dictate how instructions are executed based on certain conditions or criteria. For instance, using an if-else statement in our example, the developer could implement logic that checks if customers are above a specified age threshold before including them in further processing steps. Control structures provide flexibility and decision-making capabilities within programs, enhancing their overall functionality.
In addition to variables and control structures, functions play a critical role in organizing code and promoting reusability. Functions encapsulate sets of instructions that perform specific tasks, allowing developers to modularize their codebase effectively. Our software engineer could define a function called “sortCustomers” that takes in the dataset as input and implements algorithms like bubble sort or quicksort to arrange customer records according to predefined criteria. Employing functions improves readability, reduces redundancy, and simplifies complex programming tasks.
To summarize:
- Variables store changing data values.
- Control structures determine how instructions are executed based on conditions.
- Functions organize code into reusable units.
Markdown bullet point list:
- Variables: Store changing data values
- Control Structures: Determine instruction execution based on conditions
- Functions: Organize code into reusable units
- Case Study: Sorting a large dataset of customer information
Concept | Purpose | Example |
---|---|---|
Variables | Store and manipulate changing data values | name = "John Doe" |
Control Structures | Determine the flow of execution based on criteria | if age >= 18 |
Functions | Encapsulate sets of instructions for reusability | def sortCustomers(data): |
Moving forward, we will explore the logical structures used in programming languages. These structures provide programmers with powerful tools to control program flow and make decisions based on various conditions. By understanding these concepts, developers can construct programs that efficiently solve complex problems.
Logical Structures in Programming
Transitioning from the previous section on “Building Blocks of Code,” we now delve into the fundamental aspect of syntax, which governs how code is written in programming languages. Understanding syntax is crucial for developers as it ensures that computer programs are structured correctly and can be executed accurately. To illustrate this point, let’s consider a hypothetical scenario where a programmer attempts to write a line of code without adhering to proper syntax:
For instance, imagine a novice developer attempting to define a variable but mistakenly omitting the semicolon at the end. As a result, when running the program, they encounter an error message indicating that there is a syntax error. This example highlights the significance of syntax in coding; even small mistakes can lead to significant issues.
To better comprehend syntax and its role in programming languages, let us explore some key aspects:
- Structure: Syntax provides guidelines for organizing code elements such as statements, expressions, functions, and declarations. It defines how these components should be arranged within the program.
- Symbols and Operators: Programming languages utilize various symbols and operators (e.g., +,-,/,*), each carrying specific meanings defined by their respective language’s syntax rules.
- Keywords: Languages have reserved keywords with predetermined meanings used for control flow statements like loops or conditional statements.
- Delimiters: These special characters such as parentheses (), brackets [], or curly braces {} signify the beginning or end of certain code blocks.
Understanding these foundational elements allows programmers to effectively communicate instructions to computers through well-formed code structures. To further elucidate the importance of mastering syntax concepts, consider the following table showcasing common errors caused by violating language-specific syntactical rules:
Error Type | Cause | Example |
---|---|---|
Missing Semicolon | Failure to terminate statement | int x = 5 |
Mismatched Brackets | Inconsistent bracket placement | if (x > 5 { return x; |
Undefined Variable | Use of a variable not declared | cout << y; |
Syntax typos | Misspelling or misplacement | for(i=0;i<10,i++) {... |
By familiarizing oneself with these syntactical errors, developers can avoid common pitfalls and enhance their code’s overall quality.
Moving forward, we will explore another vital aspect of programming – making decisions in code. This involves utilizing logical structures to create programs that respond dynamically based on specific conditions. By incorporating decisions into our code, we unlock the potential for building more intelligent and interactive software systems.
Making Decisions in Code
Transitioning from the logical structures in programming, let us now delve into an essential aspect of computer programming languages—controlling program flow through loops. To illustrate this concept, consider a hypothetical scenario where you are tasked with creating a program to calculate and display the sum of all numbers between 1 and 100. Without using loops, you would need to write out each number individually—a tedious and time-consuming task. However, by utilizing loops, you can automate this process and achieve the desired outcome efficiently.
Loops allow programmers to repeat a specific set of instructions multiple times until a certain condition is met or for a predetermined number of iterations. By incorporating loops into your code, you can reduce redundancy and increase efficiency. There are several types of loops commonly used in programming languages:
- For loop: A loop that iterates over a sequence of values based on specified conditions.
- While loop: Executes as long as a given condition evaluates to true.
- Do-while loop: Similar to the while loop but guarantees at least one execution before checking the condition again.
- Nested loop: A loop within another loop, allowing for more complex repetitive operations.
To better understand how Controlling Program Flow through loops works, we can visualize it using a table:
Iteration | Current Value | Sum |
---|---|---|
1 | 1 | 1 |
2 | 2 | 3 |
… | … | … |
As shown above, each iteration updates both the current value being processed and the cumulative sum. The repetition continues until all the necessary iterations have been completed.
Controlling program flow through loops is crucial for efficient coding practices. In our next section on “Controlling Program Flow,” we will explore additional techniques that enable programmers to further manipulate the execution of their code, enhancing its functionality and flexibility.
Controlling Program Flow
Building upon our understanding of programming logic, let us now delve into the intricate world of decision-making within code. By making use of conditional statements and logical operators, programmers can guide their programs to follow specific paths based on certain conditions. This ability is crucial for creating dynamic and interactive software applications.
In order to illustrate the concept of decision-making in code, consider a case study involving an e-commerce platform. When a customer places an order, the program needs to determine whether or not they qualify for free shipping based on their total purchase amount. If the purchase amount exceeds a predefined threshold, say $100, then free shipping will be provided; otherwise, regular shipping charges apply.
To effectively implement decision-making in code, programmers employ several key techniques:
- Conditional Statements: These statements evaluate a condition and execute different blocks of code depending on whether the condition is true or false.
- Comparison Operators: Used within conditional statements, these operators allow programmers to compare values and make decisions accordingly (e.g., equal to, greater than).
- Logical Operators: These operators enable complex decision-making by combining multiple conditions using logical AND, OR, and NOT operations.
- Nesting: By nesting conditional statements within one another, programmers can create sophisticated decision trees that handle various scenarios with precision.
Let’s visualize how this process works through a table:
Purchase Amount | Shipping Method |
---|---|
Less than $100 | Regular |
$100 or more | Free |
By employing these techniques and organizing them systematically using tables like the one above or bullet point lists as shown below, programmers can design robust algorithms that respond intelligently to varying circumstances:
- Decision-making allows programs to adapt dynamically based on changing inputs.
- It empowers developers to build user-friendly interfaces with personalized experiences.
- Error handling becomes more efficient when combined with appropriate decision-making mechanisms.
- Decision-making is critical for implementing complex algorithms and solving intricate problems.
As we move forward in our exploration of the mechanics of computer programming languages, let us now turn our attention to another fundamental aspect: manipulating data with operators. This next section will delve into how programmers utilize various operators to perform mathematical computations, manipulate strings, and modify variables within their code.
[Next Section: Controlling Program Flow]
Manipulating Data with Operators
By utilizing various control structures and statements, programmers can dictate how their programs execute different tasks based on specific conditions or user input. Now we will delve into the next important topic in programming languages: Manipulating Data with Operators.
To better understand the concept of manipulating data with operators, let’s consider an example scenario where we need to calculate the total cost of purchasing multiple items from an online store. Suppose we have a list of item prices stored in an array variable called prices
, and we want to find the sum of all these prices. In this case, we can use arithmetic operators, such as addition (+), to iterate through the array and accumulate the individual prices into a separate variable representing the total cost.
When it comes to manipulating data with operators, there are several key points to keep in mind:
- Operators: Programming languages provide various types of operators that allow us to perform operations on data values. These include arithmetic operators (e.g., + for addition), comparison operators (e.g., == for equality check), logical operators (e.g., && for logical AND), and assignment operators (e.g., = for assigning values).
- Precedence: Each operator has its own precedence level, which determines the order in which operations are executed when combining multiple operators within an expression. It is essential to be aware of operator precedence rules to ensure accurate computation.
- Data Types: Different types of data require different manipulations. For instance, mathematical operations like addition or subtraction work differently on numeric values compared to string concatenation or comparison between strings.
- Operator Overloading: Some programming languages support operator overloading, allowing developers to redefine how certain operators behave when applied to custom-defined objects or data types.
Operator Type | Example | Description |
---|---|---|
Arithmetic | +, -, *, / | Perform basic arithmetic calculations |
Comparison | ==, !=, > | Compare values for equality or order |
Logical | &&, || | Combine conditions and perform logical operations |
Assignment | =, += | Assign values to variables |
By understanding the concepts of manipulating data with operators, programmers can effectively manipulate and process different types of data in their programs. In the subsequent section on Handling Different Scenarios, we will explore how these programming language constructs come together to handle various scenarios and make decisions based on specific conditions encountered during program execution.
Handling Different Scenarios
In the previous section, we explored how data can be manipulated using various operators. Now, let us delve deeper into understanding how these operators work in different scenarios. To illustrate this concept, consider a hypothetical scenario where you are developing an e-commerce website that requires calculating discounts based on customer loyalty.
When a customer makes a purchase, their loyalty level determines the discount they receive. Let’s say there are three levels of loyalty – Bronze, Silver, and Gold. The discount percentages corresponding to each level are as follows:
- Bronze: 5% off
- Silver: 10% off
- Gold: 15% off
To implement this logic in your code effectively and ensure accurate calculations, it is crucial to understand the following points:
- Conditional Statements: Use conditional statements like if-else or switch-case to evaluate the customer’s loyalty level and determine the appropriate discount.
- Arithmetic Operators: Utilize arithmetic operators such as addition (+), subtraction (-), multiplication (*), and division (/) to calculate discounts based on percentages.
- Comparison Operators: Employ comparison operators like equal to (==), greater than (>), less than (<), etc., to compare values and make decisions within your program flow.
- Logical Operators: Combine multiple conditions using logical operators such as AND (&&) or OR (||) to create complex decision-making structures when determining discounts.
By mastering these concepts and employing them judiciously in your programming endeavors, you will be able to develop robust applications that handle diverse scenarios efficiently.
Next up is our exploration of “Using Conditional Statements.” This topic builds upon our understanding of manipulating data with operators by introducing conditional control structures that allow programs to make decisions based on specific conditions or criteria.
Using Conditional Statements
Transitioning from the previous section, where we explored various ways of handling different scenarios in programming, let us now delve into the powerful concept of conditional statements. Imagine you are designing a program that simulates an online shopping experience. To ensure a smooth user journey, you want to display personalized product recommendations based on the user’s preferences and browsing history. Here is where conditional statements come into play.
Conditional statements allow programmers to control the flow of execution by making decisions based on certain conditions. These statements evaluate whether a condition is true or false and execute specific blocks of code accordingly. For instance, in our online shopping simulation, if the user has previously searched for electronics, we can use a conditional statement to display recommended electronic products; otherwise, we will show general popular items.
To better understand the significance and utility of conditional statements, consider the following key points:
- They provide flexibility: By using conditional statements, developers can create programs that dynamically adapt to different situations.
- They enable decision-making: Conditional statements allow us to make choices within our code based on specific conditions or variables.
- They enhance interactivity: With conditional logic, applications can respond differently depending on user input or system states.
- They improve efficiency: By selectively executing only relevant parts of code, conditional statements optimize performance and reduce unnecessary computations.
Let’s further illustrate how these concepts work through a table showcasing common operators used in conditional expressions:
Operator | Description |
---|---|
== | Checks if two values are equal |
!= | Checks if two values are not equal |
> | Checks if one value is greater than another |
< | Checks if one value is less than another |
By utilizing such operators within conditional statements, programmers gain great control over their programs’ behavior.
Moving forward with our exploration into the mechanics of computer programming languages, our next section will focus on Control Structures and Code Execution. Understanding how control structures guide the flow of code execution is essential to mastering programming languages and creating efficient, reliable software systems.
Control Structures and Code Execution
Section H2: Control Structures and Code Execution
Transitioning from the previous section, where we explored conditional statements, we now delve into control structures and code execution. Imagine you are building a simple calculator application that requires user input for two numbers and an operator to perform a mathematical operation. The program needs to execute specific code based on the chosen operator (+, -, *, /). Understanding control structures is crucial in ensuring the correct flow of code execution.
Control structures allow programmers to dictate how their programs will behave by manipulating the order in which instructions are executed. They provide mechanisms for branching and looping within code, allowing for more complex decision-making processes. Let’s consider some key concepts:
- Branching: Control structures such as “if” statements enable different paths of execution based on certain conditions. For example, if a condition evaluates to true, one set of instructions can be executed, while another set can be executed if the condition is false.
- Looping: With control structures like “while” or “for” loops, repetitive tasks become more manageable. By specifying conditions under which the loop should repeat itself or defining a range of iterations, developers can automate actions without having to write duplicate lines of code.
- Exception handling: Control structures also play a vital role in managing errors and exceptions that may occur during runtime. Using constructs like “try-catch,” developers can anticipate potential issues and gracefully handle them instead of letting the program crash abruptly.
- Increased efficiency in coding through optimized control flow
- Enhanced readability due to well-defined structure
- Error prevention and improved debugging capabilities
- Empowerment to create sophisticated algorithms with complex logic
Additionally, incorporating a three-column table allows us to explore various types of control structures commonly found in programming languages:
Type | Description | Example |
---|---|---|
Conditional | Allows branching based on conditions. | if-else, switch |
Iterative | Enables repeating a block of code until a condition is met or for a specific number of iterations. | while, for |
Jump | Alters the flow of control by transferring execution to another part of the program. | break, continue |
Exceptional | Handles exceptional situations like errors and exceptions that may occur during program execution. | try-catch, throw |
In summary, control structures are essential components of programming languages that enable developers to manage the flow and execution of their code effectively. By utilizing branching, looping, exception handling, and jump statements, programmers can create efficient and robust applications. Understanding these concepts will ultimately enhance code readability and maintainability.
Note: It is important to remember that mastery over control structures requires practice and experimentation with different scenarios within your chosen programming language.